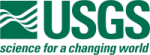 |
Isis 3 Programmer Reference
|
10 #include "ControlPoint.h"
12 #include <boost/numeric/ublas/symmetric.hpp>
13 #include <boost/numeric/ublas/io.hpp>
18 #include <QStringList>
20 #include "Application.h"
21 #include "CameraDetectorMap.h"
22 #include "CameraDistortionMap.h"
23 #include "CameraFocalPlaneMap.h"
24 #include "CameraGroundMap.h"
25 #include "ControlMeasure.h"
26 #include "ControlMeasureLogData.h"
27 #include "ControlNet.h"
31 #include "Longitude.h"
32 #include "PvlObject.h"
33 #include "SerialNumberList.h"
34 #include "SpecialPixel.h"
35 #include "Statistics.h"
37 using boost::numeric::ublas::symmetric_matrix;
38 using boost::numeric::ublas::upper;
48 ControlPoint::ControlPoint() : invalid(false) {
64 referenceMeasure = NULL;
78 referenceMeasure = NULL;
86 QListIterator<QString> i(*other.cubeSerials);
88 QString sn = i.next();
94 if (other.referenceMeasure == otherCm) {
127 referenceMeasure = NULL;
148 if (measures != NULL) {
150 for (
int i = 0; i < keys.size(); i++) {
151 delete(*measures)[keys[i]];
152 (*measures)[keys[i]] = NULL;
164 referenceMeasure = NULL;
237 QString msg =
"The SerialNumber is not unique. A measure with "
239 "exists for ControlPoint [" +
GetId() +
"]";
244 if (!measures->size()) {
246 referenceMeasure = measure;
248 else if (referenceMeasure->IsIgnored() && !measure->IsIgnored() &&
253 referenceMeasure = measure;
258 measures->insert(newSerial, measure);
259 cubeSerials->append(newSerial);
277 if (!measures->contains(serialNumber)) {
278 QString msg =
"No measure with serial number [" + serialNumber +
279 "] is owned by this point";
299 if (!IsIgnored() && !cm->IsIgnored()) {
305 return ControlMeasure::MeasureLocked;
309 measures->remove(serialNumber);
310 cubeSerials->removeAt(cubeSerials->indexOf(serialNumber));
313 if (cubeSerials->size()) {
314 if (referenceMeasure == cm) {
315 referenceMeasure = (*measures)[cubeSerials->at(0)];
320 referenceMeasure = NULL;
328 return ControlMeasure::Success;
367 if (index < 0 || index >= cubeSerials->size()) {
368 QString msg =
"index [" + QString(index) +
"] out of bounds";
372 return Delete(cubeSerials->at(index));
382 if (IsEditLocked()) {
406 return (*measures)[serialNumber];
418 return measures->value(serialNumber);
423 if (index < 0 || index >= cubeSerials->size()) {
424 QString msg =
"Index [" +
toString(index) +
"] out of range";
433 if (index < 0 || index >= cubeSerials->size()) {
434 QString msg =
"Index [" +
toString(index) +
"] out of range";
448 return !(referenceMeasure == NULL);
459 QString msg =
"Control point [" +
GetId() +
"] has no reference measure!";
463 return referenceMeasure;
472 QString msg =
"Control point [" +
GetId() +
"] has no reference measure!";
476 return referenceMeasure;
591 if (index < 0 || index >= cubeSerials->size()) {
592 QString msg =
"Index [";
593 msg +=
toString(index) +
"] out of range";
612 if (!cubeSerials->contains(sn)) {
613 QString msg =
"Point [" +
id +
"] has no measure with serial number [" +
643 referenceMeasure = measure;
662 if (oldStatus !=
ignore) {
711 QString msg =
"Invalid Point Enumeration, [" + QString(
type) +
"], for "
712 "Control Point [" +
GetId() +
"]";
737 RadiusSource::Source source) {
756 QString sourceFile) {
792 if (aprioriSP.GetLatSigma().
