Loading [MathJax]/jax/output/NativeMML/config.js
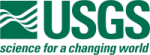 |
Isis 3 Programmer Reference
|
11 #include "WorkOrder.h"
14 #include <QFutureWatcher>
16 #include <QMutexLocker>
17 #include <QtConcurrentRun>
19 #include <QXmlStreamWriter>
21 #include "ControlList.h"
22 #include "IException.h"
23 #include "ImageList.h"
25 #include "ProgressBar.h"
27 #include "ProjectItem.h"
28 #include "ShapeList.h"
30 #include "XmlStackedHandlerReader.h"
44 m_context = NoContext;
61 m_status = WorkOrderNotStarted;
62 m_queuedAction = NoQueuedAction;
77 tr(
"Work orders cannot be created without a project."), _FILEINFO_);
80 connect(
this, SIGNAL(triggered()),
94 QAction(other.icon(), ((
QAction &)other).text(), other.parentWidget()),
97 QAction::setWhatsThis(other.whatsThis());
98 QAction::setToolTip(other.toolTip());
103 m_context = other.m_context;
105 m_imageList =
new ImageList(*other.m_imageList);
107 m_shapeList =
new ShapeList(*other.m_shapeList);
108 m_correlationMatrix = other.m_correlationMatrix;
109 m_controlList = other.m_controlList;
125 m_status = other.m_status;
126 m_queuedAction = other.m_queuedAction;
139 tr(
"Can not copy work order [%1] because it is currently running")
144 connect(
this, SIGNAL(triggered()),
298 m_controlList = controls;
394 else if ( item->isTemplate() ) {
509 else if ( item->isTemplate() ) {
547 tr(
"Can not store an unstable work order. The work order [%1] is currently "
552 stream.writeStartElement(
"workOrder");
554 stream.writeAttribute(
"actionText", QAction::text());
555 stream.writeAttribute(
"undoText", QUndoCommand::text());
557 stream.writeAttribute(
"type", metaObject()->className());
558 stream.writeAttribute(
"status",
toString(m_status));
561 stream.writeStartElement(
"images");
564 stream.writeStartElement(
"image");
565 stream.writeAttribute(
"id", imageId);
566 stream.writeEndElement();
569 stream.writeEndElement();
573 stream.writeStartElement(
"shapes");
576 stream.writeStartElement(
"shape");
577 stream.writeAttribute(
"id", shapeId);
578 stream.writeEndElement();
581 stream.writeEndElement();
585 stream.writeStartElement(
"internalDataValues");
588 stream.writeStartElement(
"dataValue");
589 stream.writeAttribute(
"value", str);
590 stream.writeEndElement();
593 stream.writeEndElement();
596 if (m_context != NoContext) {
597 stream.writeStartElement(
"context");
599 QString contextStr =
"ProjectContext";
600 stream.writeAttribute(
"value", contextStr);
602 stream.writeEndElement();
605 stream.writeEndElement();
632 QMutexLocker locker(
project()->workOrderMutex());
634 bool anyImagesAreNull =
false;
640 m_imageList->append(image);
643 anyImagesAreNull =
true;
647 if (anyImagesAreNull) {
664 QMutexLocker locker(
project()->workOrderMutex());
666 bool anyShapesAreNull =
false;
672 m_shapeList->append(shape);
675 anyShapesAreNull =
true;
679 if (anyShapesAreNull) {
697 QMutexLocker locker(
project()->workOrderMutex());
698 return m_correlationMatrix;
707 QMutexLocker locker(
project()->workOrderMutex());
708 return m_controlList;
737 QMutexLocker locker(
project()->workOrderMutex());
747 QMutexLocker locker(
project()->workOrderMutex());
757 QMutexLocker locker(
project()->workOrderMutex());
767 QMutexLocker locker(
project()->workOrderMutex());
796 QString result = QUndoCommand::text().remove(
"&").remove(
"...");
799 if (result.isEmpty()) {
801 result = QString(metaObject()->className()).remove(
"Isis::").remove(
"WorkOrder")
802 .replace(QRegExp(
"([a-z0-9])([A-Z])"),
"\\1 \\2");
803 qWarning() << QString(
"WorkOrder::bestText(): Work order [%1] has no QUndoCommand text")
820 QMutexLocker locker(
project()->workOrderMutex());
831 QMutexLocker locker(
project()->workOrderMutex());
842 QMutexLocker locker(
project()->workOrderMutex());
855 QMutexLocker locker(
project()->workOrderMutex());
865 QMutexLocker locker(
