File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
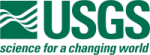 |
Isis 3 Programmer Reference
|
9 #include "ControlNetStatistics.h"
14 #include <geos/algorithm/ConvexHull.h>
15 #include <geos/geom/CoordinateSequence.h>
16 #include <geos/geom/CoordinateArraySequence.h>
17 #include <geos/geom/Envelope.h>
18 #include <geos/geom/Geometry.h>
19 #include <geos/geom/GeometryFactory.h>
20 #include <geos/geom/Polygon.h>
22 #include "ControlNet.h"
23 #include "ControlPoint.h"
24 #include "ControlMeasure.h"
25 #include "ControlMeasureLogData.h"
27 #include "CubeManager.h"
32 #include "SpecialPixel.h"
33 #include "Statistics.h"
38 QString
sPointType [] = {
"Fixed",
"Constrained",
"Free" };
100 for (
int i=0; i<numSn; i++) {
117 pStatsGrp =
PvlGroup(
"ControlNetSummary");
221 geos::geom::GeometryFactory::Ptr geosFactory = geos::geom::GeometryFactory::create();
234 foreach (QString sn, cnetSerials) {
235 geos::geom::CoordinateSequence * ptCoordinates =
236 new geos::geom::CoordinateArraySequence();
249 double cubeArea = imgStats[imgSamples] * imgStats[imgLines];
254 if (!measures.isEmpty()) {
257 imgStats[imgTotalPoints]++;
258 if (parentPoint->IsIgnored()) {
259 imgStats[imgIgnoredPoints]++;
262 imgStats[imgFixedPoints]++;
265 imgStats[imgConstrainedPoints]++;
268 imgStats[imgFreePoints]++;
270 if (parentPoint->IsEditLocked()) {
271 imgStats[imgLockedPoints]++;
274 imgStats[imgLocked]++;
276 ptCoordinates->add(geos::geom::Coordinate(measure->GetSample(),
277 measure->GetLine()));
280 ptCoordinates->add(geos::geom::Coordinate(measures[0]->GetSample(),
281 measures[0]->GetLine()));
284 if (ptCoordinates->size() >= 4) {
290 geos::geom::Geometry * convexHull = geosFactory->createPolygon(
291 geosFactory->createLinearRing(ptCoordinates), 0)->convexHull();
294 imgStats[imgConvexHullArea] = convexHull->getArea();
295 imgStats[imgConvexHullRatio] = imgStats[imgConvexHullArea] / cubeArea;
299 mConvexHullStats.
AddData(imgStats[imgConvexHullArea]);
304 delete ptCoordinates;
305 ptCoordinates = NULL;
326 QString msg =
"Serial Number of Images has not been provided to get Image Stats";
332 QString outName(outFile.
expanded());
333 ostm.open(outName.toLatin1().data(), std::ios::out);
336 QString msg = QObject::tr(
"Cannot open file [%1]").arg(psImageFile);
344 ostm <<
"Filename, SerialNumber, TotalPoints, PointsIgnored, PointsEditLocked, Fixed, Constrained, Free, ConvexHullRatio" << endl;
349 bool serialNumExists = it.value();
350 if (serialNumExists) {
352 ostm << imgStats[imgTotalPoints]<<
", " << imgStats[imgIgnoredPoints] <<
", " ;
353 ostm << imgStats[imgLockedPoints] <<
", " << imgStats[imgFixedPoints] <<
", " ;
354 ostm << imgStats[imgConstrainedPoints] <<
", " << imgStats[imgFreePoints] <<
", ";
355 ostm << imgStats[imgConvexHullRatio] << endl;
358 ostm <<
"0, 0, 0, 0, 0, 0, 0" << endl;
363 QString msg = QObject::tr(
"Error writing to file: [%1]").arg(psImageFile);
380 return (
mImageMap.find(psSerialNum)).value();
397 QString outName(outFile.
expanded());
398 ostm.open(outName.toLatin1().data(), std::ios::out);
401 QString msg = QObject::tr(
"Cannot open file [%1]").arg(psPointFile);
405 ostm <<
" PointId, PointType, PointIgnore, PointEditLock, TotalMeasures, MeasuresValid, MeasuresIgnore, MeasuresEditLock" << endl;
410 if (
mProgress != NULL && iNumPoints > 0) {
416 for (
int i = 0; i < iNumPoints; i++) {
418 int iNumMeasures = cPoint->GetNumMeasures();
420 int iIgnoredMeasures = iNumMeasures - iValidMeasures;
424 ostm <<
sBoolean[(int)cPoint->IsEditLocked()] <<
", " << iNumMeasures <<
", " << iValidMeasures <<
", ";
433 QString msg = QObject::tr(
"Error writing to file: [%1]").arg(psPointFile);
450 for (
int i=0; i<numPointIntStats; i++) {
459 for (
int i = 0; i < iNumPoints; i++) {
460 if (!
