File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
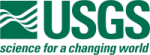 |
Isis 3 Programmer Reference
|
22 #include "BundleControlPoint.h"
23 #include "BundleObservationSolveSettings.h"
24 #include "BundleObservationVector.h"
25 #include "BundleResults.h"
26 #include "BundleSettings.h"
27 #include "BundleSolutionInfo.h"
28 #include "BundleTargetBody.h"
30 #include "CameraGroundMap.h"
31 #include "ControlMeasure.h"
32 #include "ControlNet.h"
33 #include "LinearAlgebra.h"
34 #include "MaximumLikelihoodWFunctions.h"
35 #include "ObservationNumberList.h"
37 #include "SerialNumberList.h"
38 #include "SparseBlockMatrix.h"
39 #include "Statistics.h"
41 template<
typename T >
class QList;
42 template<
typename A,
typename B >
class QMap;
322 const QString &cnetFile,
323 const QString &cubeList,
324 bool printSummary =
true);
328 bool printSummary =
true);
336 bool printSummary =
true);
339 const QString &cubeList,
340 bool printSummary =
true);
370 void statusUpdate(QString);
372 void iterationUpdate(
int);
373 void pointUpdate(
int);
374 void statusBarUpdate(QString);
404 double, boost::numeric::ublas::upper > &N22,
406 boost::numeric::ublas::compressed_vector< double > &n1,
412 int observationIndex);
414 double, boost::numeric::ublas::upper > &N22,
430 bool invert3x3(boost::numeric::ublas::symmetric_matrix<
431 double, boost::numeric::ublas::upper > &m);
433 double, boost::numeric::ublas::upper > &N22,
436 void productAlphaAV(
double alpha,
437 boost::numeric::ublas::bounded_vector< double, 3 > &v2,
470 QString m_iterationSummary;
491 boost::numeric::ublas::symmetric_matrix<
493 boost::numeric::ublas::upper,
495 cholmod_common m_cholmodCommon;
int m_numberOfImagePartials
number of image-related partials.
QString fileName(int index)
Return the ith filename in the cube list file given to constructor.
double iteration() const
Returns what iteration the BundleAdjust is currently on.
SerialNumberList * serialNumberList()
Returns a pointer to the serial number list.
bool m_abort
!< Contains information about the target body.
An image bundle adjustment object.
double computeResiduals()
This method computes the focal plane residuals for the measures.
bool wrapUp()
Compute the residuals for each adjusted point and store adjustment results in m_bundleResults.
boost::numeric::ublas::symmetric_matrix< double, boost::numeric::ublas::upper, boost::numeric::ublas::column_major > m_normalInverse
!< The lists of images used in the bundle.
This class is used to accumulate statistics on double arrays.
cholmod_factor * m_L
!< The CHOLMOD sparse normal equations matrix used by cholmod_factorize to solve the system.
This is free and unencumbered software released into the public domain.
bool invert3x3(boost::numeric::ublas::symmetric_matrix< double, boost::numeric::ublas::upper > &m)
Dedicated quick inverse of 3x3 matrix.
BundleSolutionInfo * bundleSolveInformation()
Create a BundleSolutionInfo containing the settings and results from the bundle adjustment.
bool freeCHOLMODLibraryVariables()
Free CHOLMOD library variables.
bool isConverged()
Returns if the BundleAdjust converged.
bool flagOutliers()
Flags outlier measures and control points.
bool formWeightedNormals(boost::numeric::ublas::compressed_vector< double > &n1, LinearAlgebra::Vector &nj)
Apply weighting for spacecraft position, velocity, acceleration and camera angles,...
void abortBundle()
Flag to abort when bundle is threaded.
SparseBlockMatrix m_sparseNormals
!< The right hand side of the normal equations.
void init(Progress *progress=0)
Initialize all solution parameters.
Table spVector(int index)
Return the updated instrument position table for the ith cube in the cube list given to the construct...
This class holds information about a control point that BundleAdjust needs to run correctly.
This represents an ISIS control net in a project-based GUI interface.
bool cholmodInverse()
Compute inverse of normal equations matrix for CHOLMOD.
QString modelState(int index)
Return the updated model state for the ith cube in the cube list given to the constructor.
boost::numeric::ublas::matrix< double > Matrix
Definition for an Isis::LinearAlgebra::Matrix of doubles.
Serial Number list generator.
ControlNetQsp controlNet()
Returns a pointer to the output control network.
LinearAlgebra::Vector m_imageSolution
!< The lower triangular L matrix from Cholesky decomposition.
Statistics m_xyResiduals
xy residual statistics.
bool solveCholesky()
Compute the least squares bundle adjustment solution using Cholesky decomposition.
bool solveSystem()
Compute the solution to the normal equations using the CHOLMOD library.
Internalizes a list of images and allows for operations on the entire list.
bool validateNetwork()
control network validation - on the very real chance that the net has not been checked before running...
SerialNumberList * m_serialNumberList
!< Vector of observations.
bool isAborted()
Returns if the BundleAdjust has been aborted.
