File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
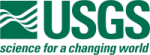 |
Isis 3 Programmer Reference
|
6 #include <QApplication>
11 #include <QMessageBox>
15 #include <QPrintDialog>
19 #include "BrowseDialog.h"
20 #include "CubeAttribute.h"
22 #include "FileDialog.h"
23 #include "Interpolator.h"
24 #include "MainWindow.h"
25 #include "MdiCubeViewport.h"
26 #include "OriginalLabel.h"
28 #include "ProcessRubberSheet.h"
29 #include "ProcessByLine.h"
32 #include "SaveAsDialog.h"
34 #include "ViewportMainWindow.h"
35 #include "Workspace.h"
48 p_dir =
"/thisDirDoesNotExist!";
50 p_open->setShortcut(Qt::CTRL + Qt::Key_O);
51 p_open->setText(
"&Open...");
53 p_open->setToolTip(
"Open cube");
55 "<b>Function:</b> Open an <i>Isis cube</i> in new viewport \
56 <p><b>Shortcut:</b> Ctrl+O\n</p> \
57 <p><b>Hint:</b> Use Ctrl or Shift in file dialog to open \
59 p_open->setWhatsThis(whatsThis);
60 connect(
p_open, SIGNAL(triggered()),
this, SLOT(
open()));
63 p_browse->setShortcut(Qt::CTRL + Qt::Key_B);
65 p_browse->setToolTip(
"Browse cubes");
67 "<b>Function:</b> Browse a <i>Isis cubes</i> in new viewport \
68 <p><b>Shortcut:</b> Ctrl+B\n</p>";
73 p_save->setShortcut(Qt::CTRL + Qt::Key_S);
76 p_save->setToolTip(
"Save");
78 "<b>Function:</b> Save changes to the current Cube \
79 <p><b>Shortcut:</b> Ctrl+S</p>";
80 p_save->setWhatsThis(whatsThis);
81 connect(
p_save, SIGNAL(triggered()),
this, SLOT(
save()));
89 "<b>Function:</b> Save the current Cube to the specified location";
99 "<b>Function:</b> Save the current Cube's Whatsthis Info to the specified location";
109 "<b>Function:</b> Save visible contents of the active \
110 viewport as a png, jpg, tiff \
111 <p><b>Hint:</b> Your local installation of Qt may not support \
112 all formats. Reinstall Qt if necessary</p>";
122 "<b>Function:</b> Save all open cubes \
123 to a .lis file containing their file names";
130 p_print->setShortcut(Qt::CTRL + Qt::Key_P);
134 "<b>Function:</b> Print visible contents of the active viewport \
135 <p><b>Shortcut:</b> Ctrl+P</b>";
136 p_print->setWhatsThis(whatsThis);
137 connect(
p_print, SIGNAL(triggered()),
this, SLOT(
print()));
144 "<b>Function:</b> Close all cube viewports.";
148 p_exit->setShortcut(Qt::CTRL + Qt::Key_Q);
152 "<b>Function:</b> Quit qview \
153 <p><b>Shortcut:</b> Ctrl+Q</p>";
154 p_exit->setWhatsThis(whatsThis);
155 connect(
p_exit, SIGNAL(triggered()),
this, SLOT(
exit()));
192 ws, SLOT(addCubeViewport(QString)));
194 connect(
p_closeAll, SIGNAL(triggered()), ws->
mdiArea(), SLOT(closeAllSubWindows()));
221 if (!
p_dir.exists()) {
222 p_dir = QDir::current();
242 if (!
p_dir.exists()) {
243 p_dir = QDir::current();
261 QMessageBox::information((
QWidget *)parent(),
"Error",
"No active cube to save");
266 p_save->setEnabled(
false);
287 QMessageBox::information((
QWidget *)parent(),
"Error",
"No active cube to save");
294 if (!
