File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
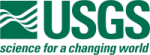 |
Isis 3 Programmer Reference
|
1 #ifndef ProjectItemModel_h
2 #define ProjectItemModel_h
12 #include <QStandardItemModel>
17 class QItemSelectionModel;
27 class BundleSolutionInfo;
147 Qt::DropAction action,
149 const QModelIndex& parent)
const;
168 bool setData(
const QModelIndex &index,
const QVariant &value,
int role);
169 Qt::ItemFlags
flags(
const QModelIndex &index)
const;
195 void onSelectionChanged(
const QItemSelection &selected,
const QItemSelection &deselected);
207 void onRowsInserted(
const QModelIndex &parent,
int start,
int end);
208 void onRowsRemoved(
const QModelIndex &parent,
int start,
int end);
Provides access to data stored in a Project through Qt's model-view framework.
void setItem(int row, ProjectItem *item)
Sets the item at the top-level row.
void onRowsInserted(const QModelIndex &parent, int start, int end)
Slot to connect to the rowsInserted() signal from QAbstractItemModel.
void onControlListAdded(ControlList *controlList)
Slot to connect to the controlListAdded() signal from a Project.
This is free and unencumbered software released into the public domain.
void onSelectionChanged(const QItemSelection &selected, const QItemSelection &deselected)
Slot to connect to the selectionChanged() signal from a selection model.
void projectNameEdited(QString)
This signal is emitted when the project name is edited.
void appendRow(ProjectItem *item)
Appends a top-level item to the model.
void onGuiCamerasAdded(GuiCameraList *cameras)
Slot to connect to the guiCamerasAdded() signal from a Project.
QList< ProjectItem * > selectedBOSSImages()
ProjectItemModel::selectedBOSSImages.
Maintains a list of Controls so that control nets can easily be copied from one Project to another,...
virtual void removeItems(QList< ProjectItem * > items)
Removes a list of items and their children from the model.
QItemSelectionModel * selectionModel()
Returns the internal selection model.
ProjectItemModel(QObject *parent=0)
Constructs an empty model.
void clean()
Used to clean the ProjectItemModel of everything but the headers.
This represents an ISIS control net in a project-based GUI interface.
ProjectItem * item(int row)
Returns the top-level item at the given row.
ProjectItem * itemFromIndex(const QModelIndex &index)
Returns the ProjectItem corresponding to a given QModelIndex.
void itemAdded(ProjectItem *)
This signal is emitted when a ProjectItem is added to the model.
The main project for ipce.
void onControlAdded(Control *control)
Slot to connect to the controlAdded() signal from a project.
~ProjectItemModel()
Destructs the model.
Internalizes a list of images and allows for operations on the entire list.
void onTemplatesAdded(TemplateList *templateList)
Slot connected to the templatesAdded() signal from a project.
void onImagesAdded(ImageList *images)
Slot to connect to the imagesAdded() signal from a Project.
QList< ProjectItem * > selectedItems()
Returns a list of the selected items of the internal selection model.
QItemSelectionModel * m_selectionModel
The internal selection model.
void cleanProject(bool)
This signal is emitted whrn a ProjectItem's name is changed.
Container class for BundleAdjustment results.
void onShapesAdded(ShapeList *shapes)
Slot to connect to the shapesAdded() signal from a Project.
ProjectItem * addProject(Project *project)
Adds a Project to the model.
Internalizes a list of shapes and allows for operations on the entire list.
List for holding TargetBodies.
ProjectItem * findItemData(const QVariant &data, int role=Qt::UserRole+1)
Returns the first item found that contains the given data in the given role or a null pointer if no i...
virtual bool canDropMimeData(const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const
You cannot drop mime data into the ProjectItemModel.
void onBundleSolutionInfoAdded(BundleSolutionInfo *bundleSolutionInfo)
Slot to connect to the bundleSolutionInfoAdded() signal from a project.
bool rejectName(QStringList &reserved, QString target)
Checks to see if we are adding a reserved name to the project (ex.
void itemRemoved(ProjectItem *)
This signal is emitted when a ProjectItem is removed to the model.
void insertRow(int row, ProjectItem *item)
Inserts a top-level item at the given row.
QModelIndex indexFromItem(const ProjectItem *item)
Returns the QModelIndex corresponding to a given ProjectItem.
ProjectItem * takeItem(int row)
Removes the top-level row and returns the removed item.
void onNameChanged(QString newName)
Slot to connect to the nameChanged() signal from a Project.
ProjectItem * currentItem()
Returns the current item of the internal selection model.
List of GuiCameras saved as QSharedPointers.
virtual void removeItem(ProjectItem *item)
Removes an item and its children from the model.
bool setData(const QModelIndex &index, const QVariant &value, int role)
This virtual method was added to handle changing the project name by double-clicking the project name...
This is free and unencumbered software released into the public domain.
Qt::ItemFlags flags(const QModelIndex &index) const
This virtual method was added to handle changing the project name by double-clicking the project name...
void onRowsRemoved(const QModelIndex &parent, int start, int end)
Slot to connect to the rowsAboutToBeRemoved() signal from QAbstractItemModel.
void onTargetsAdded(TargetBodyList *targets)
Slot to connect to the targetsAdded() signal from a Project.
Represents an item of a ProjectItemModel in Qt's model-view framework.