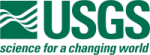 |
Isis 3 Programmer Reference
|
16 class UniversalGroundMap;
101 unsigned int &x,
unsigned int &y);
106 void SetGridBit(
unsigned int x,
unsigned int y,
bool latGrid);
107 bool GetGridBit(
unsigned int x,
unsigned int y,
bool latGrid);
109 unsigned int x2,
unsigned int y2,
bool m_extendGrid
If the grid should extend past the longitude domain boundary.
unsigned int p_width
This is the width of the grid.
This class is designed to encapsulate the concept of a Latitude.
Longitude * p_minLon
Lowest longitude in image.
PvlGroup * p_mapping
The mapping group representation of the projection or camera.
Latitude maxLatitude() const
Returns the maximum latitude for the grid.
Longitude * p_maxLon
Highest longitude in image.
UniversalGroundMap * p_groundMap
This calculates single grid pts.
Longitude maxLongitude() const
Returns the maximum longitude for the grid.
char * p_grid
This stores the bits of each pixel in the grid.
bool GetGridBit(unsigned int x, unsigned int y, bool latGrid)
Returns true if the specified coordinate is on the grid lines.
This class is designed to encapsulate the concept of a Longitude.
PvlGroup * GetMappingGroup()
Returns a mapping group representation of the projection or camera.
Latitude * p_maxLat
Highest latitude in image.
char * p_latLinesGrid
This stores the bits of each pixel in the grid.
Calculates a lat/lon grid over an area.
Contains multiple PvlContainers.
Latitude minLatitude() const
Returns the minimum latitude for the grid.
unsigned long p_gridSize
This is width*height.
double p_defaultResolution
Default step size in degrees/pixel.
bool p_reinitialize
True if we need to reset p_grid in CreateGrid.
Latitude * p_minLat
Lowest latitude in image.
Defines an angle and provides unit conversions.
void SetGroundLimits(Latitude minLat, Longitude minLon, Latitude maxLat, Longitude maxLon)
This restricts (or grows) the ground range in which to draw grid lines.
void WalkBoundary()
This draws grid lines along the extremes of the lat/lon box of the grid.
bool PixelOnGrid(int x, int y)
Returns true if the grid is on this point.
Program progress reporter.
virtual bool GetXY(Latitude lat, Longitude lon, unsigned int &x, unsigned int &y)
This method converts a lat/lon to an X/Y.
void SetGridBit(unsigned int x, unsigned int y, bool latGrid)
This flags a bit as on the grid lines.
void DrawLineOnGrid(unsigned int x1, unsigned int y1, unsigned int x2, unsigned int y2, bool isLatLine)
This sets the bits on the grid along the specified line.
UniversalGroundMap * GroundMap()
Returns the ground map for children.
unsigned int p_height
This is the height of the grid.
GroundGrid(UniversalGroundMap *gmap, bool splitLatLon, bool extendGrid, unsigned int width, unsigned int height)
This method initializes the class by allocating the grid, calculating the lat/lon range,...
Longitude minLongitude() const
Returns the minimum longitude for the grid.
void CreateGrid(Latitude baseLat, Longitude baseLon, Angle latInc, Angle lonInc, Progress *progress=0)
This method draws the grid internally, using default resolutions.
This is free and unencumbered software released into the public domain.
virtual ~GroundGrid()
Delete the object.
char * p_lonLinesGrid
This stores the bits of each pixel in the grid.