File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
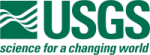 |
Isis 3 Programmer Reference
|
1 #include "MosaicGridTool.h"
3 #include <QApplication>
6 #include <QDoubleValidator>
7 #include <QGraphicsScene>
12 #include <QMessageBox>
14 #include <QPushButton>
20 #include "GridGraphicsItem.h"
21 #include "IException.h"
23 #include "Longitude.h"
24 #include "MosaicGraphicsView.h"
25 #include "MosaicGridToolConfigDialog.h"
26 #include "Projection.h"
27 #include "TProjection.h"
28 #include "MosaicSceneWidget.h"
29 #include "PvlObject.h"
62 connect(getWidget(), SIGNAL(projectionChanged(
Projection *)),
63 this, SLOT(onProjectionChanged()), Qt::UniqueConnection);
146 if (getWidget()->getProjection()) {
165 if (getWidget()->getProjection()) {
308 Projection *proj = getWidget()->getProjection();
317 QRectF boundingRect = getWidget()->cubesBoundingRect();
319 double topLeft = 100;
320 double topRight = 100;
321 double bottomLeft = 100;
322 double bottomRight = 100;
323 bool cubeRectWorked =
true;
333 if (tproj->
SetCoordinate(boundingRect.topLeft().x(), -boundingRect.topLeft().y())) {
337 cubeRectWorked =
false;
339 if (tproj->
SetCoordinate(boundingRect.topRight().x(), -boundingRect.topRight().y())) {
343 cubeRectWorked =
false;
345 if (tproj->
SetCoordinate(boundingRect.bottomLeft().x(), -boundingRect.bottomLeft().y())) {
349 cubeRectWorked =
false;
352 -boundingRect.bottomRight().y())) {
356 cubeRectWorked =
false;
359 if (cubeRectWorked) {
361 std::min(bottomLeft, bottomRight)), mappingGroup,
364 std::max(bottomLeft, bottomRight)), mappingGroup,
368 boundingRect.contains(QPointF(tproj->
XCoord(), -tproj->
YCoord()))) {
373 boundingRect.contains(QPointF(tproj->
XCoord(), -tproj->
YCoord()))) {
382 static Projection *lastProjWithThisError = NULL;
384 if (proj != lastProjWithThisError) {
385 lastProjWithThisError = proj;
386 QMessageBox::warning(NULL, tr(
"Latitude Extent Failure"),
387 tr(
"<p/>Could not extract latitude extents from the cubes.<br/>"
388 "<br/>The option <strong>\"Compute From Images\"</strong> "
389 "will default to using the <strong>Manual</strong> option "
390 "for latitude extents with a range of -90 to 90."));
432 Projection *proj = getWidget()->getProjection();
435 QRectF boundingRect = getWidget()->cubesBoundingRect();
439 double bottomLeft = 0;
440 double bottomRight = 0;
441 bool cubeRectWorked =
true;
451 if (tproj->
SetCoordinate(boundingRect.topLeft().x(), -boundingRect.topLeft().y())) {
456 cubeRectWorked =
false;
458 if (tproj->
SetCoordinate(boundingRect.topRight().x(), -boundingRect.topRight().y())) {
462 cubeRectWorked =
false;
464 if (tproj->
SetCoordinate(boundingRect.bottomLeft().x(), -boundingRect.bottomLeft().y())) {
468 cubeRectWorked =
false;
471 -boundingRect.bottomRight().y())) {
475 cubeRectWorked =
false;
478 if (cubeRectWorked) {
480 std::min(bottomLeft, bottomRight)),
483 std::max(bottomLeft, bottomRight)),
503 static Projection *lastProjWithThisError = NULL;
505 if (proj != lastProjWithThisError) {
506 lastProjWithThisError = proj;
507 QMessageBox::warning(NULL, tr(
"Longitude Extent Failure"),
508 tr(
"<p/>Could not extract longitude extents from the cubes.<br/>"
509 "<br/>The option <strong>\"Compute From Images\"</strong> "
510 "will default to using the <strong>Manual</strong> option "
511 "for longitude extents with a range of 0 to 360."));
