Loading [MathJax]/jax/output/NativeMML/config.js
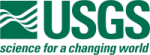 |
Isis 3 Programmer Reference
|
1 #include "MatrixOptions.h"
7 #include "CorrelationMatrix.h"
8 #include "MatrixSceneWidget.h"
9 #include "MatrixOptionsDialog.h"
19 m_goodColor = Qt::cyan;
20 m_badColor = Qt::magenta;
21 m_colorTolerance = 0.2;
24 m_focusOption = MatrixOptions::Tolerance;
27 m_image1 =
"Specific Img1";
28 m_parameter1 =
"Specific Param1";
29 m_image2 =
"Specific Img2";
30 m_parameter2 =
"Specific Param2";
34 m_focusTolSelectedElement = 1.0;
38 for (
int i = 0; i < 10; i++) {
39 m_goodElements.append(i);
40 m_badElements.append(i + 10);
45 m_currentImg1 =
"Current Image 1";
46 m_currentParam1 =
"Current Parameter 1";
47 m_currentImg2 =
"Current Image 2";
48 m_currentParam2 =
"Current Parameter 2";
51 optionsDialog->setAttribute(Qt::WA_DeleteOnClose);
52 optionsDialog->show();
54 connect(optionsDialog, SIGNAL( optionsUpdated() ),
55 this, SIGNAL( optionsUpdated() ) );
105 return m_colorTolerance;
127 m_tolerance = tolerance;
137 m_colorTolerance = tolerance;
167 return m_focusOption;
179 return m_focusTolSelectedElement;
224 return m_badElements;
233 return m_goodElements;
242 m_focusOption = option;
251 m_focusTolSelectedElement = value;
282 return m_currentValue;
291 return m_currentImg1;
300 return m_currentParam1;
309 return m_currentImg2;
318 return m_currentParam2;
327 m_currentValue = value;
336 m_currentImg1 = current;
345 m_currentParam1= current;
354 m_currentImg2 = current;
363 m_currentParam2 = current;
394 return m_parentMatrix;
void setFocusValue(double value)
void setBadElements(QList< double > badElements)
void setCurrentImage1(QString current)
bool colorScheme()
Use the green-red gradient if false.
FocusOption focusOption()
QList< double > badElements()
void setCurrentParameter1(QString current)
void setGoodElements(QList< double > goodElements)
QColor goodCorrelationColor()
The color selected for the correlation values that are below the given threshold.
double colorTolerance()
Threshold for what is considered a bad correlation.
QString currentParameter1()
QString focusParameter1()
void setCurrentImage2(QString current)
void setCurrentParameter2(QString current)
MatrixOptions(CorrelationMatrix parent, MatrixSceneWidget *scene)
Default Constructor.
This is a container for the correlation matrix that comes from a bundle adjust.
void setBadCorrelationColor(QColor color)
double currentCorrelation()
void setCurrentCorrelation(double value)
void setColorTolerance(double tolerance)
void setColorScheme(bool tolerance)
QString currentParameter2()
double focusValue()
The value of the spot on the matrix that we need to focus on.
This widget allows the user to modify the matrix display.
~MatrixOptions()
Constructor that sets up all the variables.
QString focusParameter2()
void setGoodCorrelationColor(QColor color)
QList< double > goodElements()
QMap< QString, QStringList > matrixImgsAndParams()
This slot will be called when a matrix element is clicked on.
QColor badCorrelationColor()
The color selected for the correlation values that are above the given threshold.
QMap< QString, QStringList > * imagesAndParameters()
Public access for the qmap of images and parameters.
This is free and unencumbered software released into the public domain.
void setFocusOption(FocusOption option)