Loading [MathJax]/jax/output/NativeMML/config.js
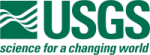 |
Isis 3 Programmer Reference
|
14 #include <QSharedPointer>
15 #include "SmtkStack.h"
17 #include "SmtkPoint.h"
19 #include "GSLUtility.h"
20 #include <gsl/gsl_rng.h>
62 return (m_gruen->PatternChip());
68 return (m_gruen->SearchChip());
74 return (m_gruen->FitChip());
81 const double &minEV,
const double &maxEV);
95 inline BigInt OffImageErrorCount()
const {
return (m_offImage); }
96 inline BigInt SpiceErrorCount()
const {
return (m_spiceErr); }
113 const gsl_rng_type * T;
117 bool validate(
const bool &throwError =
true)
const;
119 inline Camera &lhCamera() {
return (*m_lhCube->
camera()); }
120 inline Camera &rhCamera() {
return (*m_rhCube->
camera()); }
122 Coordinate
getLineSample(Camera &camera,
const Coordinate &geom);
123 Coordinate
getLatLon(Camera &camera,
const Coordinate &pnt);
125 bool inCube(
const Camera &camera,
const Coordinate &point)
const;
128 const PointGeometry &right, Gruen *gruen);
SmtkQStackIter FindSmallestEV(SmtkQStack &stack)
Find the smallest eigen value on the given stack.
void setGruenDef(const QString ®def)
Initialize Gruen algorithm with definitions in Pvl file provided.
SmtkPoint makeRegisteredPoint(const PointGeometry &left, const PointGeometry &right, Gruen *gruen)
Create an SmtkPoint from Gruen match result.
Container for SMTK match points.
Coordinate getLatLon(Camera &camera, const Coordinate &pnt)
Compute latitude, longitude from line,sample.
Chip * PatternChip() const
Return pattern chip.
Container for affine and radiometric parameters.
Container for cube-like labels.
void randomNumberSetup()
Initialize the random number generator.
Define a generic Y/X container.
Pvl RegistrationStatistics()
Return Gruen registration statistics.
SmtkPoint Clone(const SmtkPoint &point, const Coordinate &left)
Clone a point set from a nearby (left image) point and Gruen affine.
SmtkQStackIter FindExpDistEV(SmtkQStack &stack, const double &seedsample, const double &minEV, const double &maxEV)
Find the best eigen value using exponential distribution formula.
bool validate(const bool &throwError=true) const
Validates the state of the Camera and Gruen algoritm.
void setImages(Cube *lhImage, Cube *rhImage)
Assign cubes for matching.
SmtkMatcher()
Construct default matcher.
This is free and unencumbered software released into the public domain.
void setWriteSubsearchChipPattern(const QString &fileptrn="SmtkMatcher")
Set file pattern for output subsearch chips.
Contains multiple PvlContainers.
Define a point set of left, right and geometry at that location.
PvlGroup RegTemplate()
Return Gruen template parameters.
long long int BigInt
Big int.
~SmtkMatcher()
Free random number generator in destructor.
Coordinate getLineSample(Camera &camera, const Coordinate &geom)
Compute line,sample from latitude, longitude.
IO Handler for Isis Cubes.
bool isValid(const Coordinate &pnt)
Determine if a point is valid in both left/right images.
bool inCube(const Camera &camera, const Coordinate &point) const
Determines if the line/sample is within physical cube boundaries.
Camera * camera()
Return a camera associated with the cube.
A small chip of data used for pattern matching.
Container for a point and its geometry.
SmtkPoint Create(const Coordinate &left, const Coordinate &right)
Create a valid, unregistered SmtkPoint.
Workhorse of stereo matcher.
Chip * FitChip() const
Returns the fit chip.
SmtkPoint Register(const Coordinate &lpnt, const AffineRadio &affrad=AffineRadio())
This method takes a sample, line from the left-hand image and tries to find the matching point in the...
This is free and unencumbered software released into the public domain.
Chip * SearchChip() const
Return search chip.