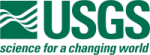 |
Isis 3 Programmer Reference
|
1 #ifndef _CONTROLNETSTATISTICS_H_
2 #define _CONTROLNETSTATISTICS_H_
17 #include "SerialNumberList.h"
18 #include "Statistics.h"
75 enum ePointDetails { total, ignore, locked, fixed, constrained, freed };
76 static const int numPointDetails = 6;
79 enum ePointIntStats { totalPoints, validPoints, ignoredPoints, fixedPoints, constrainedPoints, freePoints, editLockedPoints,
80 totalMeasures, validMeasures, ignoredMeasures, editLockedMeasures };
81 static const int numPointIntStats = 11;
84 enum ePointDoubleStats { avgResidual, minResidual, maxResidual, minLineResidual, maxLineResidual, minSampleResidual, maxSampleResidual,
85 avgPixelShift, minPixelShift, maxPixelShift, minLineShift, maxLineShift, minSampleShift, maxSampleShift,
86 minGFit, maxGFit, minEccentricity, maxEccentricity, minPixelZScore, maxPixelZScore};
87 static const int numPointDblStats = 20;
90 enum ImageStats { imgSamples, imgLines, imgTotalPoints, imgIgnoredPoints, imgFixedPoints, imgLockedPoints, imgLocked,
91 imgConstrainedPoints, imgFreePoints, imgConvexHullArea, imgConvexHullRatio };
92 static const int numImageStats = 11;
246 void UpdateMinMaxStats(
const Statistics & stats,
This class is used to accumulate statistics on double arrays.
void GenerateImageStats()
Generate stats like Total, Ignored, Fixed Points in an Image.
double GetMaxPixelShift() const
Get network Max PixelShift.
double GetMaxLineShift() const
Get network Max LineShift.
ePointDoubleStats
Enumeration for Point stats like Tolerances, PixelShifts which have double data.
void GetPointIntStats()
Get point count stats.
double GetMinLineResidual() const
Determine the minimum line error of all points in the network.
ImageStats
Enumeration for image stats.
int NumValidPoints() const
Returns the Number of Valid (Not Ignored) Points in the Control Net.
double GetAvgPixelShift() const
Get network Avg PixelShift.
double GetMaxSampleResidual() const
Determine the maximum sample error of all points in the network.
QMap< int, double > mPointDoubleStats
Contains QMap of different computed stats.
Statistics mConvexHullRatioStats
min, max, average convex hull stats
QMap< int, int > mPointIntStats
Contains QMap of different count stats.
int NumIgnoredMeasures() const
Returns the total Number of Ignored Measures in the Control Net.
double GetMaximumResidual() const
Determine the maximum error of all points in the network.
QVector< double > GetImageStatsBySerialNum(QString psSerialNum) const
Returns the Image Stats by Serial Number.
double GetMinSampleResidual() const
Determine the minimum sample error of all points in the network.
ControlNetStatistics(ControlNet *pCNet, const QString &psSerialNumFile, Progress *pProgress=0)
Constructor.
Serial Number list generator.
void GetPointDoubleStats()
Get Point stats for Residuals and Shifts.
~ControlNetStatistics()
Destructor.
int NumConstrainedPoints() const
Returns the number of Constrained Points in Control Net.
int NumEditLockedPoints() const
Returns total number of edit locked points.
int NumFixedPoints() const
Returns the Number of Fixed Points in the Control Net.
QMap< QString, QVector< double > > mImageMap
Contains stats by Image/Serial Num.
double GetMaxLineResidual() const
Determine the maximum line error of all points in the network.
int NumIgnoredPoints() const
Returns the number of ignored points.
double GetMaxSampleShift() const
Get network Max SampleShift.
void InitPointDoubleStats()
Init Pointstats std::vector.
double GetMinPixelShift() const
Get network Min PixelShift.
int NumValidMeasures() const
Returns the total Number of valid Measures in the Control Net.
Contains multiple PvlContainers.
void PrintImageStats(const QString &psImageFile)
Print the Image Stats into specified output file.
double GetAverageResidual() const
Determine the average error of all points in the network.
ePointIntStats
Enumeration for Point int stats for counts such as valid points, measures etc.
QMap< QString, bool > mSerialNumMap
Whether serial# is part of ControlNet.
double GetMinSampleShift() const
Get network Min SampleShift.
void GenerateControlNetStats(PvlGroup &pStatsGrp)
Generate the Control Net Stats into the PvlGroup.
int NumEditLockedMeasures() const
Returns total number of edit locked measures in the network.
void InitSerialNumMap()
Init SerialNum std::map.
double GetMinimumResidual() const
Determine the minimum error of all points in the network.
Program progress reporter.
void GeneratePointStats(const QString &psPointFile)
Generate stats like Ignored, Fixed, Total Measures, Ignored by Control Point.
int NumMeasures() const
Returns the total Number of Measures in the Control Net.
ePointDetails
Enumeration for Point Statistics.
SerialNumberList mSerialNumList
Serial Number List.
double GetMinLineShift() const
Get Min and Max LineShift.
int NumFreePoints() const
Returns the number of Constrained Points in Control Net.
This is free and unencumbered software released into the public domain.
Progress * mProgress
Progress state.
ControlNet * mCNet
Control Network.