Loading [MathJax]/jax/output/NativeMML/config.js
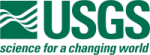 |
Isis 3 Programmer Reference
|
11 #include "ProjectItemModel.h"
13 #include <QItemSelection>
15 #include <QMessageBox>
17 #include <QModelIndex>
20 #include <QRegExpValidator>
21 #include <QStandardItemModel>
25 #include "BundleSolutionInfo.h"
27 #include "ControlList.h"
29 #include "GuiCameraList.h"
30 #include "ImageList.h"
32 #include "ProjectItem.h"
33 #include "ShapeList.h"
34 #include "TargetBodyList.h"
35 #include "TemplateList.h"
47 const QItemSelection &) ),
50 connect(
this, SIGNAL(rowsInserted(
const QModelIndex &,
int,
int)),
53 connect(
this, SIGNAL(rowsAboutToBeRemoved(
const QModelIndex &,
int,
int)),
81 Qt::DropAction action,
83 const QModelIndex &parent)
const {
109 connect(project, SIGNAL( nameChanged(QString) ),
113 connect(project, SIGNAL( controlAdded(
Control *) ),
115 connect(project, SIGNAL( controlListAdded(
ControlList *) ),
117 connect(project, SIGNAL( imagesAdded(
ImageList *) ),
119 connect(project, SIGNAL( shapesAdded(
ShapeList *) ),
123 connect(project, SIGNAL( templatesAdded(
TemplateList *)),
163 foreach ( QModelIndex index, selection.indexes() ) {
181 QModelIndexList indices = selection.indexes();
184 if (indices.size() == 0) {
186 items.append(imageRoot);
187 for (
int i = 0; i < imageRoot->rowCount(); i++) {
189 items.append(imglistItem);
190 for (
int j = 0; j < imglistItem->rowCount(); j++) {
193 items.append(imgItem);
201 foreach ( QModelIndex ix, indices ) {
209 items.append(
item );
218 if (this->hasChildren(ix)) {
222 bool childrenSelected(
false);
223 int numChildren = this->rowCount(ix);
226 for (
int i = 0; i < numChildren;i++) {
227 QModelIndex ixchild = this->index(i,0,ix);
228 if (indices.contains(ixchild) ){
229 childrenSelected=
true;
234 if (childrenSelected) {
235 for (
int i =0;i < numChildren;i++) {
236 QModelIndex ixchild = this->index(i,0,ix);
237 if (indices.contains(ixchild))
244 for (
int i =0;i < numChildren;i++) {
245 QModelIndex ixchild = this->index(i,0,ix);
258 if (!items.contains(parent)){
259 items.append(parent);
267 if (!items.contains(grandparent)) {
268 items.append(grandparent);
293 for (
int i=0; i<rowCount(); i++) {
321 if (
item->hasChildren()) {
322 for (
int row = (
item->rowCount() - 1); row >= 0; row--) {
329 removeRow(
item->row(), parentItem->index());
332 removeRow(
item->row());
355 QStandardItemModel::appendRow(
item);
367 return QStandardItemModel::indexFromItem(
item);
378 QStandardItemModel::insertRow(row,
item);
390 return static_cast<ProjectItem *
>( QStandardItemModel::item(row) );
402 return static_cast<ProjectItem *
>( QStandardItemModel::itemFromIndex(index) );
413 QStandardItemModel::setItem(row,
item);
427 if ( items.isEmpty() ) {
431 return static_cast<ProjectItem *
>( items.first() );
442 Project *project = qobject_cast<Project *>( sender() );
448 for (
int i=0; i<rowCount(); i++) {
450 if (projectItem->
project() == project) {
451 projectItem->setText(newName);
469 Project *project = qobject_cast<Project *>( sender() );
470 m_reservedNames.append(bundleSolutionInfo->
name() );
476 for (
int i=0; i<rowCount(); i++) {
478 if (projectItem->
project() == project) {
479 for (
int j=0; j < projectItem->rowCount(); j++) {
481 if (resultsItem->text() ==
"Results") {
489 QIcon(
FileName(
"$ISISROOT/appdata/images/icons/office-chart-pie.png")
495 QIcon(
FileName(
"$ISISROOT/appdata/images/icons/office-chart-pie.png")
501 QIcon(
FileName(
"$ISISROOT/appdata/images/icons/office-chart-pie.png")
507 QIcon(
FileName(
"$ISISROOT/appdata/images/icons/office-chart-pie.png")
526 Project *project = qobject_cast<Project *>( sender() );
527 if (!project) {
