File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
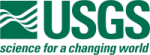 |
Isis 3 Programmer Reference
|
8 #include "CubeBsqHandler.h"
14 #include "IException.h"
16 #include "PvlKeyword.h"
17 #include "PvlObject.h"
18 #include "RawCubeChunk.h"
34 CubeBsqHandler::CubeBsqHandler(QFile * dataFile,
35 const QList<int> *virtualBandList,
const Pvl &labels,
bool alreadyOnDisk)
36 :
CubeIoHandler(dataFile, virtualBandList, labels, alreadyOnDisk) {
38 int numLinesInChunk = 1;
42 int sizeLimit = 1024 * 1024 * 1024;
43 int maxNumLines = (sizeLimit) / (
SizeOf(
pixelType()) * numSamplesInChunk);
71 PvlContainer::Replace);
81 if(dataFile->seek(startByte)) {
82 QByteArray binaryData = dataFile->read(chunkToFill.
getByteCount());
91 IString msg =
"Reading from the file [" + dataFile->fileName() +
"] "
92 "failed with reading [" +
94 "] bytes at position [" + QString::number(startByte) +
"]";
103 bool success =
false;
106 if(dataFile->seek(startByte)) {
115 IString msg =
"Writing to the file [" + dataFile->fileName() +
"] "
116 "failed with writing [" +
118 "] bytes at position [" + QString::number(startByte) +
"]";
134 int chunkDimensionSize;
136 if (dimensionSize <= maxSize) {
137 chunkDimensionSize = dimensionSize;
141 int greatestDivisor = maxSize;
142 while (dimensionSize % greatestDivisor > 0) {
145 chunkDimensionSize = greatestDivisor;
147 return chunkDimensionSize;
int SizeOf(Isis::PixelType pixelType)
Returns the number of bytes of the specified PixelType.
A section of raw data on the disk.
int findGoodSize(int maxSize, int dimensionSize) const
This method attempts to compute a good chunk line size.
@ Io
A type of error that occurred when performing an actual I/O operation.
Contains Pvl Groups and Pvl Objects.
A single keyword-value pair.
PixelType pixelType() const
void addKeyword(const PvlKeyword &keyword, const InsertMode mode=Append)
Add a keyword to the container.
BigInt getBytesPerChunk() const
Container for cube-like labels.
QByteArray & getRawData() const
BigInt getChunkStartByte(const RawCubeChunk &chunk) const
This is a helper method that goes from chunk to file position.
void updateLabels(Pvl &labels)
Function to update the labels with a Pvl object.
virtual void writeRaw(const RawCubeChunk &chunkToWrite)
This needs to write the chunkToWrite directly to disk with no modifications to the data itself.
BigInt getDataStartByte() const
Handles converting buffers to and from disk.
long long int BigInt
Big int.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
int getChunkIndex(const RawCubeChunk &) const
Given a chunk, what's its index in the file.
void setRawData(QByteArray rawData)
Sets the chunk's raw data.
Namespace for the standard library.
virtual void readRaw(RawCubeChunk &chunkToFill)
This needs to populate the chunkToFill with unswapped raw bytes from the disk.
void clearCache(bool blockForWriteCache=true) const
Free all cube chunks (cached cube data) from memory and write them to disk.
Adds specific functionality to C++ strings.
void setChunkSizes(int numSamples, int numLines, int numBands)
This should be called once from the child constructor.
This is free and unencumbered software released into the public domain.
~CubeBsqHandler()
The destructor writes all cached data to disk.