File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
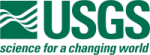 |
Isis 3 Programmer Reference
|
9 #include "ControlNetVitals.h"
16 #include "IException.h"
18 #include "ControlNet.h"
19 #include "ControlPoint.h"
20 #include "ControlMeasure.h"
111 emit historyEntry(entry,
id, oldValue, newValue, QDateTime::currentDateTime().
toString());
134 if (point->IsIgnored()) {
139 if (point->IsEditLocked()) {
177 QVariant oldValue, QVariant newValue) {
179 QString historyEntry;
182 case ControlPoint::EditLockModified:
184 historyEntry =
"Point Edit Lock Modified";
186 if (oldValue.toBool()) {
190 if (newValue.toBool()) {
195 oldValue, newValue );
199 case ControlPoint::IgnoredModified:
201 historyEntry =
"Point Ignored Modified";
203 if (oldValue.toBool()) {
205 if (point->IsEditLocked()) {
218 if (newValue.toBool()) {
220 if (point->IsEditLocked()) {
231 oldValue, newValue );
235 case ControlPoint::TypeModified:
237 historyEntry =
"Point Type Modified";
290 if (point->IsIgnored()) {
295 if (point->IsEditLocked()) {
355 QString historyEntry;
358 case ControlMeasure::IgnoredModified:
360 historyEntry =
"Measure Ignored Modified";
362 if ( !oldValue.toBool() && newValue.toBool() ) {
365 else if ( oldValue.toBool() && !newValue.toBool() ) {
489 QString historyEntry;
492 case ControlNet::Swapped:
496 case ControlNet::GraphModified:
622 if (i.key() >= num ) {
666 if (i.key() >= num ) {
718 if (point->IsIgnored()) ignoredPoints.append(point);
720 return ignoredPoints;
732 if (!point->IsIgnored() && point->IsEditLocked()) lockedPoints.append(point);
762 return constrainedPoints;
791 if (!point->IsIgnored() && point->GetNumMeasures() < num) belowThreshold.append(point);
793 return belowThreshold;
810 imagesBelowThreshold.append(serial);
813 return imagesBelowThreshold;
885 QString details =
"";
907 if (status.isEmpty()) {
909 details =
"This network is healthy.";
914 emit networkChanged();
void validateNetwork(ControlNet::ModType)
This SLOT is designed to intercept the networkModified() signal emitted by a Control Network whenever...
void measureModified(ControlMeasure *, ControlMeasure::ModType, QVariant, QVariant)
This SLOT is designed to intercept the measureModified() signal emitted by a Control Network whenever...
QList< QString > getImagesBelowMeasureThreshold(int num=3)
This method is designed to return a QList containing cube serials for all images that fall below a me...
ControlNetVitals(ControlNet *net)
Constructs a ControlNetVitals object from a ControlNet.
QString m_status
The string representing the status of the net. Healthy, Weak, or Broken.
static QString PointTypeToString(PointType type)
Obtain a string representation of a given PointType.
bool hasIslands()
This method is designed to return true if islands exist in the ControlNet Graph and False otherwise.
void emitHistoryEntry(QString entry, QString id, QVariant oldValue, QVariant newValue)
This method is designed to be called whenever a modification is made to the network,...
QList< QList< QString > > m_islandList
A QList containing every island in the net. Each island consists of a QList containing All cube seria...
void deleteMeasure(ControlMeasure *)
This SLOT is designed to intercept the measureRemoved() signal emitted by a Control Network whenever ...
This is free and unencumbered software released into the public domain.
QList< ControlPoint * > getFreePoints()
This method is designed to return all free points in the Control Network.
QList< ControlPoint * > getAllPoints()
This method is designed to return all points in the Control Network.
int numFreePoints()
This method is designed to return the number of free points in the Control Network.
QList< ControlMeasure * > GetValidMeasuresInCube(QString serialNumber)
Get all the valid measures pertaining to a given cube serial number.
ModType
Control Point Modification Types.
int m_numPointsLocked
The number of edit locked points in the network.
QList< QString > getImagesBelowHullTolerance(int num=75)
This method is designed to return a QList containing cube serials for all images that fall below a co...
int GetNumberOfValidMeasuresInImage(const QString &serialNumber)
Return the number of measures in image specified by serialNumber.
int numImagesBelowHullTolerance(int tolerance=75)
This method is designed to return the number of images that fall below a hull tolerance.