project()->workOrderMutex());
884 QMutexLocker locker(
project()->workOrderMutex());
885 return m_status == WorkOrderRedoing;
894 QMutexLocker locker(
project()->workOrderMutex());
895 return m_status == WorkOrderRedone;
904 QMutexLocker locker(
project()->workOrderMutex());
905 return m_status == WorkOrderUndoing;
914 QMutexLocker locker(
project()->workOrderMutex());
915 return m_status == WorkOrderUndone;
925 QMutexLocker locker(
project()->workOrderMutex());
935 QMutexLocker locker(
project()->workOrderMutex());
945 QMutexLocker locker(
project()->workOrderMutex());
955 QMutexLocker locker(
project()->workOrderMutex());
956 QString result =
toString(m_status);
964 result += tr(
" (elapsed: %1:%2)").arg(minutes).arg(seconds, 2, 10, QChar(
'0'));
976 QMutexLocker locker(
project()->workOrderMutex());
989 statusString = statusString.toUpper();
993 possibleResult <= WorkOrderLastStatus;
995 if (statusString ==
toString(possibleResult).toUpper()) {
996 result = possibleResult;
1013 case WorkOrderUnknownStatus:
1014 result = tr(
"Unknown");
1016 case WorkOrderNotStarted:
1017 result = tr(
"Not Started");
1019 case WorkOrderRedoing:
1020 result = tr(
"In Progress");
1022 case WorkOrderRedone:
1023 result = tr(
"Completed");
1025 case WorkOrderUndoing:
1026 result = tr(
"Undoing");
1028 case WorkOrderUndone:
1029 result = tr(
"Undone");
1032 result = tr(
"Finished");
1045 m_queuedAction = RedoQueuedAction;
1049 bool mustQueueThisRedo =
false;
1053 while (current->
previous() && !dependency) {
1058 connect(possibleDependency, SIGNAL(finished(
WorkOrder *)),
1060 dependency = possibleDependency;
1061 mustQueueThisRedo =
true;
1071 mustQueueThisRedo =
true;
1077 mustQueueThisRedo =
true;
1082 m_queuedAction = RedoQueuedAction;
1084 QString queueStatusText;
1087 QString dependencyText = dependency->
bestText();
1089 if (dependencyText.count() > 5) {
1090 dependencyText = dependencyText.mid(0, 5) +
"...";
1093 queueStatusText = tr(
"Wait for [%1]").arg(dependencyText);
1096 queueStatusText = tr(
"Wait for images");
1099 queueStatusText = tr(
"Wait for shapes");
1108 if (m_queuedAction == NoQueuedAction) {
1109 m_status = WorkOrderRedoing;
1110 emit statusChanged(
this);
1148 m_queuedAction = UndoQueuedAction;
1151 if (!
isUndone() && m_status != WorkOrderNotStarted) {
1154 while (current->
next() && !dependency) {
1156 current->
next()->m_status != WorkOrderNotStarted) {
1159 m_queuedAction = UndoQueuedAction;
1160 dependency = current->
next();
1163 current = current->
next();
1167 QString prevText = dependency->
bestText();
1169 if (prevText.count() > 5) {
1170 prevText = prevText.mid(0, 5) +
"...";
1175 m_progressBar->setText(tr(
"Undo after [%1]").arg(prevText));
1179 if (m_queuedAction == NoQueuedAction) {
1180 m_status = WorkOrderUndoing;
1181 emit statusChanged(
this);
1267 emit statusChanged(
this);
1303 "This work order no longer has a project.", _FILEINFO_);
1328 QMutexLocker locker(
project()->workOrderMutex());
1338 QMutexLocker locker(
project()->workOrderMutex());
1348 QMutexLocker locker(
project()->workOrderMutex());
1378 QMutexLocker locker(
project()->workOrderMutex());
1481 foreach (
Image *image, *m_imageList) {
1487 connect(image, SIGNAL(destroyed(
QObject *)),
1504 foreach (
Shape *shape, *m_shapeList) {
1510 connect(shape, SIGNAL(destroyed(
QObject *)),
1525 emit creatingProgress(
this);
1549 else if (
isUndone() || m_status == WorkOrderNotStarted) {
1577 m_queuedAction = NoQueuedAction;
1579 if (queued == RedoQueuedAction && m_status != WorkOrderRedone) {
1582 else if (queued == UndoQueuedAction && m_status != WorkOrderUndone) {
1599 finishedStatus = WorkOrderUndone;
1603 (this->*postMethod)();
1605 m_status = finishedStatus;
1612 emit statusChanged(
this);
1614 emit finished(
this);
1703 const QString &qName,
const QXmlAttributes &atts) {
1704 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