isValid())
794 if (aprioriSP.GetLonSigma().
isValid())
796 if (aprioriSP.GetLocalRadiusSigma().
isValid())
800 if (aprioriSP.GetXSigma().
isValid())
802 if (aprioriSP.GetYSigma().
isValid())
804 if (aprioriSP.GetZSigma().
isValid())
822 SurfacePointSource::Source source) {
840 QString sourceFile) {
905 QString msg =
"In method ControlPoint::ComputeApriori(). ControlPoint [" +
GetId() +
"] is ";
906 msg +=
"fixed or constrained and requires a priori coordinates";
914 int goodMeasures = 0;
921 for (
int i = 0; i < cubeSerials->size(); i++) {
923 if (m->IsIgnored()) {
927 Camera *cam = m->Camera();
930 QString msg =
"in method ControlPoint::ComputeApriori(). Camera has not been set in ";
931 msg +=
"measure for cube serial number [" + cubeSN +
"], Control Point id ";
932 msg +=
"[" +
GetId() +
"]. Camera must be set prior to calculating a priori coordinates";
936 bool setImageSuccess = cam->
SetImage(m->GetSample(), m->GetLine());
950 if (setImageSuccess) {
956 r2B += pB[0]*pB[0] + pB[1]*pB[1] + pB[2]*pB[2];
971 if (goodMeasures == 0) {
972 QString msg =
"in method ControlPoint::ComputeApriori(). ControlPoint [" +
GetId() +
"] has ";
973 msg +=
"no measures which project to the body";
980 double avgX = xB / goodMeasures;
981 double avgY = yB / goodMeasures;
982 double avgZ = zB / goodMeasures;
983 double avgR2 = r2B / goodMeasures;
984 double scale = sqrt(avgR2/(avgX*avgX+avgY*avgY+avgZ*avgZ));
1038 for (
int j = 0; j < keys.size(); j++) {
1040 if (m->IsIgnored()) {
1044 Camera *cam = m->Camera();
1073 double sample = m->GetSample();
1074 double computedY = m->GetFocalPlaneComputedY();
1075 double computedX = m->GetFocalPlaneComputedX();
1079 if (computedY < 0) {
1080 adjLine = m->GetLine() - 1.;
1083 adjLine = m->GetLine() + 1.;
1090 cam->
SetImage(sample, m->GetLine());
1100 if (computedY < 0) {
1101 deltaLine = -computedY/scalingY;
1104 deltaLine = computedY/scalingY;
1116 cuLine = m->GetLine() + deltaLine;
1120 cuSamp = m->GetFocalPlaneComputedX();
1121 cuLine = m->GetFocalPlaneComputedY();
1128 if (!fpmap->
SetFocalPlane(m->GetFocalPlaneComputedX(), m->GetFocalPlaneComputedY())) {
1129 QString msg =
"Sanity check #1 for ControlPoint [" +
GetId() +
1147 muSamp = m->GetSample();
1148 muLine = m->GetLine();
1153 if (!fpmap->
SetFocalPlane(m->GetFocalPlaneMeasuredX(), m->GetFocalPlaneMeasuredY())) {
1154 QString msg =
"Sanity check #2 for ControlPoint [" +
GetId() +
1166 double sampResidual = muSamp - cuSamp;
1167 double lineResidual = muLine - cuLine;
1202 for (
int j = 0; j < keys.size(); j++) {
1204 if (m->IsIgnored()) {
1208 Camera *cam = m->Camera();
1221 cam->
SetGround(GetAdjustedSurfacePoint());
1225 cam->
SetImage(m->GetSample(), m->GetLine());
1230 cam->
SetImage(m->GetSample(), m->GetLine());
1233 cam->
GroundMap()->
GetXY(GetAdjustedSurfacePoint(), &cudx, &cudy,
false);
1242 QString ControlPoint::GetChooserName()
const {
1262 QString ControlPoint::GetDateTime()
const {
1272 bool ControlPoint::IsEditLocked()
const {
1277 bool ControlPoint::IsRejected()
const {
1282 SurfacePoint ControlPoint::GetAdjustedSurfacePoint()
const {
1311 bool ControlPoint::IsIgnored()
