File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
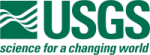 |
Isis 3 Programmer Reference
|
Go to the documentation of this file.
26 #include <QFileDialog>
28 #include <QMessageBox>
29 #include <QtConcurrentMap>
32 #include "ControlList.h"
33 #include "ControlNet.h"
34 #include "IException.h"
37 #include "ProjectItem.h"
38 #include "ProjectItemModel.h"
56 QAction::setText(tr(
"Import &Control Networks..."));
110 return (item->text() ==
"Control Networks");
127 QUndoCommand::setText(tr(
"Import Control Networks"));
131 QStringList cnetFileNames = QFileDialog::getOpenFileNames(
132 qobject_cast<QWidget *>(parent()),
133 tr(
"Import Control Networks"),
"",
134 tr(
"Isis control nets (*.net);;All Files (*)"));
136 if (!cnetFileNames.isEmpty()) {
137 QUndoCommand::setText(tr(
"Import %1 Control Networks").arg(cnetFileNames.count()));
157 foreach (
FileName fileName, cnetFileNames) {
159 cnetFileNamesAndProgress.append(qMakePair(fileName, readProgress));
165 m_watcher->setFuture(QtConcurrent::mapped(cnetFileNamesAndProgress,
170 int totalProgress = 0;
175 if (
m_watcher->future().isResultReadyAt(i)) {
176 totalProgress += 100;
181 totalProgress += qRound(progressPercent * 90);
189 QThread::yieldCurrentThread();
260 QString cnetFileName = cnetFileNameAndProgress.first.original();
263 cnet->
ReadControl(cnetFileName, cnetFileNameAndProgress.second);
266 QString destination = m_destinationFolder.canonicalPath() +
"/" + baseFilename;
268 cnet->
Write(destination);
290 QMutexLocker locker(
project()->workOrderMutex());
virtual bool setupExecution()
This sets up the state for the work order.
ControlList * m_list
List of controls added to project.
Control * operator()(const QPair< FileName, Progress * > &cnetFilename)
Reads and writes the control network(s) asynchronously.
void SetMutex(QMutex *mutex)
Set mutex to lock for making Naif calls.
This is free and unencumbered software released into the public domain.
Provide Undo/redo abilities, serialization, and history for an operation.
QFutureWatcher< Control * > * m_watcher
QFutureWatcher, allows for asynchronous import.
QString name() const
Returns the name of the file excluding the path and the attributes in the file name.
File name manipulation and expansion.
void addControl(Control *control)
Add the given Control's to the current project.
Project * m_project
The project to import to.
void setInternalData(QStringList data)
Sets the internal data for this WorkOrder.
void Write(const QString &filename, bool pvl=false)
Writes out the control network.
QString m_warning
String of any errors/warnings that occurred during import.
Control * activeControl()
Return the Active Control (control network)
virtual ImportControlNetWorkOrder * clone() const
This method clones the current ImportControlNetWorkOrder and returns it.
bool setupExecution()
Sets up the work order for execution.
This represents an ISIS control net in a project-based GUI interface.
void postExecution()
Clears progress.
ImportControlNetWorkOrder(Project *project)
Creates a work order to import a control network.
IException errors() const
Indicates if any errors occurred during the import.
bool m_isSynchronous
This is defaulted to true.
void ReadControl(const QString &filename, Progress *progress=0)
Reads in the control points from the given file.
The main project for ipce.
void append(const IException &exceptionSource)
Appends the given exception (and its list of previous exceptions) to this exception's causational exc...
QDir m_destinationFolder
The directory to copy the control net too.
~ImportControlNetWorkOrder()
Destructor.
void closeControlNet()
Cleans up the ControlNet pointer.
QList< Progress * > m_readProgresses
Keeps track of import progress.
bool m_isUndoable
Set the workorder to be undoable/redoable This is defaulted to true - his will allow the workorder to...
QString toString() const
Returns a string representation of this exception.
Project * project() const
Returns the Project this WorkOrder is attached to.
Add control networks to a project c Asks the user for a list of control nets and copies them into the...
Program progress reporter.
void setProgressRange(int, int)
Sets the progress range of the WorkOrder.
QList< ControlList * > controls()
Return controls in project.
QPointer< Project > m_project
A pointer to the Project this WorkOrder is attached to.
int MaximumSteps() const
Returns the maximum number of steps of the progress.
This is free and unencumbered software released into the public domain.
@ WorkOrderFinished
This is used for work orders that will not undo or redo (See createsCleanState())
void setProgressValue(int)
Sets the current progress value for the WorkOrder.
This is free and unencumbered software released into the public domain.
QStringList internalData() const
Gets the internal data for this WorkOrder.
void setModifiesDiskState(bool changesProjectOnDisk)
virtual bool isExecutable(ProjectItem *item)
This method returns true if the user clicked on a project tree node with the text "Control Networks".
void DisableAutomaticDisplay()
Turns off updating the Isis Gui when CheckStatus() is called.
CreateControlsFunctor(Project *project, QDir destinationFolder)
CreateControlsFunctor constructor.
QDir addCnetFolder(QString prefix)
Create and return the name of a folder for placing control networks.
void cnetReady(int ready)
Adds the control net to the project.
int CurrentStep() const
Returns the current step of the progress.
void setClean(bool value)
Function to change the clean state of the project.
void execute()
Imports the control network asynchronously.
This is free and unencumbered software released into the public domain.
Represents an item of a ProjectItemModel in Qt's model-view framework.