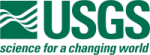 |
Isis 3 Programmer Reference
|
8 #include "CubeTileHandler.h"
12 #include "IException.h"
14 #include "PvlObject.h"
15 #include "PvlKeyword.h"
16 #include "RawCubeChunk.h"
32 CubeTileHandler::CubeTileHandler(QFile * dataFile,
33 const QList<int> *virtualBandList,
const Pvl &labels,
bool alreadyOnDisk)
34 :
CubeIoHandler(dataFile, virtualBandList, labels, alreadyOnDisk) {
69 PvlContainer::Replace);
71 PvlContainer::Replace);
73 PvlContainer::Replace);
83 if(dataFile->seek(startByte)) {
84 QByteArray binaryData = dataFile->read(chunkToFill.
getByteCount());
93 IString msg =
"Reading from the file [" + dataFile->fileName() +
"] "
94 "failed with reading [" +
96 "] bytes at position [" + QString::number(startByte) +
"]";
104 bool success =
false;
107 if(dataFile->seek(startByte)) {
116 IString msg =
"Writing to the file [" + dataFile->fileName() +
"] "
117 "failed with writing [" +
119 "] bytes at position [" + QString::number(startByte) +
"]";
138 if(dimensionSize <= maxSize) {
139 ideal = dimensionSize;
142 int greatestDividend = maxSize;
144 while(greatestDividend > ideal) {
145 if(dimensionSize % greatestDividend == 0) {
146 ideal = greatestDividend;
int SizeOf(Isis::PixelType pixelType)
Returns the number of bytes of the specified PixelType.
A section of raw data on the disk.
@ Io
A type of error that occurred when performing an actual I/O operation.
Contains Pvl Groups and Pvl Objects.
A single keyword-value pair.
PixelType pixelType() const
void addKeyword(const PvlKeyword &keyword, const InsertMode mode=Append)
Add a keyword to the container.
BigInt getBytesPerChunk() const
Container for cube-like labels.
QByteArray & getRawData() const
~CubeTileHandler()
Writes all data from memory to disk.
int getSampleCountInChunk() const
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
BigInt getTileStartByte(const RawCubeChunk &chunk) const
This is a helper method that goes from chunk to file position.
BigInt getDataStartByte() const
bool hasKeyword(const QString &kname, FindOptions opts) const
See if a keyword is in the current PvlObject, or deeper inside other PvlObjects and Pvlgroups within ...
Handles converting buffers to and from disk.
long long int BigInt
Big int.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
void updateLabels(Pvl &label)
Update the cube labels so that this cube indicates what tile size it used.
int getChunkIndex(const RawCubeChunk &) const
Given a chunk, what's its index in the file.
void setRawData(QByteArray rawData)
Sets the chunk's raw data.
virtual void readRaw(RawCubeChunk &chunkToFill)
This needs to populate the chunkToFill with unswapped raw bytes from the disk.
Namespace for the standard library.
void clearCache(bool blockForWriteCache=true) const
Free all cube chunks (cached cube data) from memory and write them to disk.
Adds specific functionality to C++ strings.
void setChunkSizes(int numSamples, int numLines, int numBands)
This should be called once from the child constructor.
virtual void writeRaw(const RawCubeChunk &chunkToWrite)
This needs to write the chunkToWrite directly to disk with no modifications to the data itself.
int getLineCountInChunk() const
This is free and unencumbered software released into the public domain.
int findGoodSize(int maxSize, int dimensionSize) const
This is a helper method that tries to compute a good tile size for one of the cube's dimensions (samp...