Loading [MathJax]/jax/output/NativeMML/config.js
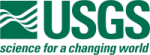 |
Isis 3 Programmer Reference
|
10 #include <QScopedPointer>
12 #include <QStringList>
15 #include <boost/foreach.hpp>
18 #include "IException.h"
19 #include "GisGeometry.h"
21 #include "PvlFlatMap.h"
22 #include "PvlObject.h"
33 Strategy::Strategy() : m_globals(), m_definition(new
PvlObject(
"Strategy")),
34 m_name(
"Strategy"), m_type(
"Counter"),
35 m_total(0), m_applyDiscarded(false), m_debug(false),
50 m_name(name), m_type(type), m_total(0),
51 m_applyDiscarded(false), m_debug(false), m_progress() {
77 m_globals(globals), m_definition(new
PvlObject(definition)),
78 m_name(
"Strategy"), m_type(
"Unknown"),
79 m_total(0), m_applyDiscarded(false), m_debug(false), m_progress() {
163 v_globals.append(myGlobals);
166 v_globals.push_back(myGlobals);
167 v_globals.append(globals);
169 return ( v_globals );
173 const PvlObject &Strategy::getDefinition()
const {
206 QString descr =
"Strategy::" +
name() +
" is running a " +
type() +
283 cout <<
"Empty apply is called...\n";
343 if ( resource->isDiscarded() ) {
345 result +=
apply(resource, globals);
350 result +=
apply(resource, globals);
409 if ( resource->isDiscarded() ) { n++; }
425 const QString &name)
const {
427 if ( resource->hasAsset(
name) ) {
428 QVariant v_asset = resource->asset(
name);
453 const QString &defValue)
const {
455 if ( keystore->exists(target) ) {
456 if ( keystore->count(target) > index ) {
457 return (keystore->value(target, index));
478 const QString &delimiter)
const {
497 const QString &replacement)
const {
499 str.replace(target, replacement, Qt::CaseInsensitive);
524 const QString &defValue)
const {
532 QString keyword = keyBase +
"Keyword";
533 if ( keys.
exists(keyword) ) {
534 idArgs.push_back(keys.
get(keyword));
535 value = keys.
get(keyBase,
"%1");
538 if ( keys.
exists(keyBase +
"Args") ) {
539 idArgs = keys.
allValues(keyBase +
"Args");
541 value = keys.
get(keyBase, defValue);
544 return (
processArgs(value, idArgs, globals, defValue));
572 const QString &defValue)
const {
574 QString result = value;
575 int i = argKeys.size();
576 BOOST_REVERSE_FOREACH ( QString arg, argKeys ) {
577 QString target(
"%"+QString::number(i));
597 BOOST_FOREACH ( QString key, keySources ) {
598 if ( source->exists(key) ) {
599 target->add(source->keyword(key));
639 QString
id = resourceA->name() +
"_" + resourceB->name();
644 if ( !keySources.isEmpty() ) {
646 BOOST_FOREACH ( QString key, keySources ) {
647 if ( resourceA->exists(key) ) {
649 keyword.
setName(keyword.
name().append(keySuffix.first));
653 if ( resourceB->exists(key) ) {
655 keyword.
setName(keyword.
name().append(keySuffix.second));
664 while ( rkeysA.constEnd() != pkeys) {
666 keyword.
setName(keyword.
name().append(keySuffix.first));
672 pkeys = rkeysB.begin();
673 while ( rkeysB.constEnd() != pkeys) {
675 keyword.
setName(keyword.
name().append(keySuffix.second));
709 QString geom(keys.
get(
"GisGeometry",
""));
712 if ( keys.
exists(
"GisGeometryRef") ) { giskey = keys.
get(
"GisGeometryRef"); }
713 if ( keys.
exists(
"GisGeometryKey") ) { giskey = keys.
get(
"GisGeometryKey"); }
715 if ( !giskey.isEmpty() ) {
716 if ( !resource->isNull(giskey) ) {
717 geom = resource->value(giskey);
720 if (
toBool(keys.
get(
"RemoveGisKeywordAfterImport",
"false")) ) {
721 resource->erase(giskey);
727 if ( !geom.isEmpty() ) {
730 if ( keys.
