Loading [MathJax]/jax/output/NativeMML/config.js
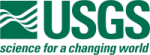 |
Isis 3 Programmer Reference
|
7 #include "CubeDataThreadTester.h"
13 #include <QReadWriteLock>
17 #include "CubeDataThread.h"
56 SLOT(ReadCube(
int,
int,
int,
int,
int,
int,
void *)));
61 SLOT(ReadWriteCube(
int,
int,
int,
int,
int,
int,
void *)));
80 QThread::yieldCurrentThread();
100 cout <<
"=============== Testing Basic Read ===============" << endl;
112 cout <<
"=============== Testing Multiple Non-Conflicting Cube Reads " <<
113 "===============" << endl;
127 cout <<
"=============== Testing Exact Overlap Cube Reads ==============="
142 cout <<
"=============== Testing Basic R/W ===============" << endl << endl;
155 cout <<
"=============== Testing Multiple Non-Conflicting Cube R/W " <<
156 "===============" << endl << endl;
173 cout <<
"=============== Testing Conflicting Cube R/W ==============="
187 cout <<
" Breaking Deadlock From Test 3" << endl;
193 cout <<
" Notify done with first brick" << endl;
207 cout <<
"=============== Testing Change Notification ==============="
230 cout <<
" CubeDataThreadTester::ReadBrick" << endl;
232 cout <<
" Requester is me? " << ((
this == requester) ?
"Yes" :
"No")
235 if(
this != requester)
return;
237 cout <<
" Data:" << endl;
239 for(
int i = 0; i < data->
size(); i++) {
240 if(i == 0) cout <<
" ";
241 if(i % 6 == 6 - 1 && i != data->
size() - 1) {
242 cout << data->
at(i) << endl <<
" ";
244 else if(i == data->
size() - 1) {
245 cout << data->
at(i) << endl;
248 cout << data->
at(i) <<
"\t";
254 cout <<
" Notify done with this brick" << endl;
258 cout <<
" Notify done with first brick" << endl;
266 cache.first = cubeId;
285 cout <<
" CubeDataThreadTester::ReadWriteBrick" << endl;
293 cout <<
" Changing Brick : Index 0 Becoming 5" << endl;
296 cout <<
" Old Data: " << endl;
298 for(
int i = 0; i < data->
size(); i++) {
299 if(i == 0) cout <<
" ";
300 if(i % 6 == 6 - 1 && i != data->
size() - 1) {
301 cout << data->
at(i) << endl <<
" ";
303 else if(i == data->
size() - 1) {
304 cout << data->
at(i) << endl;
307 cout << data->
at(i) <<
"\t";
313 cout <<
" New Data: " << endl;
315 for(
int i = 0; i < data->
size(); i++) {
316 if(i == 0) cout <<
" ";
317 if(i % 6 == 6 - 1 && i != data->
size() - 1) {
318 cout << data->
at(i) << endl <<
" ";
320 else if(i == data->
size() - 1) {
321 cout << data->
at(i) << endl;
324 cout << data->
at(i) <<
"\t";
331 cout <<
" Notify done with this brick" << endl;
335 cout <<
" Notify done with first brick" << endl;
343 cache.first = cubeId;
361 cout <<
" CubeDataThreadTester::BrickChanged" << endl;
362 cout <<
" Data:" << endl;
364 for(
int i = 0; i < data->
size(); i++) {
365 if(i == 0) cout <<
" ";
366 if(i % 6 == 6 - 1 && i != data->
size() - 1) {
367 cout << data->
at(i) << endl <<
" ";
369 else if(i == data->
size() - 1) {
370 cout << data->
at(i) << endl;
373 cout << data->
at(i) <<
"\t";
int p_numTestsDone
The count of completed tests.
CubeDataThread * p_cubeDataThread
The data thread being tested.
void BrickChanged(int cubeId, const Isis::Brick *data)
This is called when a brick is written.
void run()
This thread is centered completely around its event loop.
void ReadCubeTest2(int, int)
This tests two basic reads with no conflicts.
void RequestReadCube(int cubeId, int startSample, int startLine, int endSample, int endLine, int band, void *caller)
Ask for a brick for reading.
void NotifyDoneWithData(int, const Isis::Brick *)
Let the cube data thread know we're no longer working with a particular brick.
QVector< QPair< int, const Isis::Brick * > > * p_cachedDoneBricks
A list of bricks we haven't send the done signal for.
void ReadCubeTest(int)
This tests a basic read.
Buffer for containing a three dimensional section of an image.
void WriteCubeTest(int)
This tests a basic write.
Encapsulation of Cube I/O with Change Notifications.
void AddChangeListener()
You must call this method after connecting to the BrickChanged signal, otherwise you are not guarante...
bool p_notifyDone
True if we will notify done on the next brick received for R/W.
virtual ~CubeDataThreadTester()
This cleans up the cube data thread.
void NotifyChangeTest(int)
This test tests this automatic change notifications.
void ReadCubeTest3(int)
This tests an overlapping read.
bool p_execStarted
True if this thread is started.
double at(const int index) const
Returns the value in the shape buffer at the given index.
void ReadWriteBrick(void *requester, int cubeId, Isis::Brick *data)
This is called when a brick is given for R/W.
void WriteCubeTest3BreakDeadlock()
This test breaks the deadlock caused by the third write test.
Namespace for the standard library.
This is free and unencumbered software released into the public domain.
int size() const
Returns the total number of pixels in the shape buffer.
This is free and unencumbered software released into the public domain.
void ReadBrick(void *requester, int cubeId, const Isis::Brick *data)
This is called when a brick is read.
void Connect()
This connects this class' signals and slots with CubeDataThread's signals and slots.
void RequestReadWriteCube(int cubeId, int startSample, int startLine, int endSample, int endLine, int band, void *caller)
Ask for a brick for reading and writing.
void WriteCubeTest2(int, int)
This tests two non-conflicting writes.
This is free and unencumbered software released into the public domain.
void WriteCubeTest3(int)
This tests two conflicting* writes.