File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
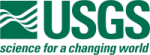 |
Isis 3 Programmer Reference
|
7 #include "ImageHistogram.h"
10 #include "ControlNet.h"
11 #include "ControlMeasure.h"
30 ImageHistogram::ImageHistogram(
double minimum,
double maximum,
int nbins) :
57 double startSample,
double startLine,
58 double endSample,
double endLine,
59 int bins,
bool addCubeData) {
60 InitializeFromCube(cube, statsBand, progress, bins, startSample, startLine,
64 Brick cubeDataBrick((
int)(endSample - startSample + 1),
68 int startBand = statsBand;
69 int endBand = statsBand;
76 if (progress != NULL) {
77 progress->
SetText(
"Gathering histogram");
79 (
int)(endLine - startLine + 1) * (
int)(endBand - startBand + 1));
83 for (
int band = startBand; band <= endBand; band++) {
84 for (
int line = (
int)startLine; line <= endLine; line++) {
86 cube.
read(cubeDataBrick);
88 if (progress != NULL) {
96 void ImageHistogram::InitializeFromCube(
Cube &cube,
int statsBand,
97 Progress *progress,
int nbins,
double startSample,
double startLine,
98 double endSample,
double endLine) {
100 if ( (statsBand < 0) || (statsBand > cube.
bandCount() ) ) {
101 string msg =
"Cannot gather histogram for band [" +
IString(statsBand) +
108 double minDnValue =
Null;
109 double maxDnValue =
Null;
120 else if (cube.
pixelType() == UnsignedWord) {
127 else if (cube.
pixelType() == SignedWord) {
138 else if (cube.
pixelType() == UnsignedInteger ||
146 IString msg =
"Unsupported pixel type";
150 if (startSample ==
Null)
153 if (endSample ==
Null)
156 if (startLine ==
Null)
163 if (minDnValue ==
Null || maxDnValue ==
Null) {
165 Brick cubeDataBrick((
int)(endSample - startSample + 1),
172 int startBand = statsBand;
173 int endBand = statsBand;
175 if (statsBand == 0) {
180 if (progress != NULL) {
182 progress->
SetText(
"Computing min/max for histogram");
184 (
int)(endLine - startLine + 1) * (
int)(endBand - startBand + 1) );
188 for (
int band = startBand; band <= endBand; band++) {
189 for (
int line = (
int)startLine; line <= endLine; line++) {
191 cubeDataBrick.SetBasePosition(qRound(startSample), line, band);
192 cube.
read(cubeDataBrick);
193 stats.AddData(cubeDataBrick.DoubleBuffer(), cubeDataBrick.size());
195 if (progress != NULL) {
201 if (stats.ValidPixels() == 0) {
206 minDnValue = stats.Minimum();
207 maxDnValue = stats.Maximum();
229 const unsigned int count) {
232 int nbins =
p_bins.size();
234 for (
unsigned int i = 0; i < count; i++) {
236 if (BinRangeStart() == BinRangeEnd() ) {
240 index = (int) floor((
double)(nbins - 1) / (BinRangeEnd() - BinRangeStart()) *
241 (data[i] - BinRangeStart() ) + 0.5);
243 if (index < 0) index = 0;
244 if (index >= nbins) index = nbins - 1;
259 int nbins =
p_bins.size();
262 if (BinRangeStart() == BinRangeEnd() ) {
266 index = (int) floor((
double)(nbins - 1) / (BinRangeEnd() - BinRangeStart() ) *
267 (data - BinRangeStart() ) + 0.5);
269 if (index < 0) index = 0;
270 if (index >= nbins) index = nbins - 1;
285 const unsigned int count) {
288 int nbins =
p_bins.size();
290 for (
unsigned int i = 0; i < count; i++) {
293 if (BinRangeStart() == BinRangeEnd() ) {
297 index = (int) floor((
double)(nbins - 1) / (BinRangeEnd() - BinRangeStart()) *
298 (data[i] - BinRangeStart()) + 0.5);
300 if (index < 0) index = 0;
301 if (index >= nbins) index = nbins - 1;
319 double &low,
double &high)
const {
320 if ( (index < 0) || (index >= (
int)
p_bins.size() ) ) {
326 double binSize = (BinRangeEnd() - BinRangeStart()) / (
double)(
p_bins.size() - 1);
327 low = BinRangeStart() - binSize / 2.0 + binSize * (double) index;
328 high = low + binSize;
void SetBasePosition(const int start_sample, const int start_line, const int start_band)
This method is used to set the base position of the shape buffer.
double base() const
Returns the base value for converting 8-bit/16-bit pixels to 32-bit.
void AddData(const double *data, const unsigned int count)
Add an array of doubles to the accumulators and counters.
virtual void AddData(const double *data, const unsigned int count)
Add an array of doubles to the histogram counters.
void CheckStatus()
Checks and updates the status.
void read(Blob &blob, const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
This method will read data from the specified Blob object.
void SetBins(const int bins)
Change the number of bins in the histogram and reset counters.
void SetMaximumSteps(const int steps)
This sets the maximum number of steps in the process.
double * DoubleBuffer() const
Returns the value of the shape buffer.
Buffer for containing a three dimensional section of an image.
ImageHistogram(double minimum, double maximum, int bins=1024)
Constructs a histogram object.
void RemoveData(const double *data, const unsigned int count)
Remove an array of doubles from the accumulators and counters.
virtual void RemoveData(const double *data, const unsigned int count)
Remove an array of doubles from the histogram counters.
double multiplier() const
Returns the multiplier value for converting 8-bit/16-bit pixels to 32-bit.
void SetValidRange(const double minimum=Isis::ValidMinimum, const double maximum=Isis::ValidMaximum)
Changes the range of the bins.
void SetText(const QString &text)
Changes the value of the text string reported just before 0% processed.
std::vector< BigInt > p_bins
The array of counts.
IO Handler for Isis Cubes.
virtual int bandCount() const
Returns the number of virtual bands for the cube.
~ImageHistogram()
Destructs a histogram object.
Program progress reporter.
const double Null
Value for an Isis Null pixel.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
PixelType pixelType() const
Container of a cube histogram.
bool IsValidPixel(const double d)
Returns if the input pixel is valid.
Statistics(QObject *parent=0)
Constructs an IsisStats object with accumulators and counters set to zero.
int size() const
Returns the total number of pixels in the shape buffer.
Adds specific functionality to C++ strings.
QString ArraySubscriptNotInRange(int index)
This error should be used when an Isis object or application is checking array bounds and the legal r...
virtual void BinRange(const int index, double &low, double &high) const
Returns the left edge and right edge values of a bin.
This is free and unencumbered software released into the public domain.