Loading [MathJax]/jax/output/NativeMML/config.js
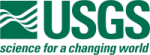 |
Isis 3 Programmer Reference
|
1 #ifndef EmbreeTargetManager_h
2 #define EmbreeTargetManager_h
12 #include "EmbreeTargetShape.h"
42 void free(
const QString &shapeFile);
46 bool inCache(
const QString &shapeFile)
const;
82 : m_fullFilePath(fullPath),
86 QString m_fullFilePath;
static EmbreeTargetManager * m_maker
Initialize the singleton factory pointer.
QString fullFilePath(const QString &filePath) const
Helper function that takes a file path and returns the full file path.
int maxCacheSize() const
Return the maximum number of stored EmbreeTargetShapes.
Reference counting container for EmbreeTargetShapes.
EmbreeTargetShape * m_targetShape
!< The full path to the file used to construct the EmbreeTargetShape.
void removeTargetShape(const QString &shapeFile)
Method for removing an EmbreeTargetShape from the internal cache.
EmbreeTargetShapeContainer(const QString &fullPath, EmbreeTargetShape *targetShape)
Constructs a container for a target shape and path.
static void DieAtExit()
Exit termination routine.
bool inCache(const QString &shapeFile) const
Check if there is an already created EmbreeTargetShape for a file.
int currentCacheSize() const
Return the number of currently stored EmbreeTargetShapes.
EmbreeTargetShapeContainer()
Default constructor.
int m_referenceCount
!< The EmbreeTargetShape.
Class for managing the construction and destruction of EmbreeTargetShapes.
static EmbreeTargetManager * getInstance()
Retrieve reference to Singleton instance of this object.
EmbreeTargetManager()
This constructor will initialize the EmbreeTargetManager object to default values.
EmbreeTargetShape * create(const QString &shapeFile)
Get a pointer to an EmbreeTargetShape containing the information from a shape file.
~EmbreeTargetManager()
Destructor that frees all of the EmbreeTargetShapes managed by this object.
int m_maxCacheSize
!< The cache of created target shapes.
Embree Target Shape for planetary bodies.
void setMaxCacheSize(const int &numShapes)
Set the maximum number of stored EmbreeTargetShapes.
QMap< QString, EmbreeTargetShapeContainer > m_targeCache
!< Pointer to the singleton factory.
void free(const QString &shapeFile)
Notify the manager that an EmbreeTargetShape is no longer in use.
This is free and unencumbered software released into the public domain.
This is free and unencumbered software released into the public domain.