File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
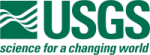 |
Isis 3 Programmer Reference
|
1 #ifndef InterestOperator_h
2 #define InterestOperator_h
16 #include "UniversalGroundMap.h"
17 #include "ControlNetValidMeasure.h"
18 #include "ImageOverlapSet.h"
20 #include "geos/geom/Point.h"
21 #include "geos/geom/Coordinate.h"
22 #include "geos/geom/MultiPolygon.h"
23 #include "geos/util/GEOSException.h"
117 void SetPatternValidPercent(
const double percent);
118 void SetPatternSampling(
const double percent);
119 void SetSearchSampling(
const double percent);
120 void SetTolerance(
double tolerance);
121 void SetPatternReduction(std::vector<int> samples, std::vector<int> lines);
132 void Operate(
ControlNet &pNewNet, QString psSerialNumFile, QString psOverlapListFile =
"");
136 return p_interestAmount;
141 return p_worstInterest;
195 double p_worstInterest, p_interestAmount;
Isis::PvlGroup Operator()
Return the Operator name.
ControlNetValidMeasure class.
double mdOrigLine
Control Measure's original line.
double p_cubeLine
Point in a cube from a chip perspective.
InterestOperator(Pvl &pPvl)
Create InterestOperator object.
void ProcessLocked_Point_Reference(ControlPoint &pCPoint, PvlObject &pPvlObj, int &piMeasuresModified)
Process (Validate and Log) Point with Lock or with Referemce Measure Locked.
Contains Pvl Groups and Pvl Objects.
virtual double Interest(Chip &subCube)=0
Calculate the interest.
Isis::ImageOverlapSet mOverlaps
Holds the overlaps from the Overlaplist.
void SetClipPolygon(const geos::geom::MultiPolygon &clipPolygon)
Set the Clip Polygon for points to be contained in the overlaps.
double mdDn
Cube DN value at most interesting sample,line.
virtual int Padding()
Sets an offset to pass in larger chips if operator requires it This is used to offset the subchip siz...
double p_minimumInterest
Specified in the Pvl Operator group.
double CubeLine() const
Return the search chip cube line that best matched.
double mdIncidence
Incidence angle at most interesting sample,line.
double mdInterest
Resulting interest amt from InterestOperator.
virtual bool CompareInterests(double int1, double int2)
Compare for int1 greater than / equal to int2.
void FindCnetRef(ControlNet &pNewNet)
Find best ref for an entire control net by calculating the interest and moving point to a better inte...
const geos::geom::MultiPolygon * FindOverlap(Isis::ControlPoint &pCnetPoint)
Find if a point is in the overlap.
Container for cube-like labels.
int miDeltaLine
The number of Lines the point has been moved.
InterestResults * mtInterestResults
Holds the results of an interest computation.
double CubeSample() const
Return the search chip cube sample that best matched.
double InterestAmount() const
Return the Interest Amount.
geos::geom::MultiPolygon * p_clipPolygon
Clipping polygon set by SetClipPolygon (line,samp)
int p_deltaSamp
Specified in the Pvl Operator group for the box car size.
void InitInterestResults(int piIndex)
Init Interest Results structure.
virtual ~InterestOperator()
Destroy InterestOperator object.
bool mbOverlaps
If Overlaplist exists.
void InitInterestOptions()
Initialise Interest Options to defaults.
bool mbValid
Value of the interest operator result (success)
double mdBestLine
Most interesting line.
Contains multiple PvlContainers.
int InterestByPoint(ControlPoint &pCnetPoint)
Calculate interest for a Control Point.
This class is used to find the overlaps between all the images in a list of serial numbers.
Structure to hold Interest Results.
double mdOrigSample
Control Measure's original sample.
IO Handler for Isis Cubes.
int miDeltaSample
The number of Samples the point has been moved.
Isis::PvlGroup mOperatorGrp
Operator group that created this projection.
double WorstInterest() const
Return the Worst(least value) Interest.
QString operatorName() const
Return name of the matching operator.
QString msSerialNum
Serial Number of the Measure.
A small chip of data used for pattern matching.
double mdEmission
Emission angle at most interesting sample,line.
double mdResolution
Camera resolution at most interesting sample,line.
double mdBestSample
Most interesting sample.
This is free and unencumbered software released into the public domain.
bool Operate(Cube &pCube, UniversalGroundMap &pUnivGrndMap, int piSample, int piLine)
Operate used by the app interestcube- to calculate interest by sample,line.
void Parse(Pvl &pPvl)
Parse the Interest specific keywords.
const geos::geom::MultiPolygon * FindOverlapByImageFootPrint(Isis::ControlPoint &pCnetPoint)
Find imageoverlaps by finding the intersection of image footprints.
bool InterestByMeasure(int piMeasure, Isis::ControlMeasure &pCnetMeasure, Isis::Cube &pCube)
Calculate interest for a measure by index.