1705 if (localName ==
"workOrder") {
1706 QString actionText = atts.value(
"actionText");
1707 QString undoText = atts.value(
"undoText");
1709 QString statusStr = atts.value(
"status");
1711 if (!actionText.isEmpty()) {
1712 ((
QAction *)m_workOrder)->setText(actionText);
1715 if (!undoText.isEmpty()) {
1720 m_workOrder->m_executionTime = QDateTime::fromString(
executionTime);
1723 if (!statusStr.isEmpty()) {
1727 if (m_workOrder->createsCleanState()) {
1731 m_workOrder->m_status = WorkOrderRedone;
1735 else if (localName ==
"dataValue") {
1736 m_workOrder->m_internalData.append(atts.value(
"value"));
1738 else if (localName ==
"context") {
1739 if (atts.value(
"value") ==
"ProjectContext") {
1740 m_workOrder->m_context = ProjectContext;
void setProgressToFinalText()
Sets the ProgressBar to display the final status of the operation.
bool isSynchronous() const
Returns true if this work order is run synchronously, otherwise false.
bool isUndoable() const
Returns true if this work order is undoable, otherwise false.
virtual bool dependsOn(WorkOrder *other) const
Indicate workorder dependency This is a virtual function whose role in child classes is to determine ...
FileItemQsp m_fileItem
A QSharedPointer to the FileItem.
bool isInStableState() const
Determines if the WorkOrder is in a stable state, or if it's busy doing something.
virtual bool setupExecution()
This sets up the state for the work order.
bool isRedone() const
Returns the WorkOrder redone status.
void addCloneToProject()
Runs a copy of the current WorkOrder and stores it in the project.
bool isGuiCamera() const
Returns true if a GuiCameraQsp is stored in the data of the item.
int m_progressRangeMinValue
The miniumum value of the Progess Bar.
Provide Undo/redo abilities, serialization, and history for an operation.
QStringList m_imageIds
A QStringList of unique image identifiers for all of the images this WorkOrder is dealing with.
Shape * shape(QString id)
Return a shape given its id.
bool isControl() const
Returns true if a Control is stored in the data of the item.
GuiCameraQsp m_guiCamera
A QSharedPointer to the GuiCamera (the Camera object but encapsulated within a Gui framework.
Image * image() const
Returns the Image stored in the data of the item.
QPointer< QTimer > m_progressBarUpdateTimer
A pointer to the QTimer which updates the ProgressBar.
int progressMax() const
Gets the maximum value of the progress range of the WorkOrder.
bool isRedoing() const
Returns the redoing status of this WorkOrder.
virtual void redo()
Starts (or enqueues) a redo.
QPointer< ControlList > controlList()
Returns the Control List for this WorkOrder (a list of control networks).
virtual bool isExecutable(Context)
Re-implement this method if your work order utilizes controls for data in order to operate.
ImageList * imageList()
Returns a pointer to the ImageList for this WorkOrder.
CorrelationMatrix correlationMatrix() const
Returns the CorrelationMatrix stored the item.
WorkOrder * m_workOrder
This is a pointer to the WorkOrder the XmlHandler is filling with information it parses from an XML f...
void updateProgress()
Updates the progress bar.
bool m_isSavedToHistory
Set the work order to be shown in the HistoryTreeWidget.
QStringList m_shapeIds
A QStringList of unique shape identifiers for all of the shapes this WorkOrder is dealing with.
Maintains a list of Controls so that control nets can easily be copied from one Project to another,...
@ Unknown
A type of error that cannot be classified as any of the other error types.
virtual void postExecution()
Perform any necessary actions after execution of a workorder.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
void setInternalData(QStringList data)
Sets the internal data for this WorkOrder.
bool isTargetBody() const
Returns true if a TargetBodyQsp is stored in the data of the item.
