File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
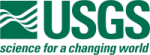 |
Isis 3 Programmer Reference
|
15 #include <QInputDialog>
17 #include <QProgressDialog>
18 #include <QtConcurrentMap>
19 #include <QXmlStreamWriter>
22 #include "IException.h"
25 #include "XmlStackedHandlerReader.h"
92 foreach (QString fileName, fileNames) {
119 for (
int i = 0; i < count(); i++) {
120 result.
add((*
this)[i]->fileName());
135 emit countChanged(count());
148 emit countChanged(count());
158 bool countChanging = count();
161 emit countChanged(count());
177 emit countChanged(count());
196 emit countChanged(count());
212 emit countChanged(count());
228 emit countChanged(count());
242 emit countChanged(count());
256 emit countChanged(count());
270 emit countChanged(count());
287 emit countChanged(count());
303 emit countChanged(count());
314 emit countChanged(count());
325 emit countChanged(count());
342 emit countChanged(count());
359 if (count() != other.count()) {
360 emit countChanged(count());
376 emit countChanged(count());
390 emit countChanged(count());
404 emit countChanged(count());
423 emit countChanged(count());
442 emit countChanged(count());
461 emit countChanged(count());
480 emit countChanged(count());
495 bool countChanging = (rhs.count() != count());
499 emit countChanged(count());
514 bool countChanging = (rhs.count() != count());
521 emit countChanged(count());
579 foreach (
Shape *shape, *
this) {
617 stream.writeStartElement(
"shapeList");
618 stream.writeAttribute(
"name",
m_name);
619 stream.writeAttribute(
"path",
m_path);
624 if (!settingsFileName.
dir().mkpath(settingsFileName.
path())) {
626 QString(
"Failed to create directory [%1]")
627 .arg(settingsFileName.
path()),
631 QFile shapeListContentsFile(settingsFileName.
toString());
633 if (!shapeListContentsFile.open(QIODevice::ReadWrite | QIODevice::Truncate)) {
635 QString(
"Unable to save shape information for [%1] because [%2] could not be opened for "
641 QXmlStreamWriter shapeDetailsWriter(&shapeListContentsFile);
642 shapeDetailsWriter.setAutoFormatting(
true);
643 shapeDetailsWriter.writeStartDocument();
645 int countWidth = QString(
"%1L").arg(count()).size() - 1;
646 QChar paddingChar(
'0');
648 QLabel *progressLabel =
new QLabel;
650 QProgressDialog progressDialog;
651 progressDialog.setLabel(progressLabel);
652 progressDialog.setRange(-1, count());
653 progressDialog.setValue(-1);
655 shapeDetailsWriter.writeStartElement(
"shapes");
657 QFuture<void *> future = QtConcurrent::mapped(*
this,
660 for (
int i = 0; i < count(); i++) {
661 int newProgressValue = progressDialog.value() + 1;
662 progressLabel->setText(
663 tr(
"Saving Shape Information for [%1] - %L2/%L3 done")
665 .arg(newProgressValue, countWidth, 10, paddingChar)
667 progressDialog.setValue(newProgressValue);
671 progressLabel->setText(tr(
"Finalizing..."));
672 progressDialog.setRange(0, 0);
673 progressDialog.setValue(0);
675 foreach (
Shape *shape, *
this) {
676 shape->
save(shapeDetailsWriter, project, newProjectRoot);
679 shapeDetailsWriter.writeEndElement();
681 shapeDetailsWriter.writeEndDocument();
683 stream.writeEndElement();
756 m_shapeList = shapeList;
768 const QString &qName,
const QXmlAttributes &atts) {
769 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
770 if (localName ==
"shapeList") {
771 QString
name = atts.value(
"name");
772 QString
path = atts.value(
"path");
774 if (!
name.isEmpty()) {
775 m_shapeList->setName(
name);
778 if (!
path.isEmpty()) {
779 m_shapeList->setPath(
path);
782 else if (localName ==
"shape") {
783 m_shapeList->append(
new Shape(m_project->shapeDataRoot() +
"/" + m_shapeList->path(),
802 const QString &qName) {
803 if (localName ==
"shapeList") {
808 reader.setErrorHandler(&handler);
810 QString shapeListXmlPath = m_project->shapeDataRoot() +
"/" + m_shapeList->path() +
812 QFile file(shapeListXmlPath);
814 if (!file.open(QFile::ReadOnly)) {
816 QString(
"Unable to open [%1] with read access")
817 .arg(shapeListXmlPath),
821 QXmlInputSource xmlInputSource(&file);
822 if (!reader.parse(xmlInputSource))
824 tr(
"Failed to open shape list XML [%1]").arg(shapeListXmlPath),
828 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
@ Io
A type of error that occurred when performing an actual I/O operation.
void insert(int i, Shape *const &value)
Inserts an shape into the shape list at an index.
void deleteFromDisk()
Delete the shape data from disk.
