File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
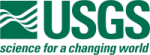 |
Isis 3 Programmer Reference
|
1 #ifndef JigsawRunWidget_h
2 #define JigsawRunWidget_h
12 #include "BundleSettings.h"
13 #include "IException.h"
117 QString outputControlFileName,
139 QString m_selectedControlName;
140 QString m_outputControlName;
156 public std::unary_function<const FileName &, Cube *> {
167 void on_JigsawSetupButton_clicked();
168 void on_JigsawRunButton_clicked();
An image bundle adjustment object.
File name manipulation and expansion.
This represents an ISIS control net in a project-based GUI interface.
The main project for ipce.
Container class for BundleAdjustment results.
IO Handler for Isis Cubes.
This is free and unencumbered software released into the public domain.