Loading [MathJax]/jax/output/NativeMML/config.js
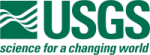 |
Isis 3 Programmer Reference
|
9 #include "LoMediumDistortionMap.h"
14 #include "CameraFocalPlaneMap.h"
32 LoMediumDistortionMap::LoMediumDistortionMap(
Camera *parent) :
79 QString centkey =
"INS" +
toString(naifIkCode) +
"_POINT_OF_SYMMETRY";
117 if(fabs(dx) > 45.79142767 || fabs(dy) > 35.09)
return false;
130 double origr2 = dists * dists + distl * distl;
131 double sp = sqrt(origr2);
140 double nS = sp / sRef;
141 double dS =
p_odk[0] * nS +
p_odk[1] * pow(nS, 3) +
p_odk[2] * pow(nS, 5);
142 double prevdS = 2 * dS;
143 double pixtol = .000001;
148 while(fabs(dS - prevdS) > pixtol) {
150 if(numit > 14 || fabs(dS) > 1E9) {
165 double ratio = s / sp;
166 double undistortedSample = dists * ratio +
p_sample0;
167 double undistortedLine = distl * ratio +
p_line0;
200 if(fabs(ux) > 45.79142767 || fabs(uy) > 35.09)
return false;
201 if(fabs(ux) > 41.85 || fabs(uy) > 35.09)
return false;
218 double rp2 = (distus * distus + distul * distul);
227 rp2 = rp2 / sRef / sRef;
228 double drOverR = (
p_odk[0] + rp2 *
p_odk[1] + rp2 * rp2 *
p_odk[2]) / sRef;
231 double ds =
p_sample0 + (distus * (1. + drOverR));
232 double dl =
p_line0 + (distul * (1. + drOverR));
void SetDistortion(const int naifIkCode)
Load LO Medium Resolution Camera perspective & distortion coefficients.
virtual void SetDistortion(int naifIkCode)
Load distortion coefficients.
double FocalPlaneY() const
virtual bool SetUndistortedFocalPlane(const double ux, const double uy)
Compute distorted focal plane x/y for Lo Medium Resolution Camera.
double p_focalPlaneX
Distorted focal plane x.
double p_undistortedFocalPlaneY
Undistorted focal plane y.
double p_line0
Center of distortion on line axis.
double p_undistortedFocalPlaneX
Undistorted focal plane x.
double DetectorLine() const
double DetectorSample() const
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
virtual bool SetFocalPlane(const double dx, const double dy)
Compute undistorted focal plane x/y for Lo Medium Resolution Camera.
virtual bool SetFocalPlane(const double dx, const double dy)
Compute detector position (sample,line) from focal plane coordinates.
Camera * p_camera
The camera to distort/undistort.
Distort/undistort focal plane coordinates.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
Namespace for the standard library.
double FocalPlaneX() const
virtual bool SetDetector(const double sample, const double line)
Compute distorted focal plane coordinate from detector position (sampel,line)
const double E
Sets some basic constants for use in ISIS programming.
double p_sample0
Center of distortion on sample axis.
std::vector< double > p_odk
Vector of distortion coefficients.
double p_focalPlaneY
Distorted focal plane y.
This is free and unencumbered software released into the public domain.