Loading [MathJax]/jax/output/NativeMML/config.js
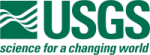 |
Isis 3 Programmer Reference
|
9 #include <QCoreApplication>
12 #include "IException.h"
14 #include "EmbreeTargetManager.h"
23 EmbreeTargetManager *EmbreeTargetManager::m_maker = 0;
30 EmbreeTargetManager::EmbreeTargetManager()
31 : m_maxCacheSize(10) {
43 for (
int i = 0; i < targetFiles.size(); i++) {
144 QString msg =
"Failed creating EmbreeTargetShape for [" + shapeFile
145 +
"] Too many EmbreeTargetShapes are already open.";
179 QString msg =
"Cannot free EmbreeTargetShape for file ["
180 + fullPath +
"] because it is not stored in the cache.";
188 int newCount = --(
m_targeCache[fullPath].m_referenceCount);
191 if ( newCount < 1 ) {
216 QString msg =
"Cannot free EmbreeTargetShape for file ["
217 + fullPath +
"] because it is not stored in the cache.";
static EmbreeTargetManager * m_maker
Initialize the singleton factory pointer.
QString fullFilePath(const QString &filePath) const
Helper function that takes a file path and returns the full file path.
int maxCacheSize() const
Return the maximum number of stored EmbreeTargetShapes.
Reference counting container for EmbreeTargetShapes.
EmbreeTargetShape * m_targetShape
!< The full path to the file used to construct the EmbreeTargetShape.
File name manipulation and expansion.
void removeTargetShape(const QString &shapeFile)
Method for removing an EmbreeTargetShape from the internal cache.
static void DieAtExit()
Exit termination routine.
bool inCache(const QString &shapeFile) const
Check if there is an already created EmbreeTargetShape for a file.
int currentCacheSize() const
Return the number of currently stored EmbreeTargetShapes.
int m_referenceCount
!< The EmbreeTargetShape.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
Class for managing the construction and destruction of EmbreeTargetShapes.
static EmbreeTargetManager * getInstance()
Retrieve reference to Singleton instance of this object.
EmbreeTargetManager()
This constructor will initialize the EmbreeTargetManager object to default values.
EmbreeTargetShape * create(const QString &shapeFile)
Get a pointer to an EmbreeTargetShape containing the information from a shape file.
~EmbreeTargetManager()
Destructor that frees all of the EmbreeTargetShapes managed by this object.
int m_maxCacheSize
!< The cache of created target shapes.
Embree Target Shape for planetary bodies.
void setMaxCacheSize(const int &numShapes)
Set the maximum number of stored EmbreeTargetShapes.
@ Programmer
This error is for when a programmer made an API call that was illegal.
QMap< QString, EmbreeTargetShapeContainer > m_targeCache
!< Pointer to the singleton factory.
Namespace for the standard library.
void free(const QString &shapeFile)
Notify the manager that an EmbreeTargetShape is no longer in use.
This is free and unencumbered software released into the public domain.