return; }
531 for (
int i = 0; i<rowCount(); i++) {
533 if (projectItem->
project() == project) {
536 for (
int j = 0; j < projectItem->rowCount(); j++) {
538 if (templatesItem->text() ==
"Templates"){
541 QString type = templateList->
type();
542 for (
int k = 0; k < templatesItem->rowCount(); k++) {
544 if (templateType->text().toLower() == type) {
564 Project *project = qobject_cast<Project *>( sender() );
565 m_reservedNames.append(control->
id() );
571 for (
int i=0; i<rowCount(); i++) {
573 if (projectItem->
project() == project) {
574 for (
int j=0; j < projectItem->rowCount(); j++) {
576 if (controlsItem->text() ==
"Control Networks") {
577 for (
int k=0; k < controlsItem->rowCount(); k++) {
580 if ( controlList && controlList->contains(control) ) {
603 Project *project = qobject_cast<Project *>( sender() );
604 m_reservedNames.append(controlList->
name() );
610 for (
int i=0; i<rowCount(); i++) {
612 if (projectItem->
project() == project) {
613 for (
int j=0; j < projectItem->rowCount(); j++) {
615 if (controlsItem->text() ==
"Control Networks") {
634 Project *project = qobject_cast<Project *>( sender() );
635 m_reservedNames.append(imageList->
name() );
640 for (
int i=0; i<rowCount(); i++) {
642 if (projectItem->
project() == project) {
643 for (
int j=0; j < projectItem->rowCount(); j++) {
645 if (imagesItem->text() ==
"Images") {
663 Project *project = qobject_cast<Project *>( sender() );
664 m_reservedNames.append(shapes->
name());
669 for (
int i=0; i<rowCount(); i++) {
671 if (projectItem->
project() == project) {
672 for (
int j=0; j < projectItem->rowCount(); j++) {
674 if (shapesItem->text() ==
"Shapes") {
692 Project *project = qobject_cast<Project *>( sender() );
693 m_reservedNames.append(targets->
name() );
699 for (
int i=0; i<rowCount(); i++) {
701 if (projectItem->
project() == project) {
702 for (
int j=0; j < projectItem->rowCount(); j++) {
704 if (targetsItem->text() ==
"Target Body") {
707 for (
int k=0; k < targetsItem->rowCount(); k++) {
733 Project *project = qobject_cast<Project *>( sender() );
734 m_reservedNames.append(cameras->
name() );
740 for (
int i=0; i<rowCount(); i++) {
742 if (projectItem->
project() == project) {
743 for (
int j=0; j < projectItem->rowCount(); j++) {
745 if (camerasItem->text() ==
"Sensors") {
748 for (
int k=0; k < camerasItem->rowCount(); k++) {
776 const QItemSelection &deselected) {
778 foreach ( QModelIndex index, selected.indexes() ) {
789 foreach ( QModelIndex index, deselected.indexes() ) {
813 for (
int row=start; row <= end; row++) {
814 QModelIndex newIndex = index(row, 0, parent);
831 for (
int row=start; row <= end; row++) {
832 QModelIndex newIndex = index(row, 0, parent);
858 QString name = value.toString();
860 bool rejected =
rejectName(m_reservedNames,name);
863 QMessageBox nameRejected;
864 nameRejected.setText(
"That name is already in use within this project.");
869 m_reservedNames.append(name);
895 else if (
item->isTemplate() && role == Qt::EditRole) {
916 return Qt::ItemIsEditable | QStandardItemModel::flags(index);
932 QRegExpValidator valid;
933 QValidator::State state;
935 foreach (QString name, reserved) {
939 state = valid.validate(target,pos);
953 for (
int i=0; i<rowCount(); i++) {
956 for (
int j=0; j < projectItem->rowCount(); j++) {
957 if (projectItem->hasChildren()) {
961 if (subProjectItem->text() ==
"Templates") {
962 if (subProjectItem->hasChildren()) {
963 for (
int k=0; k < subProjectItem->rowCount(); k++) {
965 while (tempProjectItem->hasChildren()) {
972 while (subProjectItem->hasChildren()) {
BundleSolutionInfo * bundleSolutionInfo() const
Returns the BundleSolutionInfo stored in the data of the item.