561 Projection *proj = getWidget()->getProjection();
570 if (obj[
"BaseLatitude"][0] !=
"Null")
574 if (obj[
"BaseLongitude"][0] !=
"Null")
577 if (obj[
"LatitudeIncrement"][0] !=
"Null")
580 if (obj[
"LongitudeIncrement"][0] !=
"Null")
584 if (obj[
"LatitudeExtentType"][0] !=
"Null")
589 if (obj[
"MinimumLatitude"][0] !=
"Null")
595 if (obj[
"MaximumLatitude"][0] !=
"Null")
601 if (obj[
"LongitudeExtentType"][0] !=
"Null")
606 if (obj[
"MinimumLongitude"][0] !=
"Null")
611 if (obj[
"MaximumLongitude"][0] !=
"Null")
615 if (obj[
"Density"][0] !=
"Null")
620 if (obj[
"CheckTheBoxes"][0] !=
"Null") {
625 if(
toBool(obj[
"Visible"][0])) {
638 return "MosaicGridTool";
673 Longitude MosaicGridTool::domainMinLon() {
676 if (getWidget() && getWidget()->getProjection()) {
691 Longitude MosaicGridTool::domainMaxLon() {
694 if (getWidget() && getWidget()->getProjection()) {
696 TProjection *tproj = (TProjection *) getWidget()->getProjection();
697 if (tproj->Has360Domain()) {
718 FileName(QString(
"$HOME/.Isis/%1/mosaicSceneGridTool.config")
719 .arg(QApplication::applicationName())).expanded(),
720 QSettings::NativeFormat);
721 settings.setValue(
"autoGrid", draw);
723 Projection *proj = getWidget()->getProjection();
726 QRectF boundingRect = getWidget()->cubesBoundingRect();
728 if (!boundingRect.isNull()) {
735 if (tproj->
Mapping()[
"LatitudeType"][0] ==
"Planetographic") {
758 double latOffsetMultiplier = pow(10, qFloor(log10(latRange) - 1));
759 double lonOffsetMultiplier = pow(10, qFloor(log10(lonRange) - 1));
761 double idealLatInc = latRange / 10.0;
762 double idealLonInc = lonRange / 10.0;
764 double roundedLatInc = qRound(idealLatInc / latOffsetMultiplier) * latOffsetMultiplier ;
765 double roundedLonInc = qRound(idealLonInc / lonOffsetMultiplier) * lonOffsetMultiplier ;
782 if(m_gridItem != NULL) {
783 disconnect(getWidget(), SIGNAL(projectionChanged(
Projection *)),
786 getWidget()->getScene()->removeItem(m_gridItem);
800 qobject_cast<QWidget *>(parent()));
801 configDialog->setAttribute(Qt::WA_DeleteOnClose);
802 configDialog->show();
810 void MosaicGridTool::onProjectionChanged() {
836 if(m_gridItem != NULL) {
846 if (!getWidget()->getProjection()) {
847 QString msg =
"Please set the mosaic scene's projection before trying to "
848 "draw a grid. This means either open a cube (a projection "
849 "will be calculated) or set the projection explicitly";
850 QMessageBox::warning(NULL, tr(
"Grid Tool Requires Projection"), msg);
859 connect(getWidget(), SIGNAL(projectionChanged(
Projection *)),
860 this, SLOT(
drawGrid()), Qt::UniqueConnection);
862 connect(getWidget(), SIGNAL(cubesChanged()),
865 if (m_gridItem != NULL)
866 getWidget()->getScene()->addItem(m_gridItem);
899 emit boundingRectChanged();
903 getWidget()->getView()->update();
904 QApplication::processEvents();
918 FileName(QString(
"$HOME/.Isis/%1/mosaicSceneGridTool.config")
919 .arg(QApplication::applicationName())).expanded(),
920 QSettings::NativeFormat);
922 bool drawAuto = settings.value(
"autoGrid",
true).toBool();
962 m_action->setIcon(
getIcon(
"grid.png"));
963 m_action->setToolTip(
"Grid (g)");
964 m_action->setShortcut(Qt::Key_G);
966 "<b>Function:</b> Superimpose a map grid over the area of displayed "
967 "footprints in the 'mosaic scene.'<br><br>"
968 "This tool allows you to overlay a ground grid onto the mosaic scene. "
969 "The inputs are standard ground grid parameters and a grid density."