p_dir.exists()) {
320 QMessageBox::information((
QWidget *)parent(),
"Error",
321 "No active cube to save");
327 QMessageBox::information((
QWidget *)parent(),
"Error",
328 "No output file selected");
335 for (it = vwportList->begin(); it != vwportList->end(); ++it){
336 if (QString((*it)->cube()->fileName()) == psOutFile) {
337 QMessageBox::information((
QWidget *)parent(),
"Error",
338 "Output File is already open\n\""+ psOutFile +
"\"");
359 if (
p_saveAsDialog->getSaveAsType() != SaveAsDialog::ExportAsIs ||
373 double dStartSample=0, dEndSample=0, dStartLine=0, dEndLine=0;
374 p_lastViewport->getCubeArea(dStartSample, dEndSample, dStartLine, dEndLine);
376 if (
p_saveAsDialog->getSaveAsType() == SaveAsDialog::ExportFullRes ||
380 int numSamples = (int)((dEndSample - dStartSample + 1) + 0.5);
381 int numLines = (int)((dEndLine - dStartLine + 1) + 0.5);
386 else if (
p_saveAsDialog->getSaveAsType() == SaveAsDialog::ExportAsIs ) {
394 p_save->setEnabled(
false);
414 double dStartSample=0, dEndSample=0, dStartLine=0, dEndLine=0;
415 p_lastViewport->getCubeArea(dStartSample, dEndSample, dStartLine, dEndLine);
417 double ins = dEndSample - dStartSample + 1;
418 double inl = dEndLine - dStartLine + 1;
420 double ons = (int)(ins * dScale + 0.5);
421 double onl = (int)(inl * dScale + 0.5);
432 imgEnlarge->
SetInputArea((
int)dStartSample, (
int)dEndSample, (
int)dStartLine, (
int)dEndLine);
445 QObject::tr(
"The cube could not be saved, unable to create the cube"),
464 double dStartSample=0, dEndSample=0, dStartLine=0, dEndLine=0;
465 p_lastViewport->getCubeArea(dStartSample, dEndSample, dStartLine, dEndLine);
467 double ins = dEndSample - dStartSample + 1;
468 double inl = dEndLine - dStartLine + 1;
470 double ons = (int)(ins * dScale + 0.5);
471 double onl = (int)(inl * dScale + 0.5);
474 std::vector<QString> bands = cai.
bands();
475 int inb = bands.size();
479 for(
int i = 1; i <= inb; i++) {
496 near->
setInputBoundary((
int)dStartSample, (
int)dEndSample, (
int)dStartLine, (
int)dEndLine);
511 QObject::tr(
"The cube could not be saved, unable to create the cube"),
553 Cube *ocube,
int piNumSamples,
int piNumLines,
int piNumBands) {
576 double base = icube->
base();
584 (ocube->
pixelType() != Isis::UnsignedInteger) &&
585 (ocube->
pixelType() != Isis::SignedInteger)) {
586 QString msg =
"Looks like your refactoring to add different pixel types";
587 msg +=
" you'll need to make changes here";
591 QString msg =
"You've chosen to reduce your output PixelType for [" +
592 psOutFile +
"] you must specify the output pixel range too";
601 int needLabBytes = icube->
labelSize(
true) + (1024 * 6);
612 for(
int i = 0; i < incube.
groups(); i++) {
618 for(
int i = 0; i < inlab.
objects(); i++) {
627 inlab = *icube->
label();
628 for(
int i = 0; i < inlab.
objects(); i++) {
637 inlab = *icube->
label();
638 for(
int i = 0; i < inlab.
objects(); i++) {
669 numBricks = ibrick.
Bricks();
672 numBricks = obrick.
Bricks();
678 for(
int i = 0; i < numBricks; i++) {
681 copy(ibrick, obrick);
682 ocube->
write(obrick);
701 Cube *pOutCube,
int pNumSamples,
int pNumLines) {
703 double dStartSample=0, dEndSample=0, dStartLine=0, dEndLine=0;
704 p_lastViewport->getCubeArea(dStartSample, dEndSample, dStartLine, dEndLine);
720 dEndLine, dEndSample, 1.0, 1.0);
726 for(
int iBand=1; iBand<=iNumBands; iBand++) {
728 for(
int iLine=(
int)dStartLine; iLine<=(int)dEndLine; iLine++) {
730 pInCube->
read(iPortal);
733 pOutCube->
read(oPortal);
735 oPortal.
Copy(iPortal);
736 pOutCube->
write(oPortal);
751 QMessageBox::information((
QWidget *)parent(),
"Error",
"No active cube to save info");
757 QFileDialog::getSaveFileName((
QWidget *)parent(),
758 "Choose output file",
760 QString(
"PVL Files (*.pvl)"));
763 if (output.isEmpty()) {
766 else if (!output.endsWith(
".pvl")) {
772 whatsThisPvl.