void setSelected(bool)
Change the selected state associated with this cube.
QString savedPointsFilename()
Returns filename of output bundle points csv file.
void setItem(int row, ProjectItem *item)
Sets the item at the top-level row.
void onRowsInserted(const QModelIndex &parent, int start, int end)
Slot to connect to the rowsInserted() signal from QAbstractItemModel.
void onControlListAdded(ControlList *controlList)
Slot to connect to the controlListAdded() signal from a Project.
QString savedResidualsFilename()
Returns filename of output bundle residuals csv file.
void setName(QString newName)
Set the human-readable name of this control list.
This is free and unencumbered software released into the public domain.
void onSelectionChanged(const QItemSelection &selected, const QItemSelection &deselected)
Slot to connect to the selectionChanged() signal from a selection model.
void projectNameEdited(QString)
This signal is emitted when the project name is edited.
Image * image() const
Returns the Image stored in the data of the item.
void appendRow(ProjectItem *item)
Appends a top-level item to the model.
void onGuiCamerasAdded(GuiCameraList *cameras)
Slot to connect to the guiCamerasAdded() signal from a Project.
QList< ProjectItem * > selectedBOSSImages()
ProjectItemModel::selectedBOSSImages.
File name manipulation and expansion.
Maintains a list of Controls so that control nets can easily be copied from one Project to another,...
QString name() const
Returns the name of the bundle.
void setName(QString newName)
Set the human-readable name of this shape list.
virtual void removeItems(QList< ProjectItem * > items)
Removes a list of items and their children from the model.
QItemSelectionModel * selectionModel()
Returns the internal selection model.
ProjectItemModel(QObject *parent=0)
Constructs an empty model.
void clean()
Used to clean the ProjectItemModel of everything but the headers.
ProjectItem * child(int row) const
Returns the child item at a given row.
void setName(QString name)
Sets the name of the bundle.
bool isControlList() const
Returns true if a ControlList is stored in the data of the item.
QString savedBundleOutputFilename()
Returns bundleout text filename.
This represents an ISIS control net in a project-based GUI interface.
QString savedImagesFilename()
Returns filename of output bundle images csv file.
ProjectItem * item(int row)
Returns the top-level item at the given row.
ProjectItem * itemFromIndex(const QModelIndex &index)
Returns the ProjectItem corresponding to a given QModelIndex.
void itemAdded(ProjectItem *)
This signal is emitted when a ProjectItem is added to the model.
The main project for ipce.
void onControlAdded(Control *control)
Slot to connect to the controlAdded() signal from a project.
~ProjectItemModel()
Destructs the model.
Internalizes a list of images and allows for operations on the entire list.
void setName(QString newName)
Set the human-readable name of this image list.
bool isImageList() const
Returns true if an ImageList is stored in the data of the item.
void onTemplatesAdded(TemplateList *templateList)
Slot connected to the templatesAdded() signal from a project.
bool isShapeList() const
Returns true if an ShapeList is stored in the data of the item.
GuiCameraQsp guiCamera() const
Returns the GuiCameraQsp stored in the data of the item.
bool isImage() const
Returns true if an Image is stored in the data of the item.
void onImagesAdded(ImageList *images)
Slot to connect to the imagesAdded() signal from a Project.