const {
1316 bool ControlPoint::IsValid()
const {
1321 bool ControlPoint::IsInvalid()
const {
1336 switch (pointType) {
1341 str =
"Constrained";
1360 QString pointTypeString) {
1365 QString errMsg =
"There is no PointType that has a string representation"
1367 errMsg += pointTypeString;
1370 if (pointTypeString ==
"Fixed") {
1373 else if (pointTypeString ==
"Constrained") {
1376 else if (pointTypeString ==
"Free") {
1417 case RadiusSource::None:
1420 case RadiusSource::User:
1423 case RadiusSource::AverageOfMeasures:
1424 str =
"AverageOfMeasures";
1426 case RadiusSource::Ellipsoid:
1429 case RadiusSource::DEM:
1432 case RadiusSource::BundleSolution:
1433 str =
"BundleSolution";
1451 str = str.toLower();
1452 RadiusSource::Source source = RadiusSource::None;
1454 if (str ==
"user") {
1455 source = RadiusSource::User;
1457 else if (str ==
"averageofmeasures") {
1458 source = RadiusSource::AverageOfMeasures;
1460 else if (str ==
"ellipsoid") {
1461 source = RadiusSource::Ellipsoid;
1463 else if (str ==
"dem") {
1464 source = RadiusSource::DEM;
1466 else if (str ==
"bundlesolution") {
1467 source = RadiusSource::BundleSolution;
1493 SurfacePointSource::Source source) {
1498 case SurfacePointSource::None:
1501 case SurfacePointSource::User:
1504 case SurfacePointSource::AverageOfMeasures:
1505 str =
"AverageOfMeasures";
1507 case SurfacePointSource::Reference:
1510 case SurfacePointSource::Basemap:
1513 case SurfacePointSource::BundleSolution:
1514 str =
"BundleSolution";
1529 ControlPoint::SurfacePointSource::Source
1533 str = str.toLower();
1534 SurfacePointSource::Source source = SurfacePointSource::None;
1536 if (str ==
"user") {
1537 source = SurfacePointSource::User;
1539 else if (str ==
"averageofmeasures") {
1540 source = SurfacePointSource::AverageOfMeasures;
1542 else if (str ==
"reference") {
1543 source = SurfacePointSource::Reference;
1545 else if (str ==
"basemap") {
1546 source = SurfacePointSource::Basemap;
1548 else if (str ==
"bundlesolution") {
1549 source = SurfacePointSource::BundleSolution;
1566 SurfacePoint ControlPoint::GetAprioriSurfacePoint()
const {
1571 ControlPoint::RadiusSource::Source ControlPoint::GetAprioriRadiusSource()
1577 bool ControlPoint::HasAprioriCoordinates() {
1676 QString ControlPoint::GetAprioriRadiusSourceFile()
const {
1681 ControlPoint::SurfacePointSource::Source
1682 ControlPoint::GetAprioriSurfacePointSource()
const {
1697 QString ControlPoint::GetAprioriSurfacePointSourceFile()
const {
1702 int ControlPoint::GetNumMeasures()
const {
1703 return measures->size();
1714 for (
int cm = 0; cm < keys.size(); cm++) {
1715 if (!(*measures)[keys[cm]]->IsIgnored()) {
1731 for (
int cm = 0; cm < keys.size(); cm++) {
1732 if ((*measures)[keys[cm]]->IsEditLocked()) {
1747 return cubeSerials->contains(serialNumber);
1765 QString msg =
"There is no reference measure set in the ControlPoint [" +
1797 int index = cubeSerials->indexOf(sn);
1799 if (
throws && index == -1) {
1800 QString msg =
"ControlMeasure [" + sn +
"] does not exist in point [" +
1819 QString msg =
"There is no reference measure for point [" +
id +
"]."
