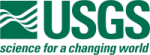 |
Isis 3 Programmer Reference
|
7 #include "RollingShutterCameraDetectorMap.h"
27 std::vector<double> times,
28 std::vector<double> sampleCoeffs,
77 const double deltaT) {
78 std::pair<double, double> jittered =
removeJitter(sample, line);
123 std::pair<double, double> jittered =
removeJitter(sample, line);
124 double currentSample = sample;
125 double currentLine = line;
128 int maxIterations = 50;
135 while((qFabs(sample - currentSample) < 1e-7) &&
136 (qFabs(line - currentLine) < 1e-7)) {
138 currentSample = (currentSample - jittered.first);
139 currentLine = (currentLine - jittered.second);
144 if (iterations > maxIterations) {
145 QString message =
"Max Iterations reached.";
151 return std::pair<double, double>(sample + currentSample, line + currentLine);
169 double sampleDejitter = 0.0;
170 double lineDejitter = 0.0;
172 for (
unsigned int n = 1; n <=
m_lineCoeffs.size(); n++) {
182 timeEntry = (int)round(line);
184 double time =
m_times[timeEntry - 1];
186 time = pow(time, exponent);
187 sampleDejitter += (sampleCoeff * time);
188 lineDejitter += (lineCoeff * time);
190 return std::pair<double, double>(sample - sampleDejitter, line - lineDejitter);
iTime time() const
Returns the ephemeris time in seconds which was used to obtain the spacecraft and sun positions.
virtual bool SetParent(const double sample, const double line)
Compute detector position from a parent image coordinate.
double p_parentLine
The parent line calculated from the detector.
Convert between parent image coordinates and detector coordinates.
@ Unknown
A type of error that cannot be classified as any of the other error types.
double p_parentSample
The parent sample calculated from the detector.
double p_detectorLineSumming
The scaling factor for computing line resolution.
virtual ~RollingShutterCameraDetectorMap()
Destructor.
double p_detectorLine
Detector coordinate line value.
std::vector< double > m_lineCoeffs
List of coefficients for the n-order polynomial characterizing the jitter in the line direction.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
std::vector< double > m_sampleCoeffs
List of coefficients for the n-order polynomial characterizing the jitter in the sample direction.
double p_detectorSampleSumming
The scaling factor for computing sample resolution.
Camera * p_camera
Pointer to the camera.
virtual bool SetDetector(const double sample, const double line)
Compute parent position from a detector coordinate.
double p_detectorSample
Detector coordinate sample value.
std::pair< double, double > applyJitter(const double sample, const double line)
Iteratively finds a solution to "apply" jitter to an image coordinate.
bool isTimeSet()
Returns true if time has been initialized.
std::pair< double, double > removeJitter(const double sample, const double line)
Remove the distortion from the image (parent) coordinates.
std::vector< double > m_times
List of normalized [-1, 1] readout times for all the lines in the input image.
This is free and unencumbered software released into the public domain.
RollingShutterCameraDetectorMap(Camera *parent, std::vector< double > times, std::vector< double > sampleCoeffs, std::vector< double > lineCoeffs)
Constructs a RollingShutterCameraDetectorMap.