Loading [MathJax]/jax/output/NativeMML/config.js
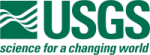 |
Isis 3 Programmer Reference
|
8 #include <QMutexLocker>
9 #include <QScopedPointer>
12 #include <QXmlStreamWriter>
14 #include <geos/geom/MultiPolygon.h>
15 #include <geos/io/WKTReader.h>
16 #include <geos/io/WKTWriter.h>
20 #include "CubeAttribute.h"
21 #include "DisplayProperties.h"
25 #include "ImagePolygon.h"
27 #include "ObservationNumber.h"
28 #include "PolygonTools.h"
30 #include "SerialNumber.h"
32 #include "XmlStackedHandlerReader.h"
68 m_id =
new QUuid(QUuid::createUuid());
101 m_id =
new QUuid(QUuid::createUuid());
195 QString pvlFileName = ((
IString)pvl[
"FileName"][0]).ToQt();
198 tr(
"Tried to load Image [%1] with properties/information from [%2].")
206 QByteArray hexValues(pvl[
"ID"][0].toLatin1());
207 QDataStream valuesStream(QByteArray::fromHex(hexValues));
208 valuesStream >> *
m_id;
233 dataBuffer.open(QIODevice::ReadWrite);
235 QDataStream idStream(&dataBuffer);
240 output +=
PvlKeyword(
"ID", QString(dataBuffer.data().toHex()));
263 QString blobType = example.
Type();
264 QString blobName = example.
Name();
268 for (
int i = 0; i < labels.
objects(); i++) {
271 if (obj.
isNamed(blobType) && obj.
hasKeyword(
"Name") && obj[
"Name"][0] == blobName)
384 m_id =
new QUuid(
id);
419 IString msg =
"Could not read the footprint from cube [" +
421 "sure footprintinit has been run";
446 return m_id->toString().remove(QRegExp(
"[{}]"));
548 QScopedPointer<Cube> newExternalLabel(
561 newExternalLabel->relocateDnData(newDnFileName.
name());
569 newExternalLabel->relocateDnData(newExternalCubeFileName);
592 tr(
"Could not remove file [%1]").arg(
m_fileName),
598 if (!QFile::remove(cubFile.
expanded() ) ) {
600 tr(
"Could not remove file [%1]").arg(
m_fileName),
628 stream.writeStartElement(
"image");
630 stream.writeAttribute(
"id",
m_id->toString());
672 stream.writeStartElement(
"footprint");
674 geos::io::WKTWriter wktWriter;
675 stream.writeCharacters(QString::fromStdString(wktWriter.write(
m_footprint)));
677 stream.writeEndElement();
682 stream.writeEndElement();
696 original.
dir().dirName() +
"/" + original.
name());
707 QMutexLocker lock(cameraMutex);
713 if (sampleStepSize <= 0) sampleStepSize = 1;
716 if (lineStepSize <= 0) lineStepSize = 1;
718 imgPoly.
Create(*
cube(), sampleStepSize, lineStepSize);
721 tr(
"Warning: Polygon re-calculated for [%1] which can be very slow")
735 bool hasCamStats =
false;
738 for (
int i = 0; !hasCamStats && i < label.
objects(); i++) {
742 if (obj.
name() ==
"Table") {
743 if (obj[
"Name"][0] ==
"CameraStatistics") {
755 int numRecords = camStatsTable.
Records();
756 for (
int recordIndex = 0; recordIndex < numRecords; recordIndex++) {
761 QString recordName((QString)record[
"Name"]);
762 double avgValue = (double)record[
"Average"];
764 if (recordName ==
"AspectRatio") {
767 else if (recordName ==
"Resolution") {
770 else if (recordName ==
"EmissionAngle") {
773 else if (recordName ==
"IncidenceAngle") {
776 else if (recordName ==
"LineResolution") {
779 else if (recordName ==
"LocalRadius") {
782 else if (recordName ==
"NorthAzimuth") {
785 else if (recordName ==
"PhaseAngle") {
788 else if (recordName ==
"SampleResolution") {
794 for (
int i = 0; i < label.