1820 " This also means of course that the point is empty!";
1846 if (!cm->IsIgnored()) {
1847 stats.
AddData((cm->*statFunc)());
1858 if (!cm->IsIgnored()) {
1874 bool excludeIgnored)
const {
1876 for (
int i = 0; i < cubeSerials->size(); i++) {
1878 if (!excludeIgnored || !measure->IsIgnored()) {
1879 orderedMeasures.append(measures->value((*cubeSerials)[i]));
1882 return orderedMeasures;
1890 return *cubeSerials;
1952 return !(*
this == other);
1965 return other.GetNumMeasures() == GetNumMeasures() &&
1978 other.measures == measures &&
1984 other.cubeSerials == cubeSerials &&
1985 other.referenceMeasure == referenceMeasure;
2007 if (
this != &other) {
2010 for (
int i = cubeSerials->size() - 1; i >= 0; i--) {
2011 (*measures)[cubeSerials->at(i)]->SetEditLock(
false);
2012 Delete(cubeSerials->at(i));
2017 QHashIterator< QString, ControlMeasure * > i(*other.measures);
2018 while (i.hasNext()) {
2021 *newMeasure = *i.value();
2023 if (other.referenceMeasure == i.value()) {
2100 int nmeasures = measures->size();
2101 if ( nmeasures <= 0 ) {
2107 for(
int i = 0; i < nmeasures; i++) {
2113 if ( !m->IsIgnored() || m->IsRejected() ) {
2117 stats.
AddData(m->GetSampleResidual());
2131 int nmeasures = measures->size();
2132 if ( nmeasures <= 0 ) {
2138 for(
int i = 0; i < nmeasures; i++) {
2144 if ( !m->IsIgnored() || m->IsRejected() ) {
2148 stats.
AddData(m->GetLineResidual());
2162 int nmeasures = measures->size();
2163 if ( nmeasures <= 0 ) {
2169 for(
int i = 0; i < nmeasures; i++) {
2175 if ( m->IsIgnored() || m->IsRejected() ) {
2179 stats.
AddData(m->GetSampleResidual());
2180 stats.
AddData(m->GetLineResidual());
2192 int nmeasures = measures->size();
2193 if ( nmeasures <= 0 ) {
2197 for(
int i = 0; i < nmeasures; i++) {
bool editLock
This stores the edit lock state.
bool HasDateTime() const
Returns true if the datetime is not empty.
void ClearJigsawRejected()
Set jigsaw rejected flag for all measures to false and set the jigsaw rejected flag for the point its...
void SetRectangular(const Displacement &x, const Displacement &y, const Displacement &z, const Distance &xSigma=Distance(), const Distance &ySigma=Distance(), const Distance &zSigma=Distance())
Set surface point in rectangular body-fixed coordinates wtih optional sigmas.
QList< ControlMeasure * > getMeasures(bool excludeIgnored=false) const
This class is used to accumulate statistics on double arrays.
void AddData(const double *data, const unsigned int count)
Add an array of doubles to the accumulators and counters.
static QString PointTypeToString(PointType type)
Obtain a string representation of a given PointType.
Contains Pvl Groups and Pvl Objects.
void ZeroNumberOfRejectedMeasures()
Initialize the number of rejected measures to 0.
Status SetAprioriRadiusSourceFile(QString sourceFile)
This updates the filename of the DEM that the apriori radius came from.
bool IsCoord3Constrained()
Return bool indicating if 3rd coordinate is Constrained or not.
virtual bool GetXY(const SurfacePoint &spoint, double *cudx, double *cudy, bool test=true)
Compute undistorted focal plane coordinate from ground position using current Spice from SetImage cal...
const ControlMeasure * GetMeasure(QString serialNumber) const
Get a control measure based on its cube's serial number.
double GetNumericalValue() const
Get the value associated with this log data.
