File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
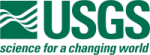 |
Isis 3 Programmer Reference
|
12 #include <QFileDialog>
13 #include <QHBoxLayout>
18 #include <QToolButton>
21 #include "Application.h"
24 #include "GuiCubeParameter.h"
25 #include "GuiInputAttribute.h"
26 #include "GuiOutputAttribute.h"
27 #include "IException.h"
28 #include "ProgramLauncher.h"
30 #include "UserInterface.h"
44 int group,
int param) :
49 fileAction->setText(
"Select Cube");
50 connect(fileAction, SIGNAL(triggered(
bool)),
this, SLOT(
SelectFile()));
51 p_menu->addAction(fileAction);
54 attAction->setText(
"Change Attributes ...");
55 connect(attAction, SIGNAL(triggered(
bool)),
this, SLOT(
SelectAttribute()));
56 p_menu->addAction(attAction);
59 viewAction->setText(
"View cube");
60 connect(viewAction, SIGNAL(triggered(
bool)),
this, SLOT(
ViewCube()));
61 p_menu->addAction(viewAction);
64 labAction->setText(
"View labels");
65 connect(labAction, SIGNAL(triggered(
bool)),
this, SLOT(
ViewLabel()));
66 p_menu->addAction(labAction);
68 p_fileButton->setMenu(p_menu);
69 p_fileButton->setPopupMode(QToolButton::MenuButtonPopup);
70 QString optButtonWhatsThisText =
"<p><b>Function:</b> \
71 Opens a file chooser window to select a file from</p> <p>\
72 <b>Hint: </b> Click the arrow for more cube parameter options</p>";
73 p_fileButton->setWhatsThis(optButtonWhatsThisText);
93 QString curAtt = att.toString();
95 int status = GuiInputAttribute::GetAttributes(curAtt, newAtt,
98 if((status == 1) && (curAtt != newAtt)) {
100 p_lineEdit->setText(f.
expanded() + newAtt);
108 QString curAtt = att.toString();
110 int status = GuiOutputAttribute::GetAttributes(curAtt, newAtt,
114 if((status == 1) && (curAtt != newAtt)) {
116 p_lineEdit->setText(f.
expanded() + newAtt);
131 QString cubeName =
Value();
139 QString command =
"$ISISROOT/bin/qview " + cubeName +
" &";
144 QString msg =
"You must enter a cube name to open";
161 QString cubeName =
Value();
176 QString msg =
"You must enter a cube name to open";
static void GuiLog(const Pvl &results)
Writes the Pvl results to the sessionlog, but not to the printfile.
void SelectAttribute()
Select cube attributes.
static void RunSystemCommand(QString commandLine)
This runs arbitrary system commands.
File name manipulation and expansion.
void ViewCube()
Opens cube in qview.
void close(bool remove=false)
Closes the cube and updates the labels.
Container for cube-like labels.
Manipulate and parse attributes of output cube filenames.
virtual bool IsModified()
Return if the parameter value is different from the default value.
void addAttributes(const FileName &fileNameWithAtts)
Append the attributes found in the filename to these cube attributes.
QString Value()
Gets the value found in the line edit text box.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
void GuiReportError(IException &e)
Loads the error message into the gui, but does not write it to the session log.
QString ParamFileMode(const int &group, const int ¶m) const
Returns the file mode for a parameter in a specified group.
virtual void SelectFile()
Gets an input/output file from a GUI filechooser or typed in filename.
IO Handler for Isis Cubes.
QString PixelType(const int &group, const int ¶m) const
Returns the default pixel type from the XML.
GuiCubeParameter(QGridLayout *grid, UserInterface &ui, int group, int param)
Constructs GuiCubeParameter object.
Command Line and Xml loader, validation, and access.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
void ViewLabel()
Displays cube label in the GUI log.
void open(const QString &cfile, QString access="r")
This method will open an isis cube for reading or reading/writing.
bool propagatePixelType() const
Return true if the pixel type is to be propagated from an input cube.
QString ParamName(const int &group, const int ¶m) const
Returns the parameter name.
~GuiCubeParameter()
Destructor of GuiCubeParameter object.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....