970 "<p><b>Shortcut:</b> g</p> ";
971 m_action->setWhatsThis(text);
985 QHBoxLayout *actionLayout =
new QHBoxLayout();
987 QString autoGridWhatsThis =
988 "Draws a grid based on the current lat/lon extents (from the cubes, map, or user).";
998 QPushButton *optionsButton =
new QPushButton(
"Grid Options");
999 optionsButton->setWhatsThis(
"Opens a dialog box that has the options to change the base"
1000 " latitude, base longitude, latitude increment, longitude"
1001 " increment, and grid density.");
1002 connect(optionsButton, SIGNAL(clicked()),
this, SLOT(
configure()));
1003 actionLayout->addWidget(optionsButton);
1005 QString drawGridWhatsThis =
1006 "Draws a grid based on the current lat/lon extents (from the cubes, map, or user).";
1007 QLabel *drawGridLabel =
new QLabel(
"Show Grid");
1008 drawGridLabel->setWhatsThis(drawGridWhatsThis);
1012 actionLayout->addWidget(drawGridLabel);
1015 connect(
this, SIGNAL(activated(
bool)),
this, SLOT(
onToolOpen(
bool)));
1017 actionLayout->addStretch(1);
1018 actionLayout->setMargin(0);
1021 toolBarWidget->setLayout(actionLayout);
1023 return toolBarWidget;
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
Contains Pvl Groups and Pvl Objects.
The visual display of the find point.
A single keyword-value pair.
This class is designed to encapsulate the concept of a Latitude.
double planetographic(Angle::Units units=Angle::Radians) const
Get the latitude in the planetographic coordinate system.
File name manipulation and expansion.
virtual bool SetCoordinate(const double x, const double y)
This method is used to set the projection x/y.
virtual double MinimumLongitude() const
This returns the minimum longitude of the area of interest.
virtual double Longitude() const
This returns a longitude with correct longitude direction and domain as specified in the label object...
ProjectionType projectionType() const
Returns an enum value for the projection type.
virtual double MaximumLatitude() const
This returns the maximum latitude of the area of interest.
double EquatorialRadius() const
This returns the equatorial radius of the target.
bool Has360Domain() const
This indicates if the longitude domain is 0 to 360 (as opposed to -180 to 180).
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
QString LongitudeDomainString() const
This method returns the longitude domain as a string.
QString LatitudeTypeString() const
This method returns the latitude type as a string.
virtual bool SetUniversalGround(const double lat, const double lon)
This method is used to set the latitude/longitude which must be Planetocentric (latitude) and Positiv...
@ Meters
The distance is being specified in meters.
Contains multiple PvlContainers.
double PolarRadius() const
This returns the polar radius of the target.
virtual double Latitude() const
This returns a latitude with correct latitude type as specified in the label object.
int toInt(const QString &string)
Global function to convert from a string to an integer.
@ Planetocentric
This is the universal (and default) latitude coordinate system.
Base class for Map TProjections.
bool hasKeyword(const QString &kname, FindOptions opts) const
See if a keyword is in the current PvlObject, or deeper inside other PvlObjects and Pvlgroups within ...
Defines an angle and provides unit conversions.
virtual double MaximumLongitude() const
This returns the maximum longitude of the area of interest.
double toDouble(const QString &string)
Global function to convert from a string to a double.
bool toBool(const QString &string)
Global function to convert from a string to a boolean.
double degrees() const
Get the angle in units of Degrees.
virtual double MinimumLatitude() const
This returns the minimum latitude of the area of interest.
@ Triaxial
These projections are used to map triaxial and irregular-shaped bodies.
virtual PvlGroup Mapping()
This function returns the keywords that this projection uses.
double YCoord() const
This returns the projection Y provided SetGround, SetCoordinate, SetUniversalGround,...
Base class for Map Projections.
double XCoord() const
This returns the projection X provided SetGround, SetCoordinate, SetUniversalGround,...
This is free and unencumbered software released into the public domain.