File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
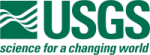 |
Isis 3 Programmer Reference
|
7 #include "PushFrameCameraDetectorMap.h"
13 #include "CameraFocalPlaneMap.h"
29 const double frameletRate,
int frameletHeight):
63 double unsummedFrameletLine;
73 double unsummedFrameletSample = sample;
136 const double deltaT) {
144 int framelet = (int)((line - 0.5) / actualFrameletHeight) + 1;
441 const int band)
const {
void SetFrameletOffset(int frameletOffset)
Reset the frame offset.
bool p_flippedFramelets
Indicates whether the geometry in a framelet is flipped.
double p_parentLine
The parent line calculated from the detector.
int p_frameletOffset
The numner of framelets padding the top of the band.
Convert between parent image coordinates and detector coordinates.
double FrameletRate() const
Return the time in seconds between framelets.
int ParentLines() const
Returns the number of lines in the parent alphacube.
int TotalFramelets() const
Return the total number of framelets including padding.
void SetFrameletOrderReversed(bool frameletOrderReversed, int nframelets)
Changes the direction of the framelets.
void SetFramelet(int framelet, const double deltaT=0)
This method changes the current framelet.
int Framelet()
This method returns the current framelet.
void SetFrameletsGeometricallyFlipped(bool frameletsFlipped)
Mirrors the each framelet in the file.
virtual bool SetParent(const double sample, const double line)
Compute detector position from a parent image coordinate.
int FrameletOffset() const
Return the frame offset.
virtual double exposureDuration(const double sample, const double line, const int band) const
This virtual method is for returning the exposure duration of a given pixel.
double p_parentSample
The parent sample calculated from the detector.
int p_bandStartDetector
The first detector line of current band.
double p_etStart
Starting time at the top of the first parent line.
double p_frameletLine
The line in the current framelet.
double p_detectorLine
Detector coordinate line value.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
int p_framelet
The current framelet.
int frameletHeight() const
This returns how many lines are considered a single framelet.
virtual bool SetDetector(const double sample, const double line)
Compute parent position from a detector coordinate.
double frameletLine() const
This returns the calculated framelet line.
int GetBandFirstDetectorLine()
Return the starting line in the detector for the current band.
virtual ~PushFrameCameraDetectorMap()
Destructor.
double p_frameletRate
iTime between framelets in parent cube.
int p_frameletHeight
Height of a framelet in detector lines.
void SetFrameletRate(const double frameletRate)
Reset the frame rate.
virtual bool SetDetector(const double sample, const double line)
Compute parent position from a detector coordinate.
virtual double LineScaleFactor() const
Return scaling factor for computing line resolution.
Camera * p_camera
Pointer to the camera.
int p_nframelets
If flipped framelets, the number of framelets in this band.
double p_exposureDuration
Exposure duration in secs.
virtual bool SetParent(const double sample, const double line)
Compute detector position from a parent image coordinate.
double p_frameletSample
The sample in the current framelet.
void SetBandFirstDetectorLine(int firstLine)
Change the starting line in the detector based on band.
double frameletSample() const
This returns the calculated framelet sample.
double p_detectorSample
Detector coordinate sample value.
void SetStartTime(const double etStart)
Reset the starting ephemeris time.
PushFrameCameraDetectorMap(Camera *parent, const double etStart, const double frameletRate, int frameletHeight)
Construct a detector map for push frame cameras.
bool p_timeAscendingFramelets
Are framelets reversed from top-to-bottom in file.
double StartEphemerisTime() const
This returns the starting ET of this band.
This is free and unencumbered software released into the public domain.
void SetExposureDuration(double exposureDuration)
Change the exposure duration in seconds.
bool timeAscendingFramelets()
Returns if the framelets are reversed from top-to-bottom.