Status SetIgnored(bool newIgnoreStatus)
Set whether to ignore or use control point.
double GetSampleResidualRms() const
Get rms of sample residuals.
bool jigsawRejected
This stores the jigsaw rejected state.
Status SetChooserName(QString name)
Set the point's chooser name.
ControlPoint * parentPoint
Pointer to parent ControlPoint, may be null.
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
QString id
This is the control point ID.
void measureAdded(ControlMeasure *measure)
Updates the ControlNet graph for the measure's serial number to reflect the addition.
@ PointLocked
This is returned when the operation requires Edit Lock to be false but it is currently true.
Status SetEditLock(bool editLock)
Set the EditLock state.
SurfacePoint::CoordinateType GetCoordType()
Get the control point coordinate type (see the available types in SurfacePoint.h).
double GetResidualRms() const
Get rms of residuals.
double UndistortedFocalPlaneX() const
Gets the x-value in the undistorted focal plane coordinate system.
virtual double Sample() const
Returns the current sample number.
virtual CameraType GetCameraType() const =0
Returns the type of camera that was created.
QString GetReferenceSN() const
bool HasAprioriRadiusSourceFile() const
Checks to see if the radius source file has been set.
Status SetAdjustedSurfacePoint(SurfacePoint newSurfacePoint)
Set or update the surface point relating to this control point.
virtual bool SetUndistortedFocalPlane(double ux, double uy)
Compute distorted focal plane x/y.
void SetNumberOfRejectedMeasures(int numRejected)
Set (update) the number of rejected measures for the control point.
Status SetResidual(double sampResidual, double lineResidual)
Set the BundleAdjust Residual of the coordinate.
Statistics GetStatistic(double(ControlMeasure::*statFunc)() const) const
This function will call a given method on every control measure that this point has.
Status SetFocalPlaneMeasured(double x, double y)
Set the focal plane x/y for the measured line/sample.
@ Success
This is returned when the operation successfully took effect.
double FocalPlaneX() const
Gets the x-value in the focal plane coordinate system.
double DetectorLine() const
SurfacePoint aprioriSurfacePoint
The apriori surface point.
Status ComputeApriori()
Computes a priori lat/lon/radius point coordinates by determining the average lat/lon/radius of all m...
ControlPoint()
Construct a control point.
double FocalPlaneY() const
Gets the y-value in the focal plane coordinate system.
Status ComputeResiduals_Millimeters()
This method computes the residuals for a point.
PointType
These are the valid 'types' of point.
ControlNet * parentNetwork
List of Control Measures.
CameraDistortionMap * DistortionMap()
Returns a pointer to the CameraDistortionMap object.
bool HasAprioriSurfacePointSourceFile() const
Checks to see if the surface point source file has been set.
QString dateTime
This is the last modified date and time.
int GetNumberOfRejectedMeasures() const
Get the number of rejected measures on the control point.
QString GetId() const
Return the Id of the control point.
SurfacePoint GetBestSurfacePoint() const
Returns the adjusted surface point if it exists, otherwise returns the a priori surface point.
virtual bool SetGround(Latitude latitude, Longitude longitude)
Sets the lat/lon values to get the sample/line values.
SurfacePoint GetSurfacePoint() const
Returns the surface point (most efficient accessor).
Status SetAprioriSurfacePointSourceFile(QString sourceFile)
This updates the filename of where the apriori surface point came from.
double DetectorSample() const
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
int GetNumValidMeasures() const
QList< QString > getCubeSerialNumbers() const
Status SetRejected(bool rejected)
Set "jigsaw" rejected flag for a measure.
QString GetRadiusSourceString() const
Obtain a string representation of the RadiusSource.
Displacement is a signed length, usually in meters.