objects(); i++) {
852 const QString &qName,
const QXmlAttributes &atts) {
855 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
856 if (localName ==
"image") {
857 QString
id = atts.value(
"id");
858 QString
fileName = atts.value(
"fileName");
859 QString instrumentId = atts.value(
"instrumentId");
860 QString spacecraftName = atts.value(
"spacecraftName");
862 QString aspectRatioStr = atts.value(
"aspectRatio");
863 QString resolutionStr = atts.value(
"resolution");
864 QString emissionAngleStr = atts.value(
"emissionAngle");
865 QString incidenceAngleStr = atts.value(
"incidenceAngle");
866 QString lineResolutionStr = atts.value(
"lineResolution");
867 QString localRadiusStr = atts.value(
"localRadius");
868 QString northAzimuthStr = atts.value(
"northAzimuth");
869 QString phaseAngleStr = atts.value(
"phaseAngle");
870 QString sampleResolutionStr = atts.value(
"sampleResolution");
873 delete m_image->m_id;
874 m_image->m_id = NULL;
875 m_image->m_id =
new QUuid(
id.toLatin1());
879 m_image->m_fileName = m_imageFolder.expanded() +
"/" +
fileName;
882 if (!instrumentId.isEmpty()) {
883 m_image->m_instrumentId = instrumentId;
886 if (!spacecraftName.isEmpty()) {
887 m_image->m_spacecraftName = spacecraftName;
890 if (!aspectRatioStr.isEmpty()) {
891 m_image->m_aspectRatio = aspectRatioStr.toDouble();
894 if (!resolutionStr.isEmpty()) {
895 m_image->m_resolution = resolutionStr.toDouble();
898 if (!emissionAngleStr.isEmpty()) {
902 if (!incidenceAngleStr.isEmpty()) {
906 if (!lineResolutionStr.isEmpty()) {
907 m_image->m_lineResolution = lineResolutionStr.toDouble();
910 if (!localRadiusStr.isEmpty()) {
914 if (!northAzimuthStr.isEmpty()) {
918 if (!phaseAngleStr.isEmpty()) {
922 if (!sampleResolutionStr.isEmpty()) {
923 m_image->m_sampleResolution = sampleResolutionStr.toDouble();
926 else if (localName ==
"displayProperties") {
948 return XmlStackedHandler::characters(ch);
964 const QString &qName) {
965 if (localName ==
"footprint" && !m_characters.isEmpty()) {
966 geos::io::WKTReader wktReader(*globalFactory);
968 wktReader.read(m_characters.toStdString()));
970 else if (localName ==
"image" && !m_image->m_footprint) {
972 m_image->initFootprint(&mutex);
973 m_image->closeCube();
977 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
Cube * m_cube
The cube associated with this Image.
Distance m_localRadius
Local radius of the image.
Angle m_phaseAngle
Phase angle for the image.
XmlHandler(Image *image, FileName imageFolder)
Create an XML Handler (reader) that can populate the Image class data.
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
virtual QString fileName() const
Returns the opened cube's filename.
@ Io
A type of error that occurred when performing an actual I/O operation.
Contains Pvl Groups and Pvl Objects.
void print() const
Prints a string representation of this exception to stderr.
A single keyword-value pair.
QString serialNumber()
Returns the serial number of the Cube.
QString Type() const
Accessor method that returns a string containing the Blob type.
double m_resolution
Resolution of the image.
geos::geom::MultiPolygon * m_footprint
A 0-360 ocentric lon,lat degrees footprint of this Image.
QString name() const
Returns the name of the file excluding the path and the attributes in the file name.
double lineResolution() const
Get the line resolution of this image, as calculated and attached by camstats.
File name manipulation and expansion.
@ Unknown
A type of error that cannot be classified as any of the other error types.
ImagePolygon readFootprint() const
Read the footprint polygon for the Cube.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
Blob toBlob() const
Serialize the ImagePolygon to a Blob.
Image * m_image
Pointer to the Image.
QString m_spacecraftName
Spacecraft name associated with this Image.
static QString Compose(Pvl &label, bool def2filename=false)
Compose a SerialNumber from a PVL.
Cube * copy(FileName newFile, const CubeAttributeOutput &newFileAttributes)
Copies the cube to the new fileName.
bool hasGroup(const QString &name) const
Returns a boolean value based on whether the object has the specified group or not.
ImageDisplayProperties * m_displayProperties
The GUI information for how this Image ought to be displayed.
void initQuickFootprint()
Creates a default ImagePolygon option which is read into the Cube.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
void Create(Cube &cube, int sinc=1, int linc=1, int ss=1, int sl=1, int ns=0, int nl=0, int band=1, bool increasePrecision=false)
Create a Polygon from given cube.
bool initFootprint(QMutex *cameraMutex)
Calculate a footprint for this image.
int objects() const
Returns the number of objects.
Manipulate and parse attributes of output cube filenames.
QString fileName() const
Get the file name of the cube that this image represents.
Create cube polygons, read/write polygons to blobs.
Angle phaseAngle() const
Get the phase angle of this image, as calculated and attached by camstats.
FileName m_imageFolder
The Name/path of the image.
PvlObject & object(const int index)
Return the object at the specified index.
QUuid * m_id
A unique ID for this Image (useful for others to reference this Image when saving to disk).
QString imageDataRoot() const
Accessor for the root directory of the image data.
Manage a stack of content handlers for reading XML files.
bool IsSpecial(const double d)
Returns if the input pixel is special.
QString Name() const
Accessor method that returns a string containing the Blob name.
Distance measurement, usually in meters.
The main project for ipce.
double resolution() const
Get the resolution of this image, as calculated and attached by camstats.
