File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
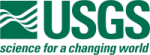 |
Isis 3 Programmer Reference
|
Go to the documentation of this file.
24 #include <QFileDialog>
25 #include <QInputDialog>
26 #include <QMessageBox>
28 #include "CorrelationMatrix.h"
29 #include "Directory.h"
30 #include "IException.h"
31 #include "MatrixSceneWidget.h"
44 QAction::setText( tr(
"View Correlation &Matrix...") );
55 qDebug() <<
"matrix good?";
101 if ( existingViews.count() ) {
102 for (
int i = 0; i < existingViews.count(); i++) {
103 viewOptions.append( existingViews[i]->windowTitle() );
107 viewOptions.append( tr(
"New Matrix View") );
109 if (viewOptions.count() > 1) {
110 QString selected = QInputDialog::getItem(NULL, tr(
"View to see matrix in"),
111 tr(
"Which view would you like your\nmatrix to be put into?"),
112 viewOptions, viewOptions.count() - 1,
false, &success);
114 viewToUse = viewOptions.indexOf(selected);
117 viewToUse = viewOptions.count() - 1;
120 bool newView =
false;
121 if (viewToUse == viewOptions.count() - 1) {
124 QUndoCommand::setText( tr(
"View matrix in new matrix view") );
127 else if (viewToUse != -1) {
140 internalData.append(newView?
"new view" :
"existing view");
177 if (matrixViewToUse == NULL) {
178 QString msg =
"The Correlation Matrix for this bundle could not be displayed";
185 QMessageBox::critical(NULL, tr(
"Error"), tr(e.
what()));
virtual bool setupExecution()
This sets up the state for the work order.
bool dependsOn(WorkOrder *other) const
This method returns true if other depends on a MatrixViewWorkOrder.
This is free and unencumbered software released into the public domain.
Provide Undo/redo abilities, serialization, and history for an operation.
bool m_isSavedToHistory
Set the work order to be shown in the HistoryTreeWidget.
void setInternalData(QStringList data)
Sets the internal data for this WorkOrder.
virtual bool isExecutable(CorrelationMatrix matrix)
This check is used by Directory::supportedActions(DataType data).
void computeCorrelationMatrix()
Read covariance matrix and compute correlation values This method reads the covariance matrix in from...
~MatrixViewWorkOrder()
Destructor.
This is a container for the correlation matrix that comes from a bundle adjust.
const char * what() const
Returns a string representation of this exception in its current state.
The main project for ipce.
MatrixViewWorkOrder(Project *project)
This method sets the text of the work order.
Directory * directory() const
Returns the directory associated with this Project.
bool setupExecution()
If WorkOrder::execute() returns true, a new matrix view is created.
This work order will open a MatrixSceneWidget and display the correlation matrix.
Project * project() const
Returns the Project this WorkOrder is attached to.
QList< MatrixSceneWidget * > matrixViews()
Accessor for the list of MatrixSceneWidgets currently available.
void undoExecution()
This method deletes the last matrix viewed.
void execute()
This method computes and displays the correlation matrix.
@ Programmer
This error is for when a programmer made an API call that was illegal.
@ WorkOrderFinished
This is used for work orders that will not undo or redo (See createsCleanState())
QStringList internalData() const
Gets the internal data for this WorkOrder.
CorrelationMatrix correlationMatrix()
Returns the CorrleationMatrix for this WorkOrder.
bool isValid()
This is the public accessor for the list of elements that should be displayed in the current view.
virtual MatrixViewWorkOrder * clone() const
This method clones the MatrixViewWorkOrder.
void setClean(bool value)
Function to change the clean state of the project.
This is free and unencumbered software released into the public domain.
MatrixSceneWidget * addMatrixView()
Add the matrix view widget to the window.