Shape * shape() const
Returns the Shape stored in the data of the item.
int progressMin() const
Gets the minimum value of the progress range of the WorkOrder.
static QString toString(WorkOrderStatus)
Gets the current status of the WorkOrder.
void save(QXmlStreamWriter &stream) const
: Saves a WorkOrder to a data stream.
Directory * directory() const
return the workorder project directory Returns the Directory object of the Project this WorkOrder is ...
WorkOrder * next() const
Gets the next WorkOrder.
void append(Image *const &value)
Appends an image to the image list.
bool isCorrelationMatrix() const
Returns true if a CorrelationMatrix is stored in the data of the item.
bool createsCleanState() const
Returns the CleanState status (whether the Project has been saved to disk or not).
QSharedPointer< TargetBody > TargetBodyQsp
Defines A smart pointer to a TargetBody obj.
XmlHandler(WorkOrder *workOrder)
Passes a pointer to a WorkOrder to the WorkOrder::XmlHandler class.
bool isControlList() const
Returns true if a ControlList is stored in the data of the item.
bool modifiesDiskState() const
Returns the modified disk state.
Control * control() const
Returns the Control stored in the data of the item.
void read(XmlStackedHandlerReader *xmlReader)
Read this work order's data from disk.
void listenForShapeDestruction()
Checks to see if we have lost any shapes in the ShapeList.
bool m_isSynchronous
This is defaulted to true.
bool isShape() const
Returns true if an Shape is stored in the data of the item.
Manage a stack of content handlers for reading XML files.
This is a container for the correlation matrix that comes from a bundle adjust.
The main project for ipce.
Directory * directory() const
Returns the directory associated with this Project.
bool isFinished() const
Returns the finished state of this WorkOrder.
Internalizes a list of images and allows for operations on the entire list.
ShapeList * shapeList()
@briefReturns a pointer to the ShapeList for this WorkOrder.
void addToProject(WorkOrder *)
This executes the WorkOrder and stores it in the project.
void disableWorkOrder()
Disables the work order.
QStringList m_internalData
A QStringList of internal properties for this WorkOrder.
bool isImageList() const
Returns true if an ImageList is stored in the data of the item.
QPointer< WorkOrder > m_previousWorkOrder
A pointer to the previous WorkOrder in the queue.
WorkOrder(Project *project)
Create a work order that will work with the given project.
QString id() const
Get a unique, identifying string associated with this shape.
QPointer< QFutureWatcher< void > > m_futureWatcher
A pointer to a QFutureWatcher object which monitors a QFuture object using signals and slots.
This class is used for processing an XML file containing information about a WorkOrder.
int m_progressValue
The current value of the Progress Bar.
bool m_isUndoable
Set the workorder to be undoable/redoable This is defaulted to true - his will allow the workorder to...
bool isSavedToHistory() const
Returns true if this work order is to be shown in History, otherwise false.
bool isShapeList() const
Returns true if an ShapeList is stored in the data of the item.
virtual void setData(Context)
Sets the context data for this WorkOrder.
GuiCameraQsp guiCamera() const
Returns the GuiCameraQsp stored in the data of the item.
bool isImage() const
Returns true if an Image is stored in the data of the item.
QString statusText() const
WorkOrder::statusText.
bool isUndone() const
Returns the WorkOrder undo status.
TargetBodyQsp targetBody()
WorkOrder::targetBody.
QTime * m_elapsedTimer
A QTime object holding the excecution time of the WorkOrder.
QDateTime m_executionTime
This is the date/time that setupExecution() was called.
ShapeList * shapeList() const
Returns the ShapeList stored in the data of the item.
bool isFileItem() const
Returns true if a FileItemQsp is stored in the data of the item.
virtual void postUndoExecution()
Perform any steps necessary after an undo of a workorder.
QueuedWorkOrderAction
This enum describes the current state of a Queued WorkOrder.
virtual void undo()
Starts (or enqueues) an undo.
QPointer< QTimer > m_progressBarDeletionTimer
A pointer to the ProgressBar deletion timer.
This represents a shape in a project-based GUI interface.
Template * getTemplate()
WorkOrder::getTemplate.
Project * project() const
Returns the Project this WorkOrder is attached to.
FileItemQsp fileItem() const
Returns the FileItemQsp stored in the data of the item.