Shape * takeLast()
Removes and returns the last shape.
ShapeList & operator<<(const QList< Shape * > &other)
Appends a list of shapes to the end of the shape list.
File name manipulation and expansion.
CopyShapeDataFunctor(const Project *project, FileName newProjectRoot)
Constructor for CopyShapeDataFunctor.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
~CopyShapeDataFunctor()
Destructor for CopyShapeDataFunctor.
Shape * takeFirst()
Removes and returns the first shape.
void setName(QString newName)
Set the human-readable name of this shape list.
void * operator()(Shape *const &shapeToCopy)
Copies the cub/ecub files for an shape into m_project.
void clear()
Clears the shape list.
static QString shapeDataRoot(QString projectRoot)
Appends the root directory name 'shapes' to the project .
void push_front(Shape *const &value)
Prepends an shape to the beginning of the shape list.
ShapeList & operator+=(const QList< Shape * > &other)
Appends a list of shapes to the end of the shape list.
iterator erase(iterator pos)
Erases a single shape from the shape list.
This class is used to read an shapes.xml file into an shape list.
FileName m_newProjectRoot
This stores the path to the root of the project that is going to be copied to.
Serial Number list generator.
Manage a stack of content handlers for reading XML files.
void copyToNewProjectRoot(const Project *project, FileName newProjectRoot)
Copy the cub/ecub files associated with this shape into the new project.
ShapeList & operator=(const QList< Shape * > &rhs)
Assigns another list of shapes to the shape list.
void setPath(QString newPath)
Set the relative path (from the project root) to this shape list's folder.
Shape * takeAt(int i)
Removes the shape at an index and returns it.
void deleteFromDisk(Project *project)
Delete all of the contained Shapes from disk.
The main project for ipce.
QString m_path
This stores the directory name that contains the shapes in this shape list.
CopyShapeDataFunctor & operator=(const CopyShapeDataFunctor &rhs)
Assignment operator for CopyShapeDataFunctor.
bool removeOne(Shape *const &value)
Removes the first occurance of an shape.
SerialNumberList serialNumberList()
Creates a SerialNumberList from the shape list.
QDir dir() const
Returns the path of the file's parent directory as a QDir object.
void removeFirst()
Removes the shape at the front of the shape list.
QString shapeDataRoot() const
Accessor for the root directory of the shape model data.
XmlHandler(ShapeList *shapeList, Project *project)
Create an XML Handler (reader) that can populate the Shape list class data.
void add(const QString &filename, bool def2filename=false)
Adds a new filename / serial number pair to the SerialNumberList.
This represents a shape in a project-based GUI interface.
void swap(QList< Shape * > &other)
Swaps the shape list with another list of shapes.
void push_back(Shape *const &value)
Appends an shape to the end of the shape list.
Internalizes a list of shapes and allows for operations on the entire list.
void removeAt(int i)
Removes the shape at an index.
void prepend(Shape *const &value)
Inserts an shape at the beginning of the shape list.
QString toString() const
Returns a QString of the full file name including the file path, excluding the attributes with any Is...
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Convert this shape list into XML format for saving/restoring capabilities.
This functor is used for copying the shapes between two projects quickly.
QString name() const
Get the human-readable name of this shape list.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
Handle an XML start element.
ShapeList(QString name, QString path, QObject *parent=NULL)
Creates an shape list from an shape list name and path (does not read Shapes).
void append(Shape *const &value)
Appends an shape to the shape list.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Output format:
QString m_name
This stores the shape list's name.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
Handle an XML end element.
QString original() const
Returns the full file name including the file path.
int removeAll(Shape *const &value)
Removes all occurances of an shape.
const Project * m_project
This stores the name of the project that is going to be copied to.
void removeLast()
Removes the shape at the end of the shape list.
QString path() const
Returns the path of the file name.
This is free and unencumbered software released into the public domain.
QString path() const
Get the path to the shapes in the shape list (relative to project root).