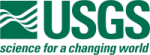 |
Isis 3 Programmer Reference
|
11 #include "ControlNetDiff.h"
17 #include "ControlMeasure.h"
18 #include "ControlNet.h"
19 #include "ControlNetVersioner.h"
20 #include "ControlPoint.h"
23 #include "PvlContainer.h"
25 #include "PvlKeyword.h"
26 #include "PvlObject.h"
69 if (diffFile.
hasGroup(
"Tolerances")) {
71 for (
int i = 0; i < tolerances.
keywords(); i++)
76 if (diffFile.
hasGroup(
"IgnoreKeys")) {
78 for (
int i = 0; i < ignoreKeys.
keywords(); i++)
96 diff(
"Filename", net1Name.
name(), net2Name.
name(), report);
116 for (
int p = 0; p < net1NumPts; p++) {
122 for (
int p = 0; p < net2NumPts; p++) {
130 for (
int i = 0; i < pointNames.size(); i++) {
132 if (idMap.size() == 2) {
133 compare(idMap[0], idMap[1], report);
135 else if (idMap.contains(0)) {
136 addUniquePoint(
"PointId", idMap[0].findKeyword(
"PointId")[0],
"N/A", report);
138 else if (idMap.contains(1)) {
139 addUniquePoint(
"PointId",
"N/A", idMap[1].findKeyword(
"PointId")[0], report);
164 int p1Measures = point1Pvl.
groups();
165 int p2Measures = point2Pvl.
groups();
171 for (
int m = 0; m < p1Measures; m++) {
173 measureMap[measure.
findKeyword(
"SerialNumber")[0]].insert(
177 for (
int m = 0; m < p2Measures; m++) {
179 measureMap[measure.
findKeyword(
"SerialNumber")[0]].insert(
184 for (
int i = 0; i < measureNames.size(); i++) {
186 if (idMap.size() == 2) {
189 else if (idMap.contains(0)) {
190 addUniqueMeasure(
"SerialNumber", idMap[0].findKeyword(
"SerialNumber")[0],
"N/A", pointReport);
192 else if (idMap.contains(1)) {
193 addUniqueMeasure(
"SerialNumber",
"N/A", idMap[1].findKeyword(
"SerialNumber")[0], pointReport);
225 for (
int k = 0; k < g1.
keywords(); k++)
226 keywordMap[g1[k].name()].insert(0, g1[k]);
227 for (
int k = 0; k < g2.
keywords(); k++)
228 keywordMap[g2[k].name()].insert(1, g2[k]);
231 for (
int i = 0; i < keywordNames.size(); i++) {
233 if (idMap.size() == 2) {
234 compare(idMap[0], idMap[1], groupReport);
236 else if (idMap.contains(0)) {
237 QString name = idMap[0].name();
239 diff(name, idMap[0][0],
"N/A", groupReport);
241 else if (idMap.contains(1)) {
242 QString name = idMap[1].name();
244 diff(name,
"N/A", idMap[1][0], groupReport);
267 QString name = k1.
name();
271 diff(name, k1[0], k2[0], report);
286 QString v1 = o1[name][0];
287 QString v2 = o2[name][0];
288 diff(name, v1, v2, report);
361 if (fabs(v1 - v2) > tol) {
QSet< QString > * m_ignoreKeys
The set of keywords to ignore by name.
QString name() const
Returns the keyword name.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
PvlGroup & group(const int index)
Return the group at the specified index.
Contains Pvl Groups and Pvl Objects.
A single keyword-value pair.
void addUniquePoint(QString label, QString v1, QString v2, PvlObject &parent)
Add a new keyword for the given point to the parent object.
QString name() const
Returns the name of the file excluding the path and the attributes in the file name.
void diff(QString name, PvlObject &o1, PvlObject &o2, PvlContainer &report)
Add a new difference keyword to the given report if the PvlObjects have different values for the keyw...
void addKeyword(const PvlKeyword &keyword, const InsertMode mode=Append)
Add a keyword to the container.
File name manipulation and expansion.
Pvl compare(FileName &net1Name, FileName &net2Name)
Compare two Control Networks given their file names, and return their differences.
void addValue(QString value, QString unit="")
Adds a value with units.
int groups() const
Returns the number of groups contained.
QMap< QString, double > * m_tolerances
The map of tolerances going from keyword name to tolerance value.
bool hasGroup(const QString &name) const
Returns a boolean value based on whether the object has the specified group or not.
void addTolerances(Pvl &diffFile)
Add the given ignore keys and tolerances to the persisent collections of such values.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
void addObject(const PvlObject &object)
Add a PvlObject.
PvlObject & object(const int index)
Return the object at the specified index.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
PvlKeyword makeKeyword(QString name, QString v1, QString v2)
Create a new keyword with the given label name and the given values.
Contains multiple PvlContainers.
ControlNetDiff()
Create a ControlNetDiff without any tolerances.
QString netId() const
Returns the ID for the network.
long long int BigInt
Big int.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
void compareGroups(PvlContainer &g1, PvlContainer &g2, PvlObject &report)
Compare two collections, or groupings, of PvlKeywords.
QString name() const
Returns the container name.
virtual ~ControlNetDiff()
Destroy the ControlNetDiff.
QString targetName() const
Returns the target for the network.
int numPoints() const
Returns the number of points that have been read in or are ready to write out.
void addGroup(const Isis::PvlGroup &group)
Add a group to the object.
double toDouble(const QString &string)
Global function to convert from a string to a double.
void addUniqueMeasure(QString label, QString v1, QString v2, PvlObject &parent)
Add a new keyword for the given measure to the parent object.
Pvl toPvl()
Generates a Pvl file from the currently stored control points and header.
This is free and unencumbered software released into the public domain.
int keywords() const
Returns the number of keywords contained in the PvlContainer.
PvlKeyword & findKeyword(const QString &kname, FindOptions opts)
Finds a keyword in the current PvlObject, or deeper inside other PvlObjects and Pvlgroups within this...
PvlKeyword & findKeyword(const QString &name)
Find a keyword with a specified name.
Contains more than one keyword-value pair.
void init()
Initialize the persistent structures used to maintain the state of this instance: its ignore keys and...
This is free and unencumbered software released into the public domain.
Handle various control network file format versions.