QList< ProjectItem * > selectedItems()
Returns a list of the selected items of the internal selection model.
QItemSelectionModel * m_selectionModel
The internal selection model.
void appendRow(ProjectItem *item)
Appends an item to the children of this item.
ShapeList * shapeList() const
Returns the ShapeList stored in the data of the item.
void cleanProject(bool)
This signal is emitted whrn a ProjectItem's name is changed.
Container class for BundleAdjustment results.
void onShapesAdded(ShapeList *shapes)
Slot to connect to the shapesAdded() signal from a Project.
QString name() const
Get the human-readable name of this image list.
ProjectItem * addProject(Project *project)
Adds a Project to the model.
Internalizes a list of shapes and allows for operations on the entire list.
List for holding TargetBodies.
ProjectItem * findItemData(const QVariant &data, int role=Qt::UserRole+1)
Returns the first item found that contains the given data in the given role or a null pointer if no i...
virtual bool canDropMimeData(const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const
You cannot drop mime data into the ProjectItemModel.
ControlList * controlList() const
Returns the ControlList stored in the data of the item.
void onBundleSolutionInfoAdded(BundleSolutionInfo *bundleSolutionInfo)
Slot to connect to the bundleSolutionInfoAdded() signal from a project.
bool isProject() const
Returns true if a Project is stored in the data of the item.
bool rejectName(QStringList &reserved, QString target)
Checks to see if we are adding a reserved name to the project (ex.
void itemRemoved(ProjectItem *)
This signal is emitted when a ProjectItem is removed to the model.
ImageDisplayProperties * displayProperties()
Get the display (GUI) properties (information) associated with this image.
void insertRow(int row, ProjectItem *item)
Inserts a top-level item at the given row.
QString name() const
Get the human-readable name of this target body list.
QModelIndex indexFromItem(const ProjectItem *item)
Returns the QModelIndex corresponding to a given ProjectItem.
QString name() const
Get the human-readable name of this shape list.
QString type() const
Get the type of template in this TemplateList.
Project * project() const
Returns the Project stored in the data of the item.
ProjectItem * takeItem(int row)
Removes the top-level row and returns the removed item.
ProjectItem * findItemData(const QVariant &value, int role=Qt::UserRole+1)
Finds and returns the first item in the model that contains the data in the role.
QSharedPointer< FileItem > FileItemQsp
A FileItem smart pointer.
ProjectItem * parent() const
Returns the parent item of this item.
ImageList * imageList() const
Returns the ImageList stored in the data of the item.
QString name() const
Get the human-readable name of this control list.
QString name() const
Get the human-readable name of this gui cameray list.
void onNameChanged(QString newName)
Slot to connect to the nameChanged() signal from a Project.
ProjectItem * currentItem()
Returns the current item of the internal selection model.
List of GuiCameras saved as QSharedPointers.
A container for a filename to be represented as a ProjectItem on the project tree.
void setClean(bool value)
Function to change the clean state of the project.
QString id() const
Access the unique ID associated with this Control.
virtual void removeItem(ProjectItem *item)
Removes an item and its children from the model.
bool isBundleSolutionInfo() const
Returns true if a BundleSolutionInfo is stored in the data of the item.
bool setData(const QModelIndex &index, const QVariant &value, int role)
This virtual method was added to handle changing the project name by double-clicking the project name...
This is free and unencumbered software released into the public domain.
Qt::ItemFlags flags(const QModelIndex &index) const
This virtual method was added to handle changing the project name by double-clicking the project name...
void onRowsRemoved(const QModelIndex &parent, int start, int end)
Slot to connect to the rowsAboutToBeRemoved() signal from QAbstractItemModel.
void onTargetsAdded(TargetBodyList *targets)
Slot to connect to the targetsAdded() signal from a Project.
TargetBodyQsp targetBody() const
Returns the TargetBodyQsp stored in the data of the item.
Represents an item of a ProjectItemModel in Qt's model-view framework.