mCNet->GetPoint(i)->IsIgnored()) {
482 if (
mCNet->GetPoint(i)->IsEditLocked()) {
506 for (
int i = 0; i < numPointDblStats; i++) {
527 for (
int i = 0; i < iNumPoints; i++) {
530 if (!cp->IsIgnored()) {
531 for (
int cmIndex = 0; cmIndex < cp->GetNumMeasures(); cmIndex++) {
534 if (!cm->IsIgnored()) {
538 pixelShiftStats.
AddData(fabs(cm->GetPixelShift()));
545 UpdateMinMaxStats(resMagStats, minResidual, maxResidual);
548 &ControlMeasure::GetLineResidual);
549 UpdateMinMaxStats(resLineStats, minLineResidual, maxLineResidual);
552 &ControlMeasure::GetSampleResidual);
553 UpdateMinMaxStats(resSampStats, minSampleResidual, maxSampleResidual);
556 &ControlMeasure::GetPixelShift);
557 UpdateMinMaxStats(pixShiftStats, minPixelShift, maxPixelShift);
560 &ControlMeasure::GetLineShift);
561 UpdateMinMaxStats(lineShiftStats, minLineShift, maxLineShift);
564 &ControlMeasure::GetSampleShift);
565 UpdateMinMaxStats(sampShiftStats, minSampleShift, maxSampleShift);
569 UpdateMinMaxStats(gFitStats, minGFit, maxGFit);
575 dValue = fabs(minPixelZScoreStats.
Minimum());
584 dValue = fabs(maxPixelZScoreStats.
Maximum());
598 void ControlNetStatistics::UpdateMinMaxStats(
const Statistics & stats,
599 ePointDoubleStats min, ePointDoubleStats max) {
double GetResidualMagnitude() const
Return Residual magnitude.
This class is used to accumulate statistics on double arrays.
@ Io
A type of error that occurred when performing an actual I/O operation.
void AddData(const double *data, const unsigned int count)
Add an array of doubles to the accumulators and counters.
void GenerateImageStats()
Generate stats like Total, Ignored, Fixed Points in an Image.
double GetMaxPixelShift() const
Get network Max PixelShift.
void CheckStatus()
Checks and updates the status.
double GetMaxLineShift() const
Get network Max LineShift.
A single keyword-value pair.
void GetPointIntStats()
Get point count stats.
const ControlMeasure * GetMeasure(QString serialNumber) const
Get a control measure based on its cube's serial number.
int size() const
How many serial number / filename combos are in the list.
double GetMinLineResidual() const
Determine the minimum line error of all points in the network.
File name manipulation and expansion.
void SetMaximumSteps(const int steps)
This sets the maximum number of steps in the process.
int NumValidPoints() const
Returns the Number of Valid (Not Ignored) Points in the Control Net.
double GetAvgPixelShift() const
Get network Avg PixelShift.
double GetMaxSampleResidual() const
Determine the maximum sample error of all points in the network.
QMap< int, double > mPointDoubleStats
Contains QMap of different computed stats.
Statistics mConvexHullRatioStats
min, max, average convex hull stats
QMap< int, int > mPointIntStats
Contains QMap of different count stats.
Statistics GetStatistic(double(ControlMeasure::*statFunc)() const) const
This function will call a given method on every control measure that this point has.
double Maximum() const
Returns the absolute maximum double found in all data passed through the AddData method.
int NumIgnoredMeasures() const
Returns the total Number of Ignored Measures in the Control Net.
int GetNumEditLockMeasures()
Return the total number of edit locked measures for all control points in the network.
double GetMaximumResidual() const
Determine the maximum error of all points in the network.
