File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
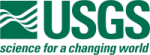 |
Isis 3 Programmer Reference
|
13 #include "NaifStatus.h"
14 #include "OsirisRexDistortionMap.h"
15 #include "CameraFocalPlaneMap.h"
75 if (filter.toUpper().compare(
"UNKNOWN") == 0) {
76 odkkey =
"INS" +
toString(naifIkCode) +
"_OD_K";
79 odkkey =
"INS" +
toString(naifIkCode) +
"_OD_K_" + filter.trimmed().toUpper();
83 for (
int i = 0; i < 5; ++i) {
97 if (filter.toUpper().compare(
"UNKNOWN") == 0) {
98 odcenterkey =
"INS" +
toString(naifIkCode) +
"_OD_CENTER";
101 odcenterkey =
"INS" +
toString(naifIkCode) +
"_OD_CENTER_" + filter.trimmed().toUpper();
125 if (
p_odk.size() < 2) {
137 double xx, yy, r, rr, rrr, rrrr;
138 double xdistortion, ydistortion;
139 double xdistorted, ydistorted;
140 double xprevious, yprevious;
143 xprevious = 1000000.0;
144 yprevious = 1000000.0;
146 double tolerance = 0.000001;
148 bool bConverged =
false;
153 for(
int i = 0; i < 50; i++) {
154 xx = (xt-x0) * (xt-x0);
155 yy = (yt-y0) * (yt-y0);
164 xdistortion = drOverR * (xt-x0);
165 ydistortion = drOverR * (yt-y0);
168 xt = dx - xdistortion;
169 yt = dy - ydistortion;
175 if((fabs(xt - xprevious) <= tolerance) && (fabs(yt - yprevious) <= tolerance)) {
212 if (
p_odk.size() < 2) {
224 double r = qSqrt(((x-x0) * (x-x0)) + ((y - y0) * (y-y0)));
double m_distortionOriginLine
The distortion's origin line coordinate.
double p_focalPlaneX
Distorted focal plane x.
virtual void SetDistortion(int naifIkCode, QString filterName)
Load distortion coefficients and center-of-distortion for OCAMS.
double p_undistortedFocalPlaneY
Undistorted focal plane y.
double p_undistortedFocalPlaneX
Undistorted focal plane x.
double m_detectorOriginSample
The origin of the detector's sample coordinate.
double m_detectorOriginLine
The origin of the detector's line coordinate.
double DetectorSampleOrigin() const
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
double DetectorLineOrigin() const
double m_distortionOriginSample
The distortion's origin sample coordinate.
Camera * p_camera
The camera to distort/undistort.
Distort/undistort focal plane coordinates.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
double PixelPitch() const
Returns the pixel pitch.
virtual ~OsirisRexDistortionMap()
Default Destructor.
const double E
Sets some basic constants for use in ISIS programming.
double m_pixelPitch
The pixel pitch for OCAMS.
OsirisRexDistortionMap(Camera *parent, double zDirection=1.0)
OSIRIS REx Camera distortion map constructor.
std::vector< double > p_odk
Vector of distortion coefficients.
virtual bool SetUndistortedFocalPlane(double ux, double uy)
Compute distorted focal plane x/y.
virtual bool SetFocalPlane(double dx, double dy)
Compute undistorted focal plane x/y.
double p_focalPlaneY
Distorted focal plane y.
This is free and unencumbered software released into the public domain.