QMutex * m_transparentConstMutex
This is used to protect the integrity of data the WorkOrder is working on so that only one thread at ...
Template * m_template
A QSharedPointer to the Template (A Template object but encapsulated within a Gui framework.
virtual void undoExecution()
Execute the steps necessary to undo this workorder.
int progressValue() const
Gets the current progress value of the WorkOrder.
WorkOrder * previous() const
Gets the previous WorkOrder.
Internalizes a list of shapes and allows for operations on the entire list.
bool m_createsCleanState
This is defaulted to false.
This represents a cube in a project-based GUI interface.
static WorkOrderStatus fromStatusString(QString)
Attempts to query the current status of the WorkOrder.
virtual ~WorkOrder()
The Destructor.
FileItemQsp fileItem()
WorkOrder::fileItem.
void setNext(WorkOrder *nextWorkOrder)
Sets the next WorkOrder in the sequence.
QPointer< ProgressBar > m_progressBar
A pointer to the ProgressBar.
ControlList * controlList() const
Returns the ControlList stored in the data of the item.
int m_progressRangeMaxValue
The maximum value of the Progess Bar.
bool isProject() const
Returns true if a Project is stored in the data of the item.
void clearImageList()
Clears the list of images.
void setProgressRange(int, int)
Sets the progress range of the WorkOrder.
void startRedo()
WorkOrder::startRedo This function is currently empty.
QPointer< Project > m_project
A pointer to the Project this WorkOrder is attached to.
Image * image(QString id)
Return an image given its id.
@ Programmer
This error is for when a programmer made an API call that was illegal.
void listenForImageDestruction()
Checks to see if we have lost any images in the ImageList.
QString bestText() const
Generate unique action names We don't use action text anymore because Directory likes to rename our a...
QPointer< WorkOrder > m_nextWorkOrder
A pointer to the next WorkOrder in the queue.
void clearShapeList()
Clears the list of shapes.
GuiCameraQsp guiCamera()
WorkOrder::guiCamera.
void executionFinished()
Signals the Project that the WorkOrder is finished, deletes the update time for the Progress bar,...
QSharedPointer< FileItem > FileItemQsp
A FileItem smart pointer.
@ WorkOrderFinished
This is used for work orders that will not undo or redo (See createsCleanState())
QString id() const
Get a unique, identifying string associated with this image.
void setProgressValue(int)
Sets the current progress value for the WorkOrder.
ProgressBar * progressBar()
Returns the ProgressBar.
ImageList * imageList() const
Returns the ImageList stored in the data of the item.
TargetBodyQsp m_targetBody
A QSharedPointer to the TargetBody (A Target object but encapsulated within a Gui framework.
WorkOrderStatus
This enumeration is used by other functions to set and retrieve the current state of the WorkOrder.
This is free and unencumbered software released into the public domain.
void resetProgressBar()
Resets the ProgressBar.
QStringList internalData() const
Gets the internal data for this WorkOrder.
void append(Shape *const &value)
Appends an shape to the shape list.
virtual void execute()
Execute the workorder.
void setModifiesDiskState(bool changesProjectOnDisk)
CorrelationMatrix correlationMatrix()
Returns the CorrleationMatrix for this WorkOrder.
void setPrevious(WorkOrder *previousWorkOrder)
Sets the previous WorkOrder in the sequence.
void setCreatesCleanState(bool createsCleanState)
Declare that this work order is saving the project.
void attemptQueuedAction()
Attempts to execute an action on the action queue.
Context
This enumeration is for recording the context of the current Workorder (whether it is part of a proje...
bool m_modifiesDiskState
This is defaulted to false.
QSharedPointer< GuiCamera > GuiCameraQsp
GuiCameraQsp Represents a smart pointer to a GuiCamera object.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
The XML reader invokes this method at the start of every element in the XML document.
Template * getTemplate() const
Returns the Template stored in the data of the item.
bool isUndoing() const
Returns the WorkOrderUndoing state.
void enableWorkOrder()
Enables the work order.
QDateTime executionTime() const
Gets the execution time of this WorkOrder.
double m_secondsElapsed
The seconds that have elapsed since the WorkOrder started executing.
This is free and unencumbered software released into the public domain.
TargetBodyQsp targetBody() const
Returns the TargetBodyQsp stored in the data of the item.
Represents an item of a ProjectItemModel in Qt's model-view framework.