File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
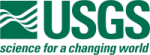 |
Isis 3 Programmer Reference
|
1 #ifndef ControlNetVitals_h
2 #define ControlNetVitals_h
12 #include <QStringList>
14 #include "ControlMeasure.h"
15 #include "ControlNet.h"
16 #include "ControlPoint.h"
95 void emitHistoryEntry(QString entry, QString
id, QVariant oldValue, QVariant newValue);
98 void networkChanged();
99 void historyEntry(QString, QString, QVariant, QVariant, QString);
void validateNetwork(ControlNet::ModType)
This SLOT is designed to intercept the networkModified() signal emitted by a Control Network whenever...
void measureModified(ControlMeasure *, ControlMeasure::ModType, QVariant, QVariant)
This SLOT is designed to intercept the measureModified() signal emitted by a Control Network whenever...
QList< QString > getImagesBelowMeasureThreshold(int num=3)
This method is designed to return a QList containing cube serials for all images that fall below a me...
ControlNetVitals(ControlNet *net)
Constructs a ControlNetVitals object from a ControlNet.
QString m_status
The string representing the status of the net. Healthy, Weak, or Broken.
bool hasIslands()
This method is designed to return true if islands exist in the ControlNet Graph and False otherwise.
void emitHistoryEntry(QString entry, QString id, QVariant oldValue, QVariant newValue)
This method is designed to be called whenever a modification is made to the network,...
QList< QList< QString > > m_islandList
A QList containing every island in the net. Each island consists of a QList containing All cube seria...
void deleteMeasure(ControlMeasure *)
This SLOT is designed to intercept the measureRemoved() signal emitted by a Control Network whenever ...
This is free and unencumbered software released into the public domain.
QList< ControlPoint * > getFreePoints()
This method is designed to return all free points in the Control Network.
QList< ControlPoint * > getAllPoints()
This method is designed to return all points in the Control Network.
int numFreePoints()
This method is designed to return the number of free points in the Control Network.
ModType
Control Point Modification Types.
int m_numPointsLocked
The number of edit locked points in the network.
QList< QString > getImagesBelowHullTolerance(int num=75)
This method is designed to return a QList containing cube serials for all images that fall below a co...
int numImagesBelowHullTolerance(int tolerance=75)
This method is designed to return the number of images that fall below a hull tolerance.
void removeMeasureFromCounts(ControlMeasure *measure)
Remove a measure from the internal counters.
const QList< QList< QString > > & getIslands()
This method is designed to return a QList containing each island present in the ControlNet.
QList< ControlPoint * > getIgnoredPoints()
This method is designed to return all ignored points in the Control Network.
QList< ControlPoint * > getPointsBelowMeasureThreshold(int num=3)
This method is designed to return all points that fall below a measure threshold.
int m_numMeasures
The number of measures in the network.
void validate()
This method is designed to evaluate the current vitals of the network to determine if any weaknesses ...
QString getStatusDetails()
This method is designed to return details for the status of the network.
int numPoints()
This method is designed to return the number of points in the Control Network.
QMap< ControlPoint::PointType, int > m_pointTypeCounts
The pointTypeCounts operates in the same fashion as the above two, except that the key would be the C...
ModType
Control Measure Modification Types.
void addPoint(ControlPoint *)
This SLOT is designed to intercept the newPoint() signal emitted from a ControlNetwork whenever a new...
int numIgnoredPoints()
This method is designed to return the number of ignored points in the Control Network.
int numImages()
This method is designed to return the number of images in the Control Network.
int numLockedPoints()
This method is designed to return the number of edit locked points in the Control Network.
QList< ControlPoint * > getFixedPoints()
This method is designed to return all fixed points in the Control Network.
int numPointsBelowMeasureThreshold(int num=3)
This method is designed to return the number of points that fall below a measure threshold.
QString m_statusDetails
The string providing details into the status of the network.
void addMeasureToCounts(ControlMeasure *measure)
Add a measure to the internal counters.
void deletePoint(ControlPoint *)
This SLOT is designed to intercept the removePoint() signal emitted by a Control Network whenever a p...
QList< ControlPoint * > getConstrainedPoints()
This method is designed to return all constrained points in the Control Network.
void initializeVitals()
This will initialize all necessary values and set up the point measure and image measure QMaps approp...
QList< QString > getCubeSerials()
This method is designed to return all cube serials present in the Control Network.
ControlPoint * getPoint(QString id)
This method is designed to return the Control Point with the associated point id from the Control Net...
QString getStatus()
This method is designed to return the current status of the network.
int m_numPoints
The number of points in the network.
ModType
Control Point Modification Types.
int numConstrainedPoints()
This method is designed to return the number of constrained points in the Control Network.
int numMeasures()
This method is designed to return the number of measures in the Control Network.
QMap< int, int > m_pointMeasureCounts
The measureCount maps track how many points/images have how many measures. For instance,...
QMap< int, int > m_imageMeasureCounts
The same is true for imageMeasureCounts, except for images.
void pointModified(ControlPoint *, ControlPoint::ModType, QVariant, QVariant)
This SLOT is designed to receive a signal emitted from the Control Network whenever a modification is...
ControlNet * m_controlNet
The Control Network that the vitals class is observing.
virtual ~ControlNetVitals()
De-constructor.
int m_numPointsIgnored
The number of ignored points in the network.
int numImagesBelowMeasureThreshold(int num=3)
This method is designed to return the number of images that fall below a measure threshold.
void addMeasure(ControlMeasure *)
This SLOT is designed to intercept the newMeasure() signal emitted by a Control Network whenever a me...
This is free and unencumbered software released into the public domain.
QList< ControlPoint * > getLockedPoints()
This method is designed to return all edit locked points in the Control Network.
int numFixedPoints()
This method is designed to return the number of fixed points in the Control Network.
int numIslands()
This method is designed to return the number of islands that exist in the ControlNet Graph.
QString getNetworkId()
This method is designed to return networkId of the observed Control Network.