File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
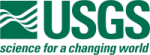 |
Isis 3 Programmer Reference
|
9 #include "VimsCamera.h"
10 #include "VimsGroundMap.h"
11 #include "VimsSkyMap.h"
22 #include "CameraDetectorMap.h"
23 #include "CameraDistortionMap.h"
24 #include "CameraFocalPlaneMap.h"
25 #include "Constants.h"
27 #include "IException.h"
30 #include "NaifStatus.h"
31 #include "SpecialPixel.h"
63 QString channel = (QString) inst [
"Channel"];
68 if (channel ==
"VIS") {
72 if (QString((QString)inst[
"SamplingMode"]).toUpper() ==
"NORMAL") {
75 m_pixelPitchX = 0.024 * 3;
76 m_pixelPitchY = 0.024 * 3;
78 else if (QString((QString)inst[
"SamplingMode"]).toUpper() ==
"HI-RES") {
80 m_pixelPitchX = 0.024;
81 m_pixelPitchY = 0.024;
83 else if (QString((QString)inst[
"SamplingMode"]).toUpper() ==
"UNDER") {
84 QString msg =
"Isis cannot process images with a SamplingMode = \"UNDER\" (or NYQUIST)";
88 QString msg =
"Unknown SamplingMode [" + (QString) inst[
"SamplingMode"] +
"]";
92 else if (channel ==
"IR") {
97 if (QString((QString)inst[
"SamplingMode"]).toUpper() ==
"NORMAL") {
101 else if (QString((QString)inst[
"SamplingMode"]).toUpper() ==
"HI-RES") {
102 m_pixelPitchX = 0.103;
105 else if (QString((QString)inst[
"SamplingMode"]).toUpper() ==
"UNDER") {
106 QString msg =
"Isis cannot process images with a SamplingMode = \"UNDER\" (or NYQUIST)";
110 QString msg =
"Unknown SamplingMode [" + (QString) inst[
"SamplingMode"] +
"]";
116 QString stime = inst [
"NativeStartTime"][0];
117 QString intTime = stime.split(
".").first();
118 stime = stime.split(
".").last();
125 etStart +=
toDouble(stime) / 15959.0 - 2.;
128 QString etime = (QString) inst [
"NativeStopTime"];
129 intTime = etime.split(
".").first();
130 etime = etime.split(
".").last();
137 etStop +=
toDouble(stime) / 15959.0 + 2.;
181 for (
double x = -m_pixelPitchX / 2.0; x <= m_pixelPitchX / 2.0; x += m_pixelPitchX / (npts-1)) {
182 offsets.append(QPointF(x, -m_pixelPitchY / 2.0));
185 for (
double y = -m_pixelPitchY / 2.0; y <= m_pixelPitchY / 2.0; y += m_pixelPitchY / (npts-1)) {
186 offsets.append(QPointF(m_pixelPitchX / 2.0, y));
189 for (
double x = m_pixelPitchX / 2.0; x >= -m_pixelPitchX / 2.0; x -= m_pixelPitchX / (npts-1)) {
190 offsets.append(QPointF(x, m_pixelPitchY / 2.0));
193 for (
double y = m_pixelPitchY / 2.0; y >= -m_pixelPitchY / 2.0; y -= m_pixelPitchY / (npts-1)) {
194 offsets.append(QPointF(-m_pixelPitchX / 2.0, y));
void IgnoreProjection(bool ignore)
Set whether or not the camera should ignore the Projection.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
Convert between parent image coordinates and detector coordinates.
CameraSkyMap * SkyMap()
Returns a pointer to the CameraSkyMap object.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
QString m_instrumentNameLong
Full instrument name.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
Container for cube-like labels.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
@ Traverse
Search child objects.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
Convert between undistorted focal plane and ground coordinates.
virtual QList< QPointF > PixelIfovOffsets()
Returns the pixel ifov offsets from center of pixel.
Distort/undistort focal plane coordinates.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
double toDouble(const QString &string)
Global function to convert from a string to a double.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
CameraGroundMap * GroundMap()
Returns a pointer to the CameraGroundMap object.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
Cassini Vims camera model.
Convert between undistorted focal plane and ground coordinates.
QString m_spacecraftNameShort
Shortened spacecraft name.
void SetFocalLength()
Reads the focal length from the instrument kernel.
This is free and unencumbered software released into the public domain.