Loading [MathJax]/jax/output/NativeMML/config.js
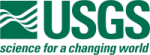 |
Isis 3 Programmer Reference
|
9 #include "AtmosModel.h"
10 #include "NumericalApproximation.h"
11 #include "IException.h"
14 ShadeAtm::ShadeAtm(Pvl &pvl, PhotoModel &pmodel, AtmosModel &amodel) : NormModel(pvl, pmodel, amodel) {
15 PvlGroup &algorithm = pvl.findObject(
"NormalizationModel").findGroup(
"Algorithm",
Pvl::Traverse);
22 if(algorithm.hasKeyword(
"Incref")) {
26 if(algorithm.hasKeyword(
"Pharef")) {
29 p_normPharef = p_normIncref;
32 if(algorithm.hasKeyword(
"Emaref")) {
36 if(algorithm.hasKeyword(
"Albedo")) {
55 void ShadeAtm::NormModelAlgorithm(
double phase,
double incidence,
double emission,
56 double demincidence,
double dememission,
double dn,
57 double &albedo,
double &mult,
double &base) {
61 static double ahInterp;
69 static double old_phase = -9999;
70 static double old_incidence = -9999;
71 static double old_emission = -9999;
72 static double old_demincidence = -9999;
73 static double old_dememission = -9999;
77 psurfref = GetPhotoModel()->
CalcSurfAlbedo(p_normPharef, p_normIncref, p_normEmaref);
84 std::string msg =
"Divide by zero error";
88 rho = p_normAlbedo / psurfref;
90 if (old_phase != phase || old_incidence != incidence || old_emission != emission ||
91 old_demincidence != demincidence || old_dememission != dememission) {
93 psurf = GetPhotoModel()->
CalcSurfAlbedo(phase, demincidence, dememission);
97 munot = cos(incidence * (
PI / 180.0));
100 old_incidence = incidence;
101 old_emission = emission;
102 old_demincidence = demincidence;
103 old_dememission = dememission;
106 GetAtmosModel()->
CalcAtmEffect(phase, incidence, emission, &pstd, &trans, &trans0, &sbar,
109 albedo = pstd + rho * (ahInterp * munot * trans /
110 (1.0 - rho * GetAtmosModel()->
AtmosAb() * sbar) + (psurf - ahInterp * munot) * trans0);
123 if(pharef < 0.0 || pharef >= 180.0) {
124 std::string msg =
"Invalid value of normalization pharef [" +
129 p_normPharef = pharef;
142 if(incref < 0.0 || incref >= 90.0) {
143 std::string msg =
"Invalid value of normalization incref [" +
148 p_normIncref = incref;
161 if(emaref < 0.0 || emaref >= 90.0) {
162 std::string msg =
"Invalid value of normalization emaref [" +
167 p_normEmaref = emaref;
179 p_normAlbedo = albedo;
void SetNormIncref(const double incref)
Set the normalization function parameter.
@ Extrapolate
Evaluate() attempts to extrapolate if a is outside of the domain. This is only valid for NumericalApp...
const double PI
The mathematical constant PI.
@ Unknown
A type of error that cannot be classified as any of the other error types.
Isotropic atmos scattering model.
double CalcSurfAlbedo(double pha, double inc, double ema)
Calculate the surface brightness using photometric angle information.
Container for cube-like labels.
void SetNormEmaref(const double emaref)
Set the normalization function parameter.
void SetNormAlbedo(const double albedo)
Set the normalization function parameter.
@ Traverse
Search child objects.
void SetNormIncref(const double incref)
Set the normalization function parameter.
void GenerateAhTable()
This method computes the values of the atmospheric Ah table and sets the properties of the atmospheri...
void SetNormPharef(const double pharef)
Set the normalization function parameter.
NumericalApproximation AtmosAhSpline()
If GenerateAhTable() has been called this returns a clamped cubic spline of the data set (p_atmosIncT...
virtual void SetStandardConditions(bool standard)
Sets whether standard conditions will be used.
double AtmosAb() const
Return atmospheric Ab value.
void SetNormPharef(const double pharef)
Set parameters needed for albedo normalization.
Adds specific functionality to C++ strings.
void CalcAtmEffect(double pha, double inc, double ema, double *pstd, double *trans, double *trans0, double *sbar, double *transs)
Calculate the atmospheric scattering effect using photometric angle information.
void SetNormAlbedo(const double albedo)
Set the normalization function parameter.
void SetNormEmaref(const double emaref)
Set the normalization function parameter.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....