Loading [MathJax]/jax/output/NativeMML/config.js
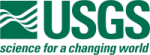 |
Isis 3 Programmer Reference
|
16 #include "NaifDskPlateModel.h"
23 #if defined(MAKE_THREAD_SAFE)
24 #include <QMutexLocker>
26 #include <QScopedPointer>
29 #include "IException.h"
30 #include "Intercept.h"
33 #include "Longitude.h"
34 #include "NaifDskApi.h"
35 #include "NaifStatus.h"
36 #include "SurfacePoint.h"
37 #include "TriangularPlate.h"
44 NaifDskPlateModel::NaifDskPlateModel() : m_dsk(0) { }
52 QString mess =
"Could not open DSK file " + dskfile;
59 NaifDskPlateModel::~NaifDskPlateModel() { }
65 return ( !
m_dsk.isNull() );
88 return (
m_dsk->m_plates );
96 return (
m_dsk->m_vertices );
125 verify ( lat.
isValid(),
"Latitude parameter invalid in NaifDskPlateMode::point()" );
126 verify ( lon.
isValid(),
"Longitude parameter invalid in NaifDskPlateMode::point()" );
132 SpiceDouble lonlat[2];
137 SpiceInt plateId(-1);
139 #if defined(MAKE_THREAD_SAFE)
140 QMutexLocker lock(&
m_dsk->m_mutex);
143 llgrid_pl02(
m_dsk->m_handle, &
m_dsk->m_dladsc, npoints,
144 (ConstSpiceDouble (*)[2]) lonlat,
145 (SpiceDouble (*)[3]) &spoint[0], &plateId);
150 QString mess =
"Plateid = " + QString::number(plateId) +
" is invalid";
214 if ( !
isValid() )
return (
false);
215 return ( (plateid >= 1) && (plateid <= m_dsk->m_plates) );
242 verify(
validate(vertex),
"Invalid/bad dimensions on intercept source point");
243 verify(
validate(raydir),
"Invalid/bad dimensions on ray direction vector");
247 "Invalid point source data to determine intercept",
259 #if defined(MAKE_THREAD_SAFE)
260 QMutexLocker lock(&
m_dsk->m_mutex);
264 dskx02_c(
m_dsk->m_handle, &
m_dsk->m_dladsc, &vertex[0], &raydir[0],
265 &plateid, &xpt[0], &found);
268 if ( !found )
return (0);
297 QString mess =
"Plateid = " + QString::number(plateid) +
" is invalid";
305 #if defined(MAKE_THREAD_SAFE)
306 QMutexLocker lock(&
m_dsk->m_mutex);
310 dskp02_c(
m_dsk->m_handle, &
m_dsk->m_dladsc, plateid, 1, &nplates,
311 ( SpiceInt(*)[3] )(iplate));
317 for (
int i = 0 ; i < 3 ; i++) {
318 dskv02_c(
m_dsk->m_handle, &
m_dsk->m_dladsc, iplate[i], 1, &n,
319 ( SpiceDouble(*)[3] )(
plate[i]));
350 QString mess =
"NAIF DSK file [" + dskfile +
"] does not exist.";
356 dsk->m_dskfile = dskfile;
358 dasopr_c( dskFile.
expanded().toLatin1().data(), &dsk->m_handle );
363 dlabfs_c( dsk->m_handle, &dsk->m_dladsc, &found );
366 QString mess =
"No segments found in DSK file " + dskfile ;
371 dskgd_c( dsk->m_handle, &dsk->m_dladsc, &dsk->m_dskdsc );
374 dskz02_c( dsk->m_handle, &dsk->m_dladsc, &dsk->m_vertices,
379 return ( dsk.take() );
388 if ( (
Throw == action ) && ( !test ) ) {
408 NaifDskPlateModel::NaifDskDescriptor::NaifDskDescriptor() : m_dskfile(), m_handle(-1),
409 m_dladsc(), m_dskdsc(), m_plates(0),
410 m_vertices(0), m_mutex() {
414 NaifDskPlateModel::NaifDskDescriptor::~NaifDskDescriptor() {
bool verify(const bool &test, const QString &errmsg, const ErrAction &action=Throw) const
Convenience method for generalized error reporting.
TNT::Array1D< SpiceDouble > NaifVertex
1-D Buffer[3]
This class is designed to encapsulate the concept of a Latitude.
NAIF DSK file descriptor.
double positiveEast(Angle::Units units=Angle::Radians) const
Get the longitude in the PositiveEast coordinate system.
NaifTriangle plate(SpiceInt plateid) const
Retrieve the triangular plate identified by its ID.
File name manipulation and expansion.
bool fileExists() const
Returns true if the file exists; false otherwise.
SpiceInt numberVertices() const
Returns the number of vertices in the plate model.
SpiceInt plateIdOfIntercept(const NaifVertex &vertex, const NaifVector &raydir, NaifVertex &xpoint) const
Primary API to determine ray intercept from observer/look direction.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
int size() const
Returns the number of plates in the DSK file - mostly for conformity.
This class is designed to encapsulate the concept of a Longitude.
Displacement is a signed length, usually in meters.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
Intercept * intercept(const NaifVertex &vertex, const NaifVector &raydir) const
Determine a target body intercept point from an observer and look direction.
NaifDskPlateModel()
Default empty constructor.
QString filename() const
Returns the nane of the NAIF DSK file.
bool isValid() const
Checks validity of the object.
TNT::Array2D< SpiceDouble > NaifTriangle
3-D triangle[3][3]
NaifDskDescriptor * openDSK(const QString &dskfile)
Opens a valid NAIF DSK plate model file.
bool isPlateIdValid(const SpiceInt plateid) const
Determines if the plate ID is valid.
SharedNaifDskDescriptor m_dsk
Shared pointer to the NaifDskDescriptor for this plate.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
@ Throw
Throw an exception if an error occurs.
Container for a intercept condition.
double planetocentric(Angle::Units units=Angle::Radians) const
Get the latitude in the planetocentric (universal) coordinate system.
ErrAction
Enumeration to indicate whether to throw an exception if an error occurs.
SurfacePoint * makePoint(const NaifVertex &v) const
Construct and return a SurfacePoint pointer
TNT::Array1D< SpiceDouble > NaifVector
Namespace to contain type definitions of NAIF DSK fundamentals.
SpiceInt m_handle
The DAS file handle of the DSK file.
bool validate(const NaifVertex &v)
Verifies that the given NaifVector or NaifVertex is 3 dimensional.
@ Kilometers
The distance is being specified in kilometers.
SurfacePoint * point(const Latitude &lat, const Longitude &lon) const
Get surface intersection for a lat/lon grid point.
This class defines a body-fixed surface point.
Specification for an abstract triangular plate.
@ Radians
Radians are generally used in mathematical equations, 0-2*PI is one circle, however these are more di...
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
SpiceInt numberPlates() const
Returns the number of plates in the model.