write(output);
803 QMessageBox::information((
QWidget *)parent(),
"Error",
"No active cube to export");
808 QFileDialog::getSaveFileName((
QWidget *)parent(),
809 QString(
"Choose output file"),
811 QString(
"PNG (*.png);;JPG (*.jpg);;TIF (*.tif)"));
812 if (output.isEmpty())
return;
816 QString format = QFileInfo(output).suffix();
818 if (format.isEmpty()) {
819 if (output.endsWith(
'.')) {
820 output.append(QString(
"png"));
823 output.append(QString(
".png"));
826 else if (format.compare(
"png", Qt::CaseInsensitive) &&
827 format.compare(
"jpg", Qt::CaseInsensitive) &&
828 format.compare(
"tif", Qt::CaseInsensitive)) {
830 QMessageBox::information((
QWidget *)parent(),
"Error", format +
" is an invalid extension.");
838 if (!pm.save(output)) {
839 QMessageBox::information((
QWidget *)parent(),
"Error",
"Unable to save " + output);
855 QMessageBox::information((
QWidget *)parent(),
"Error",
"No active cubes to export");
864 if (window == NULL) {
865 QMessageBox::critical((
QWidget *)parent(),
"Error",
"There was an error reading the viewport window.");
872 for (
int i = 0; i < openCubes.size(); i++) {
879 cubeFilePaths.append(cubeFileName.absoluteFilePath());
882 QString fileName = QFileDialog::getSaveFileName((
QWidget *) parent(),
883 "Export to cube list",
885 "Cube List (*.lis)");
887 if (!fileName.contains(
".lis")) {
888 fileName.append(
".lis");
891 QFile outputFile(fileName);
892 outputFile.open(QIODevice::WriteOnly | QIODevice::Text);
894 QTextStream out(&outputFile);
897 for (
int i = 0; i < cubeFilePaths.size(); i++){
898 out << cubeFilePaths.value(i) <<
"\n";
911 QMessageBox::information((
QWidget *)parent(),
"Error",
"No active cube to print");
916 static QPrinter *printer = NULL;
917 if (printer == NULL) printer =
new QPrinter;
918 printer->setPageSize(QPrinter::Letter);
919 printer->setColorMode(QPrinter::GrayScale);
920 if (
cubeViewport()->isColor()) printer->setColorMode(QPrinter::Color);
922 QPrintDialog printDialog(printer, (
QWidget *)parent());
923 if (printDialog.exec() == QDialog::Accepted) {
926 QImage img = pixmap.toImage();
929 QPainter painter(printer);
930 QRect rect = painter.viewport();
931 QSize size = img.size();
932 size.scale(rect.size(), Qt::KeepAspectRatio);
933 painter.setViewport(rect.x(), rect.y(),
934 size.width(), size.height());
935 painter.setWindow(img.rect());
936 painter.drawImage(0, 0, img);
951 for(
int i = 0; i < (int)tempList.size(); i++) {
956 if (!d->parentWidget()->close()) {
985 p_save->setEnabled(enable);
998 p_save->setEnabled(
false);
1013 if (
cubeViewport()->parentWidget()->windowTitle().endsWith(
"*")) {
1014 p_save->setEnabled(
true);
1018 p_save->setEnabled(
false);
double base() const
Returns the base value for converting 8-bit/16-bit pixels to 32-bit.
Cube display widget for certain Isis MDI applications.
virtual QString fileName() const
Returns the opened cube's filename.
PvlGroup & group(const int index)
Return the group at the specified index.
@ Io
A type of error that occurred when performing an actual I/O operation.
Contains Pvl Groups and Pvl Objects.
Buffer for containing a two dimensional section of an image.
void SetSubArea(const int orignl, const int origns, const int sl, const int ss, const int el, const int es, const double linc, const double sinc)
Defines the subarea.
A single keyword-value pair.
This is free and unencumbered software released into the public domain.
void getAllWhatsThisInfo(Pvl &pWhatsThisPvl)
Get All WhatsThis info - viewport, cube, area in PVL format.
Enlarge the pixel dimensions of an image.
void read(Blob &blob, const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
This method will read data from the specified Blob object.
int groups() const
Returns the number of groups contained.
void setLabelSize(int labelBytes)
Used prior to the Create method, this will allocate a specific number of bytes in the label area for ...
int labelSize(bool actual=false) const
Returns the number of bytes used by the label.
virtual Cube * SetOutputCube(const QString &fname, const CubeAttributeOutput &att)
Create the output file.
void close(bool remove=false)
Closes the cube and updates the labels.
Container for cube-like labels.
int objects() const
Returns the number of objects.
Manipulate and parse attributes of output cube filenames.
Class for browsing cubes.
virtual void StartProcess(Transform &trans, Interpolator &interp)
Applies a Transform and an Interpolator to every pixel in the output cube.
Functor for reduce using near functionality.
void write(const QString &file)
Opens and writes PVL information to a file and handles the end of line sequence.
PvlObject & object(const int index)
Return the object at the specified index.