QString GetCubeSerialNumber() const
Return the serial number of the cube containing the coordinate.
bool referenceExplicitlySet
This indicates if a program has explicitely set the reference in this point or the implicit reference...
static QString RadiusSourceToString(RadiusSource::Source source)
Obtain a string representation of a given RadiusSource.
@ Fixed
A Fixed point is a Control Point whose lat/lon is well established and should not be changed.
const ControlPoint & operator=(const ControlPoint &pPoint)
bool IsCoord2Constrained()
Return bool indicating if 2nd coordinate is Constrained or not.
std::bitset< 3 > constraintStatus
This stores the constraint status of the a priori SurfacePoint.
This is free and unencumbered software released into the public domain.
@ Rectangular
Body-fixed rectangular x/y/z coordinates.
bool operator!=(const ControlPoint &pPoint) const
Compare two Control Points for inequality.
ModType
Control Measure Modification Types.
double GetLineResidualRms() const
Get rms of line residuals.
Status SetRefMeasure(ControlMeasure *cm)
Set the point's reference measure.
double Rms() const
Computes and returns the rms.
bool HasChooserName() const
Returns true if the choosername is not empty.
void emitMeasureModified(ControlMeasure *measure, ControlMeasure::ModType modType, QVariant oldValue, QVariant newValue)
This method is a wrapper to emit the measureModified() signal in the parent network is called wheneve...
bool invalid
If we forced a build that we would normally have thrown an exception for then this is set to true.
CoordinateType
Defines the coordinate typ, units, and coordinate index for some of the output methods.
Status SetAprioriSurfacePoint(SurfacePoint aprioriSP)
This updates the apriori surface point.
Status SetType(PointType newType)
Updates the control point's type.
virtual bool SetFocalPlane(const double dx, const double dy)
Compute detector position (sample,line) from focal plane coordinates.
void UpdatePointReference(ControlPoint *point, QString oldId)
Updates the key reference (poind Id) from the old one to what the point id was changet to.
QString GetSurfacePointSourceString() const
Obtain a string representation of the SurfacePointSource.
PointType type
What this control point is tying together.
int GetNumLockedMeasures() const
Returns the number of locked control measures.
const ControlMeasure * operator[](QString serialNumber) const
Same as GetMeasure (provided for convenience)
static QString SurfacePointSourceToString(SurfacePointSource::Source source)
Obtain a string representation of a given SurfacePointSource.
Status SetId(QString id)
Sets the Id of the control point.
@ Constrained
A Constrained point is a Control Point whose lat/lon/radius is somewhat established and should not be...
static SurfacePointSource::Source StringToSurfacePointSource(QString str)
Obtain a SurfacePoint::Source from a string.
static RadiusSource::Source StringToRadiusSource(QString str)
Obtain a RadiusSource::Source from a string.
void measureDeleted(ControlMeasure *measure)
Updates the node for this measure's serial number to reflect the deletion.
SurfacePoint adjustedSurfacePoint
This is the calculated, or aposterori, surface point.
void Load(PvlObject &p)
Loads the PvlObject into a ControlPoint.
static QString Name()
Returns the name of the application.
Status SetDateTime(QString newDateTime)
Set the point's last modified time.
bool IsFree() const
Return bool indicating if point is Free or not.
QString aprioriRadiusSourceFile
The name of the file that derives the apriori surface point's radius.
bool HasRefMeasure() const
Checks to see if a reference measure is set.
bool IsEditLocked() const
Return value for p_editLock or implicit lock on reference measure.
bool isValid() const
Test if this distance has been initialized or not.
bool ignore
True if we should preserve but ignore the entire control point and its measures.
int IndexOfRefMeasure() const
SurfacePointSource::Source aprioriSurfacePointSource
Where the apriori surface point originated from.
void pointUnIgnored(ControlPoint *point)
Update the ControlNet's internal structure when a ControlPoint is un-ignored.
void SetExplicitReference(ControlMeasure *measure)
Explicitly defines a new reference measure by pointer.
