Loading [MathJax]/jax/output/NativeMML/config.js
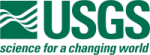 |
Isis 3 Programmer Reference
|
17 #include "ControlPoint.h"
21 #include "Longitude.h"
22 #include "XmlStackedHandler.h"
30 class QXmlStreamWriter;
44 class ShapeDisplayProperties;
45 class XmlStackedHandlerReader;
77 explicit Shape(QString shapeFileName,
QObject *parent = 0);
88 ControlPoint::SurfacePointSource::Source surfacePointSource();
89 ControlPoint::RadiusSource::Source radiusSource();
91 ShapeType shapeType();
96 const geos::geom::MultiPolygon *
footprint()
const;
97 void setId(QString
id);
114 void save(QXmlStreamWriter &stream,
const Project *project,
FileName newProjectRoot)
const;
123 void initMemberData();
130 void initQuickFootprint();
142 virtual bool startElement(
const QString &namespaceURI,
const QString &localName,
143 const QString &qName,
const QXmlAttributes &atts);
144 virtual bool characters(
const QString &ch);
145 virtual bool endElement(
const QString &namespaceURI,
const QString &localName,
146 const QString &qName);
153 QString m_characters;
169 ControlPoint::SurfacePointSource::Source m_surfacePointSource;
170 ControlPoint::RadiusSource::Source m_radiusSource;
171 ShapeType m_shapeType;
203 double m_aspectRatio;
205 Angle m_emissionAngle;
206 Angle m_incidenceAngle;
207 double m_lineResolution;
209 Angle m_northAzimuth;
211 double m_sampleResolution;
214 QString m_targetName;
215 QString m_projectionName;
222 double m_pixelResolution;
QString m_spacecraftName
Spacecraft name associated with this Shape.
void setId(QString id)
Override the automatically generated ID with the given ID.
ShapeDisplayProperties * displayProperties()
Get the display (GUI) properties (information) associated with this shape.
Contains Pvl Groups and Pvl Objects.
void deleteFromDisk()
Delete the shape data from disk.
This class is designed to encapsulate the concept of a Latitude.
File name manipulation and expansion.
void closeCube()
Cleans up the Cube *.
geos::geom::MultiPolygon * createFootprint(QMutex *cameraMutex)
Calculate a footprint for an Shape using the camera or projection information.
ShapeDisplayProperties * m_displayProperties
The GUI information for how this Shape ought to be displayed.
geos::geom::MultiPolygon * footprint()
Get the footprint of this shape (if available).
Angle incidenceAngle() const
Get the incidence angle of this shape, as calculated and attached by camstats.
bool isFootprintable() const
Test to see if it's possible to create a footprint from this shape.
Cube * cube()
Get the Cube * associated with this display property.
Angle northAzimuth() const
Get the north azimuth of this shape, as calculated and attached by camstats.
Manage a stack of content handlers for reading XML files.
void copyToNewProjectRoot(const Project *project, FileName newProjectRoot)
Copy the cub/ecub files associated with this shape into the new project.
PvlObject toPvl() const
Convert this Shape to PVL.
QString m_serialNumber
This will always be simply the filename and is created on construction.
This is the GUI communication mechanism for shape objects.
Distance measurement, usually in meters.
The main project for ipce.
This class is designed to encapsulate the concept of a Longitude.
QString fileName() const
Get the file name of the cube that this shape represents.
Angle emissionAngle() const
Get the emission angle of this shape, as calculated and attached by camstats.
QString m_fileName
The on-disk file name of the cube associated with this Shape.
void fromPvl(const PvlObject &pvl)
Read the shape settings from a Pvl.
geos::geom::MultiPolygon * m_footprint
A 0-360 ocentric lon,lat degrees footprint of this Shape.
QString id() const
Get a unique, identifying string associated with this shape.
double aspectRatio() const
Get the aspect ratio of this shape, as calculated and attached by camstats.
Angle phaseAngle() const
Get the phase angle of this shape, as calculated and attached by camstats.
QString m_instrumentId
Instrument id associated with this Shape.
double resolution() const
Get the resolution of this shape, as calculated and attached by camstats.
This represents a shape in a project-based GUI interface.
IO Handler for Isis Cubes.
Distance localRadius() const
Get the local radius of this shape, as calculated and attached by camstats.
Defines an angle and provides unit conversions.
Cube * m_cube
The cube associated with this Shape.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
Handle an XML start element.
Shape(QString shapeFileName, QObject *parent=0)
Create an Shape from a cube file on disk.
void updateFileName(Project *)
Change the on-disk file name for this cube to be where the shape ought to be in the given project.
QString serialNumber()
Get the serial number.
XML Handler that parses XMLs in a stack-oriented way.
bool initFootprint(QMutex *cameraMutex)
Calculate a footprint for this shape.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Output format:
SpiceInt * m_bodyCode
The NaifBodyCode value, if it exists in the labels.
QUuid * m_id
A unique ID for this Shape (useful for others to reference this Shape when saving to disk).
XmlHandler(Shape *shape, FileName shapeFolder)
Create an XML Handler (reader) that can populate the Shape class data.
double lineResolution() const
Get the line resolution of this shape, as calculated and attached by camstats.
double sampleResolution() const
Get the sample resolution of this shape, as calculated and attached by camstats.
~Shape()
Clean up this shape.
This is free and unencumbered software released into the public domain.
Q_DECLARE_METATYPE(Isis::PlotWindow *)
We have plot windows as QVariant data types, so here it's enabled.