BundleSettingsQsp m_bundleSettings
Contains the solve settings.
cholmod_triplet * m_cholmodTriplet
!< The sparse block normal equations matrix.
boost::numeric::ublas::vector< double > Vector
Definition for an Isis::LinearAlgebra::Vector of doubles.
void applyParameterCorrections()
Apply parameter corrections for solution.
bool productATransB(boost::numeric::ublas::symmetric_matrix< double, boost::numeric::ublas::upper > &N22, SparseBlockColumnMatrix &N12, SparseBlockRowMatrix &Q)
Perform the matrix multiplication Q = N22 x N12(transpose)
bool initializeNormalEquationsMatrix()
Initialize Normal Equations matrix (m_sparseNormals).
bool loadCholmodTriplet()
Load sparse normal equations matrix into CHOLMOD triplet.
BundleResults m_bundleResults
Stores the results of the bundle adjust.
Table cMatrix(int index)
Return the updated instrument pointing table for the ith cube in the cube list given to the construct...
BundleAdjust(BundleSettingsQsp bundleSettings, const QString &cnetFile, const QString &cubeList, bool printSummary=true)
Construct a BundleAdjust object from the given settings, control network file, and cube list.
Container class for BundleAdjustment results.
Class for storing Table blobs information.
bool formMeasureNormals(boost::numeric::ublas::symmetric_matrix< double, boost::numeric::ublas::upper > &N22, SparseBlockColumnMatrix &N12, boost::numeric::ublas::compressed_vector< double > &n1, LinearAlgebra::Vector &n2, LinearAlgebra::Matrix &coeffTarget, LinearAlgebra::Matrix &coeffImage, LinearAlgebra::Matrix &coeffPoint3D, LinearAlgebra::Vector &coeffRHS, int observationIndex)
Form the auxilary normal equation matrices for a measure.
Statistics m_yResiduals
y residual statistics.
bool m_cleanUp
!< If the iteration summaries should be output to the log file.
~BundleAdjust()
Destroys BundleAdjust object, deallocates pointers (if we have ownership), and frees variables from c...
A container class for a ControlMeasure.
LinearAlgebra::Vector m_RHS
!< Contains object parameters, statistics, and workspace used by the CHOLMOD library.
int m_rank
!< If the serial number lists need to be deleted by the destructor.
Program progress reporter.
int numberOfImages() const
Returns the number of images.
QString m_cnetFileName
The control net filename.
void accumProductAlphaAB(double alpha, SparseBlockRowMatrix &A, LinearAlgebra::Vector &B, LinearAlgebra::Vector &C)
Performs the matrix multiplication nj = nj + alpha (Q x n2).
BundleObservationVector m_bundleObservations
!< Vector of control points.
void productAB(SparseBlockColumnMatrix &A, SparseBlockRowMatrix &B)
Perform the matrix multiplication C = N12 x Q.
BundleSolutionInfo * solveCholeskyBR()
Compute the least squares bundle adjustment solution using Cholesky decomposition.
Statistics m_xResiduals
x residual statistics.
bool formPointNormals(boost::numeric::ublas::symmetric_matrix< double, boost::numeric::ublas::upper > &N22, SparseBlockColumnMatrix &N12, LinearAlgebra::Vector &n2, LinearAlgebra::Vector &nj, BundleControlPointQsp &point)
Compute the Q matrix and NIC vector for a control point.
bool computeRejectionLimit()
Compute rejection limit.
bool formNormalEquations()
Form the least-squares normal equations matrix via cholmod.
ControlNetQsp m_controlNet
Output control net.
QString iterationSummaryGroup() const
Returns the iteration summary string.
void iterationSummary()
Creates an iteration summary and an iteration group for the solution summary.
int m_iteration
The current iteration.
cholmod_sparse * m_cholmodNormal
!< The CHOLMOD triplet representation of the sparse normal equations matrix.
bool computeBundleStatistics()
Compute Bundle statistics and store them in m_bundleResults.
This is free and unencumbered software released into the public domain.
void outputBundleStatus(QString status)
Slot for deltack and jigsaw to output the bundle status.
bool errorPropagation()
Error propagation for solution.
QList< ImageList * > imageLists()
This method returns the image list used in the bundle adjust.
bool initializeCHOLMODLibraryVariables()
Initializations for CHOLMOD sparse matrix package.
A container class for statistical results from a BundleAdjust solution.
This is free and unencumbered software released into the public domain.
bool computePartials(LinearAlgebra::Matrix &coeffTarget, LinearAlgebra::Matrix &coeffImage, LinearAlgebra::Matrix &coeffPoint3D, LinearAlgebra::Vector &coeffRHS, BundleMeasure &measure, BundleControlPoint &point)
Compute partial derivatives and weighted residuals for a measure.
bool m_printSummary
!< Summary of the most recently completed iteration.
int m_previousNumberImagePartials
!< The image parameter solution vector.
This is free and unencumbered software released into the public domain.
This class is a container class for BundleObservations.