QList< ControlMeasure * > GetMeasuresInCube(QString serialNumber)
Get all the measures pertaining to a given cube serial number.
QString GetId() const
Return the Id of the control point.
BigInt ValidPixels() const
Returns the total number of valid pixels processed.
QVector< double > GetImageStatsBySerialNum(QString psSerialNum) const
Returns the Image Stats by Serial Number.
double GetMinSampleResidual() const
Determine the minimum sample error of all points in the network.
int GetNumEditLockPoints()
Returns the number of edit locked control points.
ControlNetStatistics(ControlNet *pCNet, const QString &psSerialNumFile, Progress *pProgress=0)
Constructor.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Serial Number list generator.
int GetNumValidMeasures() const
bool IsSpecial(const double d)
Returns if the input pixel is special.
QList< QString > GetCubeSerials() const
Use this method to get a complete list of all the cube serial numbers in the network.
QString serialNumber(const QString &filename)
Return a serial number given a filename.
void GetPointDoubleStats()
Get Point stats for Residuals and Shifts.
Cube * OpenCube(const QString &cubeFileName)
This method opens a cube.
~ControlNetStatistics()
Destructor.
int NumConstrainedPoints() const
Returns the number of Constrained Points in Control Net.
QString sBoolean[]
String values for Boolean.
Class for quick re-accessing of cubes based on file name.
int NumFixedPoints() const
Returns the Number of Fixed Points in the Control Net.
QMap< QString, QVector< double > > mImageMap
Contains stats by Image/Serial Num.
double GetMaxLineResidual() const
Determine the maximum line error of all points in the network.
double GetMaxSampleShift() const
Get network Max SampleShift.
@ Fixed
A Fixed point is a Control Point whose lat/lon is well established and should not be changed.
void InitPointDoubleStats()
Init Pointstats std::vector.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
double GetMinPixelShift() const
Get network Min PixelShift.
int NumValidMeasures() const
Returns the total Number of valid Measures in the Control Net.
int GetNumPoints() const
Return the number of control points in the network.
Contains multiple PvlContainers.
void PrintImageStats(const QString &psImageFile)
Print the Image Stats into specified output file.
@ MinimumPixelZScore
Control measures store z-scores in pairs.
double GetAverageResidual() const
Determine the average error of all points in the network.
double Minimum() const
Returns the absolute minimum double found in all data passed through the AddData method.
int GetNumLockedMeasures() const
Returns the number of locked control measures.
@ Constrained
A Constrained point is a Control Point whose lat/lon/radius is somewhat established and should not be...
void SetText(const QString &text)
Changes the value of the text string reported just before 0% processed.
bool IsEditLocked() const
Return value for p_editLock or implicit lock on reference measure.
QString sPointType[]
String names for Point Type.
QMap< QString, bool > mSerialNumMap
Whether serial# is part of ControlNet.
IO Handler for Isis Cubes.
double GetMinSampleShift() const
Get network Min SampleShift.
void GenerateControlNetStats(PvlGroup &pStatsGrp)
Generate the Control Net Stats into the PvlGroup.
void InitSerialNumMap()
Init SerialNum std::map.
double GetMinimumResidual() const
Determine the minimum error of all points in the network.
Program progress reporter.
const double Null
Value for an Isis Null pixel.
double Average() const
Computes and returns the average.
void GeneratePointStats(const QString &psPointFile)
Generate stats like Ignored, Fixed, Total Measures, Ignored by Control Point.
int NumMeasures() const
Returns the total Number of Measures in the Control Net.
@ Free
A Free point is a Control Point that identifies common measurements between two or more cubes.
This is free and unencumbered software released into the public domain.
PointType GetType() const
@ GoodnessOfFit
GoodnessOfFit is pointreg information for reference measures.
void SetNumOpenCubes(unsigned int numCubes)
This sets the maximum number of opened cubes for this instance of CubeManager.
QString fileName(const QString &sn)
Return a filename given a serial number.
SerialNumberList mSerialNumList
Serial Number List.
double GetMinLineShift() const
Get Min and Max LineShift.
int NumFreePoints() const
Returns the number of Constrained Points in Control Net.
This is free and unencumbered software released into the public domain.
Progress * mProgress
Progress state.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
ControlNet * mCNet
Control Network.