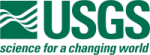 |
Isis 3 Programmer Reference
|
2 #include "ScatterPlotData.h"
9 #include "SpecialPixel.h"
27 Cube *xCube,
int xCubeBand,
int xBinCount,
28 Cube *yCube,
int yCubeBand,
int yBinCount,
29 QwtInterval sampleRange, QwtInterval lineRange) :
QwtRasterData(),
33 m_alarmedBins(new
QMap<int, bool>) {
34 int startLine = qRound(lineRange.minValue());
35 int endLine = qRound(lineRange.maxValue());
39 sampleRange.minValue(), lineRange.minValue(),
40 sampleRange.maxValue(), lineRange.maxValue(),
47 sampleRange.minValue(), lineRange.minValue(),
48 sampleRange.maxValue(), lineRange.maxValue(),
62 Brick brick1((
int)(sampleRange.maxValue() - sampleRange.minValue() + 1),
64 Brick brick2((
int)(sampleRange.maxValue() - sampleRange.minValue() + 1),
67 ASSERT(brick1.
size() == brick2.
size());
69 for (
int line = startLine; line <= endLine; line++) {
70 brick1.
SetBasePosition(qRound(sampleRange.minValue()), line, xCubeBand);
73 brick2.
SetBasePosition(qRound(sampleRange.minValue()), line, yCubeBand);
76 for (
int i = 0; i < brick1.
size(); i++) {
77 double xDn = brick1[i];
78 double yDn = brick2[i];
85 int roundedX = qRound(x);
86 int roundedY = qRound(y);
88 if (roundedX >= 0 && roundedX < xBinCount &&
89 roundedY >= 0 && roundedY < yBinCount) {
90 int value = (*m_counts)[roundedY][roundedX] + 1;
91 (*m_counts)[roundedY][roundedX] =
value;
102 setInterval(Qt::ZAxis, QwtInterval(0,
m_maxCount));
156 int index =
binIndex(indices.first, indices.second);
234 int xIndex = indices.first;
235 int yIndex = indices.second;
237 if (xIndex != -1 && yIndex != -1) {
243 xSize = (*m_counts)[0].size();
245 double percentAcrossXRange = ((double)xIndex / (
double)xSize);
249 double percentAcrossYRange = ((double)yIndex / (
double)ySize);
257 QString msg =
"Bin at index [" + QString::number(index) +
"] not found. "
258 "There are [" + QString::number(
numberOfBins()) +
"] bins";
271 return binCount(indices.first, indices.second);
286 xSize = (*m_counts)[0].size();
288 return xSize * ySize;
302 int xSize = (*m_counts)[0].size();
303 xValues.resize(xSize);
305 for (
int xIndex = 0; xIndex < xSize; xIndex++) {
306 double percentAcrossXRange = ((double)xIndex / (
double)xSize);
325 if (binToAlarm != -1)
326 (*m_alarmedBins)[binToAlarm] =
true;
384 if (yIndex >= 0 && yIndex < m_counts->size()) {
385 if (xIndex >= 0 && xIndex < (*
m_counts)[yIndex].size()) {
386 count = (*m_counts)[yIndex][xIndex];
408 xSize = (*m_counts)[0].size();
411 if ((xIndex >= 0 && xIndex < xSize) && (yIndex >= 0 && yIndex < ySize))
412 index = xSize * yIndex + xIndex;
427 return binIndex(indices.first, indices.second);
443 xSize = (*m_counts)[0].size();
450 if (xIndex < 0 || yIndex < 0 || xIndex >= xSize || yIndex >= ySize) {
451 QString msg =
"Bin at index [" + QString::number(
binIndex) +
"] not found. "
452 "There are [" + QString::number(
numberOfBins()) +
"] bins";
475 int discreteXBin = qRound(xBinPosition);
476 int discreteYBin = qRound(yBinPosition);
478 if (discreteXBin >= 0 && discreteXBin < m_counts->at(0).size() &&
479 discreteYBin >= 0 && discreteYBin < m_counts->size()) {
void SetBasePosition(const int start_sample, const int start_line, const int start_band)
This method is used to set the base position of the shape buffer.
QScopedPointer< QMap< int, bool > > m_alarmedBins
map from bin index to alarm state (true for alarmed)
int binIndex(int xIndex, int yIndex) const
Get the single-index position given an x/y index position.
double m_xCubeMax
The maximum DN value for the x cube.
QScopedPointer< Stretch > m_yDnToBinStretch
Stretch 2.
double m_yCubeMin
The minimum DN value for the y cube.
void read(Blob &blob, const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
This method will read data from the specified Blob object.
int m_maxCount
The maximum value in m_counts, stored for efficiency.
double Maximum() const
Returns the absolute maximum double found in all data passed through the AddData method.
double m_yCubeMax
The maximum DN value for the y cube.
QScopedPointer< Stretch > m_xDnToBinStretch
Stretch 1.
QScopedPointer< QVector< QVector< int > > > m_counts
The bin counts stored by 2D (x/y) index position.
double xCubeMax() const
Return the max DN value for the y-axis cube's data range.
void clearAlarms()
Forget all alarmed bins (viewport->plot).
Buffer for containing a three dimensional section of an image.
bool IsSpecial(const double d)
Returns if the input pixel is special.
ScatterPlotData(Cube *xCube, int xCubeBand, int xBinCount, Cube *yCube, int yCubeBand, int yBinCount, QwtInterval sampleRange, QwtInterval lineRange)
ScatterPlotDataConstructor.
void swap(ScatterPlotData &other)
This is part of the copy-and-swap paradigm.
ScatterPlotData & operator=(const ScatterPlotData &other)
Take the data from other and copy it into this.
QPair< double, double > binXY(int binIndex) const
Get the center X/Y Dn values for the bin at index.
virtual QwtRasterData * copy() const
Returns a copy of the ScatterPlotData object.
int numberOfBins() const
Get the total number of bins (bin count in x * bin count in y).
QRectF pixelHint(const QRectF &area) const
This is a hint given to qwt for how to render a pixel in the spectrogram.
double Minimum() const
Returns the absolute minimum double found in all data passed through the AddData method.
double yCubeMin() const
Return the min DN value for the y-axis cube's data range.
This is the QwtRasterData for a scatter plot.
Container of a cube histogram.
IO Handler for Isis Cubes.
double xCubeMin() const
Return the min DN value for the x-axis cube's data range.
QVector< double > discreteXValues() const
Get a list of all of the x-bin center values for this scatter plot.
virtual double value(double x, double y) const
This gets called every time the scatter plot is re-drawn.
double m_xCubeMin
The minimum DN value for the x cube.
double yCubeMax() const
Return the max DN value for the y-axis cube's data range.
@ Programmer
This error is for when a programmer made an API call that was illegal.
PixelType pixelType() const
int binCount(int binIndex) const
Get the count (number of values) which fall into the bin at index.
This is free and unencumbered software released into the public domain.
~ScatterPlotData()
Destructor.
This is free and unencumbered software released into the public domain.
int size() const
Returns the total number of pixels in the shape buffer.
QPair< int, int > binXYIndices(int binIndex) const
Get the 2D index index position given a 1D (flat) index position.
This is free and unencumbered software released into the public domain.
This is free and unencumbered software released into the public domain.
void alarm(double x, double y)
Alarm the bin (highlight it) at the given x/y DN value.