exists(
"GisGeometryArgs") ) {
736 QString gisType = keys.
get(
"GisType");
739 double tolerance(0.0);
743 if ( !geom.isEmpty() ) {
746 if ( geosgeom.isNull() || !geosgeom->isValid() )
return (
false);
748 npointsOrg = npoints = geosgeom->points();
752 if ( !gisTolerance.isEmpty() ) {
755 if ( 0 != simple ) geosgeom.reset(simple);
756 npoints = geosgeom->
points();
759 resource->add(geosgeom.take());
765 if ( !pointsKey.isEmpty() ) {
766 resource->add(pointsKey,
toString(npoints));
767 resource->add(pointsKey+
"Original",
toString(npointsOrg));
768 resource->add(pointsKey+
"Tolerance",
toString(tolerance));
773 cout <<
" " <<
type() <<
":" <<
name() <<
" has a geometry with "
774 << npoints <<
" points!\n";
775 if ( npoints != npointsOrg ) {
776 cout <<
" Geometry has been simplified/reduced from original "
777 << npointsOrg <<
" points.\n";
786 cout <<
" " <<
type() <<
":" <<
name() <<
" does not have a geometry!\n";
804 if ( !resource->isDiscarded() ) { v_active.append(resource); }
817 resource->activate();
883 const bool &withAssets)
const {
886 v_clone.append(
SharedResource(resource->clone(resource->name(), withAssets)));
938 QString mess =
type() +
":" +
name() +
939 "Cannot apply RTree search to bad geometry.";
948 if (
"direct" == method ) {
950 cout <<
"Using direct Geom intersects for " << v_active.size() <<
" geometries...\n";
957 if ( resource->hasValidGeometry() ) {
958 if ( geom.
intersects( *resource->geometry()) ) {
959 overlaps.append(resource);
969 cout <<
"Allocating " << v_active.size() <<
" RTree geometries.\n";
971 GEOSSTRtree *rtree = GEOSSTRtree_create(v_active.size());
973 QString mess =
"GEOS RTree allocation failed for " +
974 toString(v_active.size()) +
" geometries.";
979 cout <<
"GEOS RTree allocated " << v_active.size() <<
" geometry entries.\n";
986 for (
int i = 0 ; i < v_active.size() ; i++ ) {
987 if ( v_active[i]->hasValidGeometry() ) {
988 GEOSSTRtree_insert(rtree, v_active[i]->geometry()->geometry(),
997 cout <<
"Valid Geometries found: " << nvalid <<
" - running query...\n";
1002 GEOSSTRtree_destroy(rtree);
1006 cout <<
"Potential Intersections Found: " << overlaps.size() <<
"\n";
1021 resource->activate();
1022 n +=
apply(resource, globals);
1028 cout <<
"Total valid Intersections Found: " << n <<
"\n";
1052 rlist->append(*resource);
1103 QString p_text = text;
1105 bool status =
false;
1110 if (
toBool(p_var.
get(
"ShowProgress",
"false")) ) {
1112 if ( p_text.isEmpty() ) {
1121 if ( !p_text.isEmpty() )
m_progress->SetText(p_text);
1134 PvlObject::ConstPvlObjectIterator
object = source.
beginObject();
1135 while (
object != source.
endObject() ) {
1136 objList <<
object->name();
QString name() const
Returns the keyword name.
int points() const
Get number of points in geometry.
Contains Pvl Groups and Pvl Objects.
QString processArgs(const QString &value, const QStringList &argKeys, const ResourceList &globals, const QString &defValue="") const
Processes the given string value using the given argument list, resource and default resource.
bool initProgress(const int &nsteps=0, const QString &text="")
Initializes strategy progress monitor if requested by user.
A single keyword-value pair.
ResourceList activeList(ResourceList &resources) const
Get list of all active Resources only - no discarded Resources.
void deactivateList(ResourceList &resources) const
Deactivate all resources contained in the resource list.
Provides a flat map of PvlKeywords.
PvlObjectIterator beginObject()
Returns the index of the beginning object.
ResourceList cloneList(const ResourceList &resources, const bool &withAssets=false) const
Create a clone of a Resource list.
ResourceList copyList(const ResourceList &resources) const
Make a copy of the resource list that is independently managed.
void propagateKeys(SharedResource &source, SharedResource &target) const
Adds the PVL definition keywords from the source to the target.
ResourceList getGlobals(SharedResource &myGlobals, const ResourceList &globals) const
PvlObjectIterator endObject()
Returns the index of the ending object.
QMap< QString, PvlKeyword >::const_iterator ConstPvlFlatMapIterator
A const iterator for the underling QMap that PvlFlatMap is built on.
Type type() const
Returns the type (origin) of the geometry.
ResourceList getGlobalDefaults() const
Accessor method to get the global defaults.
bool exists(const QString &key) const
Determines whether a given keyword exists in the PvlFlatMap.
const GEOSGeometry * geometry() const
Returns the GEOSGeometry object to extend functionality.
int applyToIntersectedGeometry(ResourceList &resources, GisGeometry &geom, const ResourceList &globals)
Identify and apply Strategy to Resources that intersect a geometry.
