Loading [MathJax]/jax/output/NativeMML/config.js
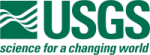 |
Isis 3 Programmer Reference
|
8 #include "RawCubeChunk.h"
16 #include "Displacement.h"
18 #include "IException.h"
58 int endSample,
int endLine,
int endBand,
103 IString msg =
"Cannot set raw data on a RawCubeChunk with a differently "
122 return ((
unsigned char *)
m_rawBuffer->data())[offset];
void setDirty(bool dirty)
Sets the chunk's dirty flag, indicating whether or not the chunk's data matches the data that is on d...
bool m_dirty
True if the data does not match what is on disk.
char * m_rawBufferInternalPtr
This is the internal pointer to the raw buffer for performance.
float getFloat(int offset) const
int m_startBand
The one-based (inclusive) start band of the cube chunk.
int m_bandCount
The number of bands in the cube chunk.
RawCubeChunk(const Area3D &placement, int numBytes)
This constructor creates a new cube chunk based on the provided placement and data size.
Distance getDepth() const
Returns the depth (in the Z dimension) of the 3D area.
int m_startLine
The one-based (inclusive) start line of the cube chunk.
int m_startSample
The one-based (inclusive) start sample of the cube chunk.
int m_sampleCount
The number of samples in the cube chunk.
short getShort(int offset) const
Displacement getStartX() const
Returns the leftmost X position of the 3D area.
QByteArray * m_rawBuffer
This is the raw data to be put on disk.
int m_lineCount
The number of lines in the cube chunk.
virtual ~RawCubeChunk()
The destructor.
Displacement getStartZ() const
Returns the frontmost Z position of the 3D area.
Distance getWidth() const
Returns the width (in the X dimension) of the 3D area.
void setData(unsigned char value, int offset)
Sets the char at the given offset in the raw data buffer of this chunk.
double pixels(double pixelsPerMeter=1.0) const
Get the displacement in pixels using the given conversion ratio.
void setRawData(QByteArray rawData)
Sets the chunk's raw data.
Distance getHeight() const
Returns the height (in the Y dimension) of the 3D area.
unsigned char getChar(int offset) const
This method is currently not in use due to a faster way of getting data from the buffer (through the ...
@ Programmer
This error is for when a programmer made an API call that was illegal.
Represents a 3D area (a 3D "cube")
Adds specific functionality to C++ strings.
double pixels(double pixelsPerMeter=1.0) const
Get the distance in pixels using the given conversion ratio.
Displacement getStartY() const
Returns the topmost Y position of the 3D area.
This is free and unencumbered software released into the public domain.