Distance localRadius() const
Get the local radius of this image, as calculated and attached by camstats.
FileName externalCubeFileName() const
If this is an external cube label file, this will give you the cube dn file that this label reference...
QString m_observationNumber
The observation number for this image.
void deleteFromDisk()
Delete the image data from disk.
double aspectRatio() const
Get the aspect ratio of this image, as calculated and attached by camstats.
@ Meters
The distance is being specified in meters.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
Angle northAzimuth() const
Get the north azimuth of this image, as calculated and attached by camstats.
Contains multiple PvlContainers.
QDir dir() const
Returns the path of the file's parent directory as a QDir object.
geos::geom::MultiPolygon * createFootprint(QMutex *cameraMutex)
Calculates a footprint for an Image using the camera or projection information.
bool isNamed(const QString &match) const
Returns whether the given string is equal to the container name or not.
Angle m_northAzimuth
North Azimuth for the image.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Write the Image properties out to an XML file.
bool hasKeyword(const QString &kname, FindOptions opts) const
See if a keyword is in the current PvlObject, or deeper inside other PvlObjects and Pvlgroups within ...
geos::geom::MultiPolygon * footprint()
Get the footprint of this image (if available).
Class for storing Table blobs information.
Cube * cube()
Get the Cube pointer associated with this display property.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
bool isValid() const
Test if this distance has been initialized or not.
void closeCube()
Cleans up the Cube pointer.
QString newProjectRoot() const
Get the top-level folder of the new project.
QString displayName() const
Returns the display name.
void fromPvl(const PvlObject &pvl)
Read the image settings from a Pvl.
static QString Compose(Pvl &label, bool def2filename=false)
Compose a ObservationNumber from a PVL.
bool isFootprintable() const
Test to see if it's possible to create a footprint from this image.
IO Handler for Isis Cubes.
QString name() const
Returns the container name.
double sampleResolution() const
Get the sample resolution of this image, as calculated and attached by camstats.
void copyToNewProjectRoot(const Project *project, FileName newProjectRoot)
Copy the cub/ecub files associated with this image into the new project.
This represents a cube in a project-based GUI interface.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
The XML reader invokes this method at the end of every element in the XML document.
Angle m_incidenceAngle
Incidence angle of the image.
Defines an angle and provides unit conversions.
QString toString() const
Returns a QString of the full file name including the file path, excluding the attributes with any Is...
QString projectRoot() const
Get the top-level folder of the project.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
Read mage class attributes.
const double Null
Value for an Isis Null pixel.
void updateFileName(Project *)
Change the on-disk file name for this cube to be where the image ought to be in the given project.
Image(QString imageFileName, QObject *parent=0)
Create an image from a cube file on disk.
ImageDisplayProperties * displayProperties()
Get the display (GUI) properties (information) associated with this image.
QString m_serialNumber
The serial number for this image.
Angle m_emissionAngle
Emmission angle of the image.
QString m_instrumentId
Instrument id associated with this Image.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
bool storesDnData() const
This method returns a boolean value.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
QString id() const
Get a unique, identifying string associated with this image.
PvlObject toPvl() const
Convert to Pvl for project files.
double m_lineResolution
Line resolution of the image.
virtual bool characters(const QString &ch)
This implementation of a virtual function calls QXmlDefaultHandler::characters(QString &ch) which in ...
double meters() const
Get the distance in meters.
void initCamStats()
Checks to see if the Cube label contains Camera Statistics.
PvlObject toPvl() const
Convert this Image to PVL.
QString m_fileName
The on-disk file name of the cube associated with this Image.
Adds specific functionality to C++ strings.
Process XML in a stack-oriented fashion.
Angle incidenceAngle() const
Get the incidence angle of this image, as calculated and attached by camstats.
int Records() const
Returns the number of records.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Output format:
Angle emissionAngle() const
Get the emission angle of this image, as calculated and attached by camstats.
FileName setExtension(const QString &extension) const
Sets all current file extensions to a new extension in the file name.
QString observationNumber()
Returns the observation number of the Cube.
static QString imageDataRoot(QString projectRoot)
Appends the root directory name 'images' to the project .
~Image()
Clean up this image.
double m_sampleResolution
Sample resolution of the image.
geos::geom::MultiPolygon * Polys()
Return a geos Multipolygon.
void setId(QString id)
Override the automatically generated ID with the given ID.
QString path() const
Returns the path of the file name.
@ Radians
Radians are generally used in mathematical equations, 0-2*PI is one circle, however these are more di...
This is free and unencumbered software released into the public domain.
QString ToQt() const
Retuns the object string as a QString.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
SpiceInt * m_bodyCode
The NaifBodyCode value, if it exists in the labels.
double m_aspectRatio
Aspect ratio of the image.
This is the GUI communication mechanism for cubes.
double radians() const
Convert an angle to a double.