File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
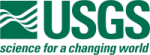 |
Isis 3 Programmer Reference
|
13 #include "Interpolator.h"
15 #include "SpecialPixel.h"
19 #include <geos/geom/MultiPolygon.h>
90 Chip(
const int samples,
const int lines);
93 void SetSize(
const int samples,
const int lines);
126 void SetValue(
int sample,
int line,
const double &value) {
127 m_buf[line-1][sample-1] = value;
146 return m_buf[line-1][sample-1];
163 inline double GetValue(
int sample,
int line)
const {
164 return m_buf[line-1][sample-1];
167 void TackCube(
const double cubeSample,
const double cubeLine);
191 void Load(
Cube &cube,
const double rotation = 0.0,
const double scale = 1.0,
194 const double scale = 1.0,
const int band = 1);
195 void Load(
Cube &cube,
const Affine &affine,
const bool &keepPoly =
true,
241 double value =
GetValue(sample, line);
255 bool IsValid(
double percentage);
256 Chip Extract(
int samples,
int lines,
int samp,
int line);
260 void Write(
const QString &filename);
324 if (type == Interpolator::NearestNeighborType ||
325 type == Interpolator::BiLinearType ||
326 type == Interpolator::CubicConvolutionType) {
331 QString msg =
"Invalid Interpolator type. Cannot use [";
332 msg +=
toString(type) +
"] to read cube into chip.";
337 void Init(
const int samples,
const int lines);
338 void Read(
Cube &cube,
const int band);
339 std::vector<int>
MovePoints(
int startSamp,
int startLine,
340 int endSamp,
int endLine);
342 double x1,
double y1,
343 double x2,
double y2,
348 std::vector< std::vector<double> >
m_buf;
const double ValidMaximum
The maximum valid double value for Isis pixels.
Chip & operator=(const Chip &other)
Copy assignment operator.
bool IsInsideChip(double sample, double line)
void SetValidRange(const double minimum=Isis::ValidMinimum, const double maximum=Isis::ValidMaximum)
Set the valid range of data in the chip.
std::vector< int > MovePoints(int startSamp, int startLine, int endSamp, int endLine)
This method is called by Load() to move a control point across the chip.
This class is used to accumulate statistics on double arrays.
int m_tackSample
Middle sample of the chip.
void SetSize(const int samples, const int lines)
Change the size of the Chip.
void TackCube(const double cubeSample, const double cubeLine)
This sets which cube position will be located at the chip tack position.
double GetValue(int sample, int line)
Loads a Chip with a value.
void SetReadInterpolator(const Interpolator::interpType type)
Sets Interpolator Type for loading a chip.
double ChipSample() const
void SetCubePosition(const double sample, const double line)
Compute the position of the chip given a cube coordinate.
double CubeSample() const
bool PointsColinear(double x0, double y0, double x1, double y1, double x2, double y2, double tol)
This method is called by Load() to determine whether the given 3 points are nearly colinear.
Chip Extract(int samples, int lines, int samp, int line)
Extract a sub-chip from a chip.
double m_validMaximum
valid maximum chip pixel value
void SetValue(int sample, int line, const double &value)
Sets a value in the chip.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
geos::geom::MultiPolygon * m_clipPolygon
clipping polygon set by SetClipPolygon
void Load(Cube &cube, const double rotation=0.0, const double scale=1.0, const int band=1)
Load cube data into the Chip.
void SetTransform(const Affine &affine, const bool &keepPoly=true)
Sets the internal Affine transform to new translation.
double m_chipLine
chip line set by SetChip/CubePosition
int TackSample() const
This method returns a chip's fixed tack sample; the middle of the chip.
void SetAllValues(const double &d)
Single value assignment operator.
Interpolator::interpType m_readInterpolator
Interpolator type set by.
int TackLine() const
This method returns a chip's fixed tack line; the middle of the chip.
double m_cubeTackSample
cube sample at the chip tack
interpType
The interpolator type, including: None, Nearest Neighbor, BiLinear or Cubic Convultion.
void Write(const QString &filename)
Writes the contents of the Chip to a cube.
double m_validMinimum
valid minimum chip pixel value
int m_chipLines
Number of lines in the chip.
double m_cubeTackLine
cube line at the chip tack
void Init(const int samples, const int lines)
Common initialization used by constructors.
IO Handler for Isis Cubes.
const Affine & GetTransform() const
Returns the Affine transformation of chip-to-cube indices.
virtual ~Chip()
Destroys the Chip object.
int m_tackLine
Middle line of the chip.
const double ValidMinimum
The minimum valid double value for Isis pixels.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool IsValid(int sample, int line)
double GetValue(int sample, int line) const
Get a value from a Chip.
double m_chipSample
chip sample set by SetChip/CubePosition
A small chip of data used for pattern matching.
int m_chipSamples
Number of samples in the chip.
double m_cubeSample
cube sample set by SetCubePosition
void Read(Cube &cube, const int band)
This method reads data from a cube and puts it into the chip.
Isis::Statistics * Statistics()
Returns a statistics object of the current data in the chip.
Interpolator::interpType GetReadInterpolator()
Access method that returns the Interpolator Type used for loading a chip.
void SetChipPosition(const double sample, const double line)
Compute the position of the cube given a chip coordinate.
std::vector< std::vector< double > > m_buf
Chip buffer.
void SetClipPolygon(const geos::geom::MultiPolygon &clipPolygon)
Sets the clipping polygon for this chip.
QString m_filename
FileName of loaded cube.
Affine m_affine
Transform set by SetTransform.
double m_cubeLine
cube line set by SetCubePosition
This is free and unencumbered software released into the public domain.