File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
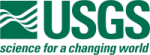 |
Isis 3 Programmer Reference
|
12 #include "Constants.h"
13 #include "IException.h"
16 #include "PvlKeyword.h"
17 #include "RingPlaneProjection.h"
39 Planar::Planar(
Pvl &label,
bool allowDefaults) :
51 if ((allowDefaults) && (!mapGroup.
hasKeyword(
"CenterRingLongitude"))) {
58 if ((allowDefaults) && (!mapGroup.
hasKeyword(
"CenterRingRadius"))) {
72 string message =
"Invalid label group [Mapping]";
90 if (!RingPlaneProjection::operator==(proj))
return false;
176 double azRadians = ringLongitude *
DEG2RAD;
180 if (ringRadius < 0) {
205 double x = ringRadius * cos(deltaAz);
206 double y = ringRadius * sin(deltaAz);
380 double &minY,
double &maxY) {
491 bool allowDefaults) {
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
@ Io
A type of error that occurred when performing an actual I/O operation.
PvlGroup MappingRingRadii()
This function returns the radius keywords that this projection uses.
double m_maximumRingLongitude
Contains the maximum longitude for the entire ground range.
A single keyword-value pair.
virtual PvlGroup Mapping()
This method is used to find the XY range for oblique aspect projections (non-polar projections) by "w...
static double To360Domain(const double lon)
This method converts an ring longitude into the 0 to 360 domain.
const double DEG2RAD
Multiplier for converting from degrees to radians.
double PixelResolution() const
Returns the pixel resolution value from the PVL mapping group in meters/pixel.
QString Version() const
Returns the version of the map projection.
double m_minimumY
See minimumX description.
bool operator==(const Projection &proj)
Compares two Projection objects to see if they are equal.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
void SetXY(double x, double y)
This protected method is a helper for derived classes.
double m_maximumRingRadius
Contains the maximum ring radius for the entire ground range.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
double m_ringRadius
This contain a ring radius value in m.
double CenterRingLongitude() const
Returns the center longitude, in degrees.
PvlGroup m_mappingGrp
Mapping group that created this projection.
void XYRangeCheck(const double ringRadius, const double ringLongitude)
This convience function is established to assist in the development of the XYRange virtual method.
@ Traverse
Search child objects.
double CenterRingRadius() const
Returns the center radius, in meters.
Base class for Map Projections of plane shapes.
double m_minimumX
The data elements m_minimumX, m_minimumY, m_maximumX, and m_maximumY are convience data elements when...
Contains multiple PvlContainers.
bool m_good
Indicates if the contents of m_x, m_y, m_latitude, and m_longitude are valid.
QString Name() const
Returns the name of the map projection, "Planar".
PvlGroup MappingRingLongitudes()
This function returns the azimuth keywords that this projection uses.
virtual PvlGroup MappingRingRadii()
This function returns the ring radius keywords that this projection uses.
double m_ringLongitude
This contain a ring longitude value.
RingLongitudeDirection m_ringLongitudeDirection
An enumerated type indicating the LongitudeDirection read from the labels.
static double To180Domain(const double lon)
This method converts a ring longitude into the -180 to 180 domain.
double m_centerRingLongitude
The center longitude for the map projection.
double m_centerRingRadius
The center radius for the map projection.
void SetComputedXY(double x, double y)
This protected method is a helper for derived classes.
Namespace for the standard library.
~Planar()
Destroys the Planar object.
bool SetGround(const double ringRadius, const double ringLongitude)
This method is used to set the radius/azimuth (assumed to be of the correct RingLongitudeDirection,...
double m_minimumRingRadius
Contains the minimum ring radius for the entire ground range.
PvlGroup Mapping()
This function returns the keywords that this projection uses.
virtual PvlGroup MappingRingLongitudes()
This function returns the ring longitude keywords that this projection uses.
@ Clockwise
Ring longitude values increase in the clockwise direction.
double TrueScaleRingRadius() const
Returns the center radius, in meters.
double m_maximumY
See minimumX description.
double m_minimumRingLongitude
Contains the minimum longitude for the entire ground range.
int m_ringLongitudeDomain
This integer is either 180 or 360 and is read from the labels.
Base class for Map Projections.
double m_maximumX
See minimumX description.
const double RAD2DEG
Multiplier for converting from radians to degrees.
virtual bool HasGroundRange() const
This indicates if the longitude direction type is positive west (as opposed to postive east).
bool SetCoordinate(const double x, const double y)
This method is used to set the projection x/y.
This is free and unencumbered software released into the public domain.
bool XYRange(double &minX, double &maxX, double &minY, double &maxY)
This method is used to determine the x/y range which completely covers the area of interest specified...