File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
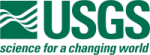 |
Isis 3 Programmer Reference
|
7 #include "TriangularPlate.h"
15 #include "AbstractPlate.h"
18 #include "Intercept.h"
19 #include "IException.h"
22 #include "Longitude.h"
23 #include "NaifDskApi.h"
24 #include "SurfacePoint.h"
36 TriangularPlate::TriangularPlate(
const NaifTriangle &plate,
const int &plateId) :
38 m_plateId(plateId) { }
40 TriangularPlate::~TriangularPlate() { }
42 int TriangularPlate::id()
const {
47 return "TriangularPlate";
65 double radius = qMax(qMax(vnorm_c(
m_plate[0]), vnorm_c(
m_plate[1])),
71 double radius = qMin(qMin(vnorm_c(
m_plate[0]), vnorm_c(
m_plate[1])),
90 double s1 = vnorm_c(&edge[0]);
93 double s2 = vnorm_c(&edge[0]);
96 double s3 = vnorm_c(&edge[0]);
99 double S = (s1 + s2 + s3) / 2.0;
100 double p_area = std::sqrt(S * (S - s1) * (S - s2) * (S - s3));
125 ucrss_c(&edge1[0], &edge2[0], &norm[0]);
131 double third(0.33333333333333331);
156 double sepang = vsep_c(&norm[0], &raydir[0]);
211 vminus_c(&obs[0], &raydir[0]);
249 vminus_c(&obs[0], &raydir[0]);
308 if ( (v < 0) || (v > 2) ) {
309 QString msg =
"Unable to get TriangularPlate vertex for index ["
310 +
toString(v) +
"]. Valid index range is 0-2.";
313 for (
int i = 0 ; i < 3 ; i++ ) {
363 vsub_c(
m_plate[0], &obs[0], &e1[0]);
364 vsub_c(
m_plate[1], &obs[0], &e2[0]);
365 vsub_c(
m_plate[2], &obs[0], &e3[0]);
369 vcrss_c(&e1[0], &e2[0], &tnorm12[0]);
370 double tdot12 = vdot_c(&raydir[0], &tnorm12[0]);
371 double en = vdot_c(&e3[0], &tnorm12[0]);
375 if ( qFuzzyCompare(en+1.0, 1.0) )
return (
false);
378 if ( (en > 0.0) && (tdot12 < 0.0) )
return (
false);
379 if ( (en < 0.0) && (tdot12 > 0.0) )
return (
false);
384 vcrss_c(&e2[0], &e3[0], &tnorm23[0]);
385 double tdot23 = vdot_c(&raydir[0], &tnorm23[0]);
388 if ( (en > 0.0) && (tdot23 < 0.0) )
return (
false);
389 if ( (en < 0.0) && (tdot23 > 0.0) )
return (
false);
394 vcrss_c(&e3[0], &e1[0], &tnorm31[0]);
395 double tdot31 = vdot_c(&raydir[0], &tnorm31[0]);
398 if ( (en > 0.0) && (tdot31 < 0.0) )
return (
false);
399 if ( (en < 0.0) && (tdot31 > 0.0) )
return (
false);
403 double denom = tdot12 + tdot23 + tdot31;
411 if ( qFuzzyCompare(denom+1.0, 1.0) )
return (
false);
413 double scale = en / denom;
415 vlcom_c(1.0, &obs[0], scale, &raydir[0], &xpt[0]);
Angle separationAngle(const NaifVector &raydir) const
Computes the separation angle from the plate normal of a given vector.
double kilometers() const
Get the distance in kilometers.
TNT::Array1D< SpiceDouble > NaifVertex
1-D Buffer[3]
This class is designed to encapsulate the concept of a Latitude.
NaifVertex vertex(int v) const
Returns the vth point of the triangle.
Intercept * intercept(const NaifVertex &vertex, const NaifVector &raydir) const
Conpute the intercept point on a triangular plate.
Abstract interface to a TIN plate.
SurfacePoint * point(const Latitude &lat, const Longitude &lon) const
Determine the intercept point of a lat/lon location for the plate.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
@ Kilometers
The distance is being specified in kilometers.
Distance maxRadius() const
Determines the maximum radius from all the vertices of the plate.
bool hasPoint(const Latitude &lat, const Longitude &lon) const
Determines the give lat/lon point intercept the triangular plate.
AbstractPlate * clone() const
Retrns a clone of the current plate.
TNT::Array2D< SpiceDouble > NaifTriangle
3-D triangle[3][3]
QString name() const
Gets the name of this Plate type.
void FromNaifArray(const double naifValues[3])
A naif array is a c-style array of size 3.
NaifTriangle m_plate
Tetrahedron, defined by the coordinate system origin and 3 vertices, used to represent the Triangular...
NaifVector normal() const
Compute the surface normal of the plate.
Defines an angle and provides unit conversions.
bool findPlateIntercept(const NaifVertex &obs, const NaifVector &raydir, NaifVertex &point) const
Determines of if given a vertex and look direction intercepts the plate.
double area() const
Returns the area of the plate in km.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
Container for a intercept condition.
int m_plateId
ID for this plate on the ShapeModel.
void ToNaifArray(double naifOutput[3]) const
A naif array is a c-style array of size 3.
Distance minRadius() const
Gets the minimum radius.
TNT::Array1D< SpiceDouble > NaifVector
Namespace to contain type definitions of NAIF DSK fundamentals.
This class defines a body-fixed surface point.
Specification for an abstract triangular plate.
@ Radians
Radians are generally used in mathematical equations, 0-2*PI is one circle, however these are more di...
This is free and unencumbered software released into the public domain.
bool hasIntercept(const NaifVertex &vertex, const NaifVector &raydir) const
Determines if a look direction from a point intercepts the plate.