Buffer for containing a three dimensional section of an image.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
bool begin()
Moves the shape buffer to the first position.
int Bricks()
Returns the number of Bricks in the cube.
virtual void EndProcess()
End the processing sequence and cleans up by closing cubes, freeing memory, etc.
Isis::PvlGroup UpdateOutputLabel(Isis::Cube *pOutCube)
Create label for the reduced output image.
Buffer for reading and writing cube data.
Class for browsing cubes.
void ProcessCubeInPlace(const Functor &funct, bool threaded=true)
Same functionality as StartProcess(void funct(Isis::Buffer &inout)) using Functors.
@ AttachedLabel
The input label is embedded in the image file.
double multiplier() const
Returns the multiplier value for converting 8-bit/16-bit pixels to 32-bit.
void Copy(const Buffer &in, bool includeRawBuf=true)
Allows copying of the buffer contents to another Buffer.
Widget to save(Save As) Isis cubes(used in qview) to display the FileDialog to select the output cube...
void UpdateLabel(Cube *icube, Cube *ocube, PvlGroup &results)
Modifies a label for a file containing a subarea.
Contains multiple PvlContainers.
This was called the Qisis MainWindow.
QMdiArea * mdiArea()
This method returns the QMdiArea.
void ClearInputCubes()
Close owned input cubes from the list and clear the list.
void setDimensions(int ns, int nl, int nb)
Used prior to the Create method to specify the size of the cube.
void create(const QString &cfile)
This method will create an isis cube for writing.
bool isNamed(const QString &match) const
Returns whether the given string is equal to the container name or not.
PixelType pixelType() const
Return the pixel type as an Isis::PixelType.
virtual Isis::Cube * SetInputCube(const QString ¶meter, const int requirements=0)
Opens an input cube specified by the user and verifies requirements are met.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
bool propagateMinimumMaximum() const
Return true if the min/max are to be propagated from an input cube.
void setInputBoundary(int startSample, int endSample, int startLine, int endLine)
Parameters to input image sub area.
PvlGroup UpdateOutputLabel(Cube *pOutCube)
Update the Mapping, Instrument, and AlphaCube groups in the output cube label.
IO Handler for Isis Cubes.
QString name() const
Returns the container name.
Widget to display Isis cubes for qt apps.
virtual int bandCount() const
Returns the number of virtual bands for the cube.
void addGroup(const Isis::PvlGroup &group)
Add a group to the object.
void setByteOrder(ByteOrder byteOrder)
Used prior to the Create method, this will specify the byte order of pixels, either least or most sig...
virtual Isis::Cube * SetOutputCube(const QString ¶meter)
Allocates a user-specified output cube whose size matches the first input cube.
void SetInputArea(double pdStartSample, double pdEndSample, double pdStartLine, double pdEndLine)
Sets the sub area dimensions of the input image.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Read and store original labels.
PixelType pixelType() const
ByteOrder byteOrder() const
Return the byte order as an Isis::ByteOrder.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
void write(Blob &blob, bool overwrite=true)
This method will write a blob of data (e.g.
Apply corrections to a cube label for subarea extraction.
double maximum() const
Return the output cube attribute maximum.
double minimum() const
Return the output cube attribute minimum.
void setFormat(Format format)
Used prior to the Create method, this will specify the format of the cube, either band,...
void setPixelType(PixelType pixelType)
Used prior to the Create method, this will specify the output pixel type.
Derivative of Process, designed for geometric transformations.
void setBaseMultiplier(double base, double mult)
Used prior to the Create method, this will specify the base and multiplier for converting 8-bit/16-bi...
OriginalLabel readOriginalLabel(const QString &name="IsisCube") const
Read the original PDS3 label from a cube.
This is free and unencumbered software released into the public domain.
void open(const QString &cfile, QString access="r")
This method will open an isis cube for reading or reading/writing.
void EndProcess()
End the processing sequence and cleans up by closing cubes, freeing memory, etc.
Cube::Format fileFormat() const
Return the file format an Cube::Format.
void setMinMax(double min, double max)
Used prior to the Create method, this will compute a good base and multiplier value given the minimum...
Isis::Cube * SetInputCube(const QString ¶meter, const int requirements=0)
Opens an input cube specified by the user and verifies requirements are met.
bool propagatePixelType() const
Return true if the pixel type is to be propagated from an input cube.
void SetPosition(const double sample, const double line, const int band)
Sets the line and sample position of the buffer.
This is free and unencumbered software released into the public domain.
void reopen(QString access="r")
This method will reopen an isis sube for reading or reading/writing.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
void setLabelsAttached(bool attached)
Use prior to calling create, this sets whether or not to use separate label and data files.