File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
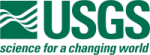 |
Isis 3 Programmer Reference
|
11 #include "ProjectItemProxyModel.h"
14 #include <QItemSelection>
17 #include <QModelIndex>
21 #include "ProjectItem.h"
22 #include "ProjectItemModel.h"
49 return proxyItem->index();
70 return sourceItem->index();
88 const QItemSelection &sourceSelection) {
90 QItemSelection proxySelection = QItemSelection();
92 foreach ( QModelIndex sourceIndex, sourceSelection.indexes() ) {
94 if ( proxyIndex.isValid() ) {
95 proxySelection.select(proxyIndex, proxyIndex);
99 return proxySelection;
113 QItemSelection sourceSelection = QItemSelection();
115 foreach ( QModelIndex proxyIndex, proxySelection.indexes() ) {
117 if ( sourceIndex.isValid() ) {
118 sourceSelection.select(sourceIndex, sourceIndex);
122 return sourceSelection;
135 return m_sourceProxyMap.value(sourceItem, 0);
148 return m_sourceProxyMap.key(proxyItem, 0);
175 proxyItem =
addChild(sourceItem, parentItem);
178 proxyItem =
addChild(sourceItem, 0);
181 for (
int i=0; i < sourceItem->rowCount(); i++) {
238 SIGNAL( currentChanged(
const QModelIndex &,
const QModelIndex &) ),
242 SIGNAL( currentChanged(
const QModelIndex &,
const QModelIndex &) ),
246 SIGNAL( selectionChanged(
const QItemSelection &,
const QItemSelection &) ),
250 SIGNAL( selectionChanged(
const QItemSelection &,
const QItemSelection &) ),
280 proxyItem->setProjectItem(sourceItem);
293 selectionModel()->setCurrentIndex(newProxyCurrent, QItemSelectionModel::Current);
306 QItemSelectionModel::Current);
319 selectionModel()->select(newProxySelection, QItemSelectionModel::ClearAndSelect);
333 QItemSelectionModel::ClearAndSelect);
364 if ( parentItem && (parentItem->
model() !=
this) ) {
373 m_sourceProxyMap.insert(sourceItem, proxyItem);
377 oldParent->takeRow( proxyItem->row() );
380 model->takeRow( proxyItem->row() );
419 Qt::DropAction action,
421 const QModelIndex &parent)
const {
438 Qt::DropAction action,
440 const QModelIndex &parent) {
441 if ( data->hasFormat(
"application/x-qabstractitemmodeldatalist") ) {
Provides access to data stored in a Project through Qt's model-view framework.
ProjectItemModel * m_sourceModel
The source model. Map of items from the source model to the proxy model.
QItemSelection mapSelectionFromSource(const QItemSelection &sourceSelection)
Returns a QItemSelection of items in the proxy model that corresponds with a QItemSelection of items ...
virtual bool dropMimeData(const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent)
Adds the data (selected items) from the source model to the proxy model.
void updateProxyCurrent()
Slot that updates the current item in the proxy model only if it is different than the corresponding ...
void addItems(QList< ProjectItem * > sourceItems)
Adds a list of items to the proxy model.
This is free and unencumbered software released into the public domain.
QModelIndex mapIndexToSource(const QModelIndex &proxyIndex)
Returns the QModelIndex of an item in the souce model that corresponds with the QModelIndex of an ite...
ProjectItem * addChild(ProjectItem *sourceItem, ProjectItem *parentItem)
Creates an item in the proxy model corresponding to an item in the source model as a child of a paren...
void appendRow(ProjectItem *item)
Appends a top-level item to the model.
void updateSourceCurrent()
Slot that updates the current item in the proxy model only if it is different than the corresponding ...
ProjectItemModel * sourceModel()
Returns the source model.
QItemSelectionModel * selectionModel()
Returns the internal selection model.
ProjectItem * child(int row) const
Returns the child item at a given row.
void onItemChanged(QStandardItem *item)
Signal to connect to the itemChanged() signal from a ProjectItemModel.
ProjectItem * mapItemToSource(ProjectItem *proxyItem)
Returns the ProjectItem in the source model that corresponds with a ProjectItem in the source model.
QItemSelection mapSelectionToSource(const QItemSelection &proxySelection)
Returns a QItemSelection of items in the source model that corresponds with a QItemSelection of itesm...
ProjectItem * item(int row)
Returns the top-level item at the given row.
void removeItem(ProjectItem *item)
Removes an item and its children from the proxy model.
ProjectItem * itemFromIndex(const QModelIndex &index)
Returns the ProjectItem corresponding to a given QModelIndex.
QModelIndex mapIndexFromSource(const QModelIndex &sourceIndex)
Returns the QModelIndex of an item in the proxy model that corresponds with the QModelIndex of an ite...
void updateProxySelection()
Slot that updates the selection in the proxy model only if it is different than the corresponding sel...
ProjectItem * mapItemFromSource(ProjectItem *sourceItem)
Returns the ProjectItem in the proxy model that corresponds with a ProjectItem in the source model.
QList< ProjectItem * > selectedItems()
Returns a list of the selected items of the internal selection model.
void appendRow(ProjectItem *item)
Appends an item to the children of this item.
void setSourceModel(ProjectItemModel *sourceModel)
Sets the source model.
void updateSourceSelection()
Slot that updates the selection in the source model only if it is different than the corresponding se...
ProjectItem * addItem(ProjectItem *sourceItem)
Adds an item and its children to the proxy model.
void updateItem(ProjectItem *sourceItem)
Given an item in the source model, this method changes the data of the corresponding item in the prox...
virtual bool canDropMimeData(const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const
Returns true.
ProjectItem * parent() const
Returns the parent item of this item.
This is free and unencumbered software released into the public domain.
ProjectItemModel * model() const
Returns the ProjectItemModel associated with this item.
void setProjectItem(ProjectItem *item)
Sets the text, icon, and data to those of another item.
ProjectItemProxyModel(QObject *parent=0)
Constructs the proxy model.
virtual void removeItem(ProjectItem *item)
Removes an item and its children from the model.
This is free and unencumbered software released into the public domain.
Represents an item of a ProjectItemModel in Qt's model-view framework.