File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
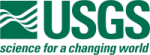 |
Isis 3 Programmer Reference
|
13 #include "IException.h"
14 #include "PolygonTools.h"
16 #include "StripPolygonSeeder.h"
54 std::vector<geos::geom::Point *> points;
57 const geos::geom::Envelope *polyBoundBox = multiPoly->getEnvelopeInternal();
70 geos::geom::Point *centroid = multiPoly->getCentroid();
71 double centerX = centroid->getX();
72 double centerY = centroid->getY();
75 int xStepsToCentroid = (int)((centerX - polyBoundBox->getMinX()) /
p_Xspacing + 0.5);
76 int yStepsToCentroid = (int)((centerY - polyBoundBox->getMinY()) /
p_Yspacing + 0.5);
77 double dRealMinX = centerX - (xStepsToCentroid *
p_Xspacing);
78 double dRealMinY = centerY - (yStepsToCentroid *
p_Yspacing);
82 for(
double y = dRealMinY; y <= polyBoundBox->getMaxY(); y +=
p_Yspacing) {
84 for(
double x = dRealMinX; x <= polyBoundBox->getMaxX(); x +=
p_Xspacing) {
85 geos::geom::Coordinate c(x + dDeltaXToReal, y + dDeltaYToReal);
86 geos::geom::Point *p = Isis::globalFactory->createPoint(c);
87 if(p->within(multiPoly)) {
88 points.push_back(Isis::globalFactory->createPoint(c));
91 geos::geom::Coordinate c2(x - dDeltaXToReal, y - dDeltaYToReal);
92 p = Isis::globalFactory->createPoint(c2);
93 if(p->within(multiPoly)) {
94 points.push_back(Isis::globalFactory->createPoint(c2));
128 QString msg =
"PVL for StripSeeder must contain [XSpacing] in [";
141 QString msg =
"PVL for StripSeeder must contain [YSpacing] in [";
147 QString msg =
"Improper format for PolygonSeeder PVL [" + pvl.
fileName() +
"]";
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
A single keyword-value pair.
double p_Yspacing
The spacing in the y direction between points.
void addKeyword(const PvlKeyword &keyword, const InsertMode mode=Append)
Add a keyword to the container.
virtual void Parse(Pvl &pvl)
Parse the StripSeeder spicific parameters from the PVL.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
virtual PvlGroup PluginParameters(QString grpName)
Plugin parameters.
double MinimumArea()
Return the minimum allowed area of the polygon.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Seed points using a grid with a staggered pattern.
@ Traverse
Search child objects.
double MinimumThickness()
Return the minimum allowed thickness of the polygon.
Contains multiple PvlContainers.
Pvl * invalidInput
The Pvl passed in by the constructor minus what was used.
QString fileName() const
Returns the filename used to initialise the Pvl object.
StripPolygonSeeder(Pvl &pvl)
Construct a StripPolygonSeeder algorithm.
virtual void Parse(Pvl &pvl)
Initialize parameters in the PolygonSeeder class using a PVL specification.
void deleteKeyword(const QString &name)
Remove a specified keyword.
std::vector< geos::geom::Point * > Seed(const geos::geom::MultiPolygon *mp)
Seed a polygon with points.
QString Algorithm() const
The name of the algorithm, read from the Name Keyword in the PolygonSeeder Pvl passed into the constr...
double p_Xspacing
The spacing in the x direction between points.
QString StandardTests(const geos::geom::MultiPolygon *multiPoly, const geos::geom::Envelope *polyBoundBox)
Check the polygon to see if it meets standard criteria.
Adds specific functionality to C++ strings.
This class is used as the base class for all PolygonSeeder objects.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....