Strategy()
Constructs default Strategy object of name "Strategy" and type "Counter".
SharedPvlObject m_definition
A shared pointer to the PvlObject that defines the strategy.
void setDoNotApplyToDiscarded()
Disables the general application of Strategy algorithm for all Resources regardless of state.
unsigned int processed()
Increments the total number of resources processed and returns the incremented value.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
void resetProcessed()
Resets the total number of processed resources to zero.
bool importGeometry(SharedResource &resource, const ResourceList &globals) const
Imports a geometry from the given resource.
void activateList(ResourceList &resources) const
Activate all resources contained in the resource list.
SharedResource composite(SharedResource &resourceA, SharedResource &resourceB, const QPair< QString, QString > &keySuffix=qMakePair(QString("A"), QString("B"))) const
Create a composite Resource from a pair by merging keywords.
Encapsulation class provides support for GEOS-C API.
QScopedPointer< Progress > m_progress
Progress percentage monitor.
QString m_name
A string containing the name of the strategy.
void setName(QString name)
Sets the keyword name.
QStringList allValues(const QString &key) const
Gets all the values associated with a keyword in the PvlFlatMap.
ResourceList m_globals
A shared pointer to the global Resource of keywords.
static PvlConstraints withExcludes(const QStringList &excludes)
Static method to construct a PvlConstraints object from a list of names for the PvlObjects and PvlGro...
int applyToResources(ResourceList &resources, const ResourceList &globals)
Applies the strategy algorithms to the resources in the given list.
bool isDebug() const
An accessor method so that inherited classes can determine whether to print debug messages for this o...
QString get(const QString &key, const int &index=0) const
Gets the value of a keyword in the PvlFlatMap.
GisGeometry * simplify(const double &tolerance) const
Simplify complex or overdetermined geoemtry.
bool m_applyDiscarded
Indicates whether to apply strategy to discarded resources.
int countActive(const ResourceList &resources) const
Counts the number of active (i.e.
QStringList qualifiers(const QString &keyspec, const QString &delimiter="::") const
Splits the given keyspec string into a list using the given delimiter string.
int countDiscarded(const ResourceList &resources) const
Counts the number of non-active (i.e.
Program progress reporter.
This class provides a resource of PVL keywords for Strategy classes.
bool doShowProgress() const
bool m_debug
Indicates whether to print debug messages.
QString findReplacement(const QString &target, const ResourceList &globals, const int &index=0, const QString &defValue="") const
Find keyword replacement value in globals list.
bool intersects(const GisGeometry &target) const
Computes a new geometry from the intersection of the two geomtries.
int apply(ResourceList &resources)
Apply algorithm to resource list.
double toDouble(const QString &string)
Global function to convert from a string to a double.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
static void queryCallback(void *item, void *userdata)
Important GEOS query callback for class and overlap geometry.
QString scanAndReplace(const QString &input, const QString &target, const QString &replacement) const
Performs a case insensitive scan of the input string for a substring matching the target string and r...
QString m_type
A string containing the type of strategy.
This is free and unencumbered software released into the public domain.
bool toBool(const QString &string)
Global function to convert from a string to a boolean.
PvlFlatMap getDefinitionMap() const
Returns the keyword definitions found in the Strategy object.
unsigned int m_total
The total number of resources processed.
virtual ~Strategy()
Destroys the Strategy object.
QSharedPointer< Resource > SharedResource
Defintion of a SharedResource, a shared pointer to a Resource object.
QString name() const
Accessor method to get the name of the strategy.
QString type() const
Accessor method to get the type of strategy.
QString description() const
Return description for the strategy.
void setName(const QString &name)
Allow derived strategies to reset name (mostly for default constructors)
bool isApplyToDiscarded() const
Accessor for the apply discarded variable.
bool isValid() const
Determines validity of the geometry contained in this object.
void setApplyToDiscarded()
Sets Resource as discarded.
void setType(const QString &type)
Allow derived strategies to reset type (mostly for default constructors)
ResourceList assetResourceList(const SharedResource &resource, const QString &name) const
Searches the given resource for an asset with the given name and converts it to a ResourceList,...
This is free and unencumbered software released into the public domain.
unsigned int totalProcessed() const
Accessor for the total number of resources processed.
QString translateKeywordArgs(const QString &value, const ResourceList &globals, const QString &defValue="") const
Translates the arguments of the PVL keyword in the PVL definition object.