QString chooserName
This is the user name of the person who last modified this control point.
void emitMeasureModified(ControlMeasure *measure, ControlMeasure::ModType type, QVariant oldValue, QVariant newValue)
This method is a wrapper to emit the measureModified() signal and is called whenever a change is made...
int numberOfRejectedMeasures
This parameter is used and maintained by BundleAdjust for the jigsaw application.
@ Csm
Community Sensor Model Camera.
int IndexOf(ControlMeasure *, bool throws=true) const
int NumberOfConstrainedCoordinates()
Return bool indicating if point is Constrained or not.
void emitPointModified(ControlPoint *point, ControlPoint::ModType type, QVariant oldValue, QVariant newValue)
This method is a wrapper to emit the pointModified() signal and is called whenever a change is made t...
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool IsCoord1Constrained()
Return bool indicating if 1st coordinate is Constrained or not.
Namespace for the standard library.
const ControlMeasure * GetRefMeasure() const
Get the reference control measure.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
Convert between distorted focal plane and detector coordinates.
CameraGroundMap * GroundMap()
Returns a pointer to the CameraGroundMap object.
void emitNetworkStructureModified()
This method is a wrapper to emit the networkStructureModified() signal.
bool operator==(const ControlPoint &pPoint) const
Compare two Control Points for equality.
Status ResetApriori()
Reset all the Apriori info to defaults.
void pointIgnored(ControlPoint *point)
Update the ControlNet's internal structure when a ControlPoint is ignored.
@ Free
A Free point is a Control Point that identifies common measurements between two or more cubes.
bool isValid() const
Test if this displacement has been initialized or not.
int Delete(ControlMeasure *measure)
Remove a measurement from the control point, deleting reference measure is allowed.
QString aprioriSurfacePointSourceFile
FileName where the apriori surface point originated from.
PointType GetType() const
Status SetRejected(bool rejected)
Set the jigsawRejected state.
~ControlPoint()
This destroys the current instance and cleans up any and all allocated memory.
void Coordinate(double p[3]) const
Returns the x,y,z of the surface intersection in BodyFixed km.
@ Latitudinal
Planetocentric latitudinal (lat/lon/rad) coordinates.
Status SetAprioriSurfacePointSource(SurfacePointSource::Source source)
This updates the source of the surface point.
void ValidateMeasure(QString serialNumber) const
Throws an exception if none of the point's measures have the given serial number.
Status
This is a return status for many of the mutating (setter) method calls.
virtual double Line() const
Returns the current line number.
@ Failure
This is returned when an operation cannot be performed due to a problem such as the point is ignored ...
bool HasSerialNumber(QString serialNumber) const
Return true if given serial number exists in point.
static QString DateTime(time_t *curtime=0)
Returns the date and time as a QString.
bool IsConstrained()
Return bool indicating if point is Constrained or not.
Status SetFocalPlaneComputed(double x, double y)
Set the computed focal plane x/y for the apriori lat/lon.
void AddMeasure(ControlMeasure *measure)
Do the actual work of adding a measure to this point, without changing any extra data.
bool IsReferenceExplicit() const
double UndistortedFocalPlaneY() const
Gets the y-value in the undistorted focal plane coordinate system.
static PointType StringToPointType(QString pointTypeString)
Obtain a PointType given a string representation of it.
void PointModified()
What the heck is the point of this?
void Add(ControlMeasure *measure)
Add a measurement to the control point, taking ownership of the measure in the process.
@ Kilometers
The distance is being specified in kilometers.
RadiusSource::Source aprioriRadiusSource
Where the apriori surface point's radius originated from, most commonly used by jigsaw.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
bool IsFixed() const
Return bool indicating if point is Fixed or not.
QString GetPointTypeString() const
Obtain a string representation of the PointType.
Status SetAprioriRadiusSource(RadiusSource::Source source)
This updates the source of the radius of the apriori surface point.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
Status ComputeResiduals()
This method computes the BundleAdjust residuals for a point.