PointType
These are the valid 'types' of point.
void removeMeasureFromCounts(ControlMeasure *measure)
Remove a measure from the internal counters.
const QList< QList< QString > > & getIslands()
This method is designed to return a QList containing each island present in the ControlNet.
QString GetId() const
Return the Id of the control point.
QList< ControlPoint * > getIgnoredPoints()
This method is designed to return all ignored points in the Control Network.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
int GetNumValidMeasures() const
int GetNumMeasures() const
Returns the total number of measures for all control points in the network.
QList< QString > GetCubeSerials() const
Use this method to get a complete list of all the cube serial numbers in the network.
QList< ControlPoint * > getPointsBelowMeasureThreshold(int num=3)
This method is designed to return all points that fall below a measure threshold.
int m_numMeasures
The number of measures in the network.
void validate()
This method is designed to evaluate the current vitals of the network to determine if any weaknesses ...
QString getStatusDetails()
This method is designed to return details for the status of the network.
QString GetCubeSerialNumber() const
Return the serial number of the cube containing the coordinate.
@ Fixed
A Fixed point is a Control Point whose lat/lon is well established and should not be changed.
int numPoints()
This method is designed to return the number of points in the Control Network.
QMap< ControlPoint::PointType, int > m_pointTypeCounts
The pointTypeCounts operates in the same fashion as the above two, except that the key would be the C...
ModType
Control Measure Modification Types.
void addPoint(ControlPoint *)
This SLOT is designed to intercept the newPoint() signal emitted from a ControlNetwork whenever a new...
int numIgnoredPoints()
This method is designed to return the number of ignored points in the Control Network.
int numImages()
This method is designed to return the number of images in the Control Network.
int numLockedPoints()
This method is designed to return the number of edit locked points in the Control Network.
QList< ControlPoint * > GetPoints()
Return QList of all the ControlPoints in the network.
QList< ControlPoint * > getFixedPoints()
This method is designed to return all fixed points in the Control Network.
int numPointsBelowMeasureThreshold(int num=3)
This method is designed to return the number of points that fall below a measure threshold.
QString m_statusDetails
The string providing details into the status of the network.
void addMeasureToCounts(ControlMeasure *measure)
Add a measure to the internal counters.
void deletePoint(ControlPoint *)
This SLOT is designed to intercept the removePoint() signal emitted by a Control Network whenever a p...
@ Constrained
A Constrained point is a Control Point whose lat/lon/radius is somewhat established and should not be...
QList< ControlPoint * > getConstrainedPoints()
This method is designed to return all constrained points in the Control Network.
void initializeVitals()
This will initialize all necessary values and set up the point measure and image measure QMaps approp...
QList< QString > getCubeSerials()
This method is designed to return all cube serials present in the Control Network.
ControlPoint * getPoint(QString id)
This method is designed to return the Control Point with the associated point id from the Control Net...
QString getStatus()
This method is designed to return the current status of the network.
int m_numPoints
The number of points in the network.
ModType
Control Point Modification Types.
int numConstrainedPoints()
This method is designed to return the number of constrained points in the Control Network.
@ Free
A Free point is a Control Point that identifies common measurements between two or more cubes.
int numMeasures()
This method is designed to return the number of measures in the Control Network.
QMap< int, int > m_pointMeasureCounts
The measureCount maps track how many points/images have how many measures. For instance,...
This is free and unencumbered software released into the public domain.
PointType GetType() const
QMap< int, int > m_imageMeasureCounts
The same is true for imageMeasureCounts, except for images.
void pointModified(ControlPoint *, ControlPoint::ModType, QVariant, QVariant)
This SLOT is designed to receive a signal emitted from the Control Network whenever a modification is...
ControlNet * m_controlNet
The Control Network that the vitals class is observing.
virtual ~ControlNetVitals()
De-constructor.
int m_numPointsIgnored
The number of ignored points in the network.
int numImagesBelowMeasureThreshold(int num=3)
This method is designed to return the number of images that fall below a measure threshold.
void addMeasure(ControlMeasure *)
This SLOT is designed to intercept the newMeasure() signal emitted by a Control Network whenever a me...
This is free and unencumbered software released into the public domain.
QList< ControlPoint * > getLockedPoints()
This method is designed to return all edit locked points in the Control Network.
QList< QList< QString > > GetSerialConnections() const
This method searches through all the cube serial numbers in the network.
int numFixedPoints()
This method is designed to return the number of fixed points in the Control Network.
int numIslands()
This method is designed to return the number of islands that exist in the ControlNet Graph.
QString getNetworkId()
This method is designed to return networkId of the observed Control Network.