Loading [MathJax]/jax/output/NativeMML/config.js
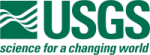 |
Isis 3 Programmer Reference
|
16 #include "ZeroBufferFit.h"
18 #include "HiCalTypes.h"
19 #include "HiCalUtil.h"
20 #include "HiCalConf.h"
21 #include "LeastSquares.h"
22 #include "LowPassFilter.h"
23 #include "MultivariateStatistics.h"
24 #include "IException.h"
38 ZeroBufferFit::ZeroBufferFit(
const HiCalConf &conf) :
42 _timet.setBin(
ToInteger(prof(
"Summing")));
43 _timet.setLineTime(
ToDouble(prof(
"ScanExposureDuration")));
45 _skipFit =
IsEqual(
ConfKey(prof,
"ZeroBufferFitSkipFit", QString(
"TRUE")),
"TRUE");
46 _useLinFit =
IsTrueValue(prof,
"ZeroBufferFitOnFailUseLinear");
52 _sWidth =
toInt(
ConfKey(prof,
"GuessFilterWidth", QString(
"17")));
53 _sIters =
toInt(
ConfKey(prof,
"GuessFilterIterations", QString(
"1")));
55 if ( prof.
exists(
"MaximumIterations") ) {
61 _minLines =
toInt(
ConfKey(prof,
"ZeroBufferFitMinimumLines", QString(
"100")));
64 QString histstr =
"ZeroBufferFit(AbsErr[" +
ToString(_absErr) +
101 hist <<
"NotEnoughLines(GoodLines[" <<
goodLines(d)
102 <<
"],MinimumLines[" << _minLines <<
"]);";
105 hist <<
"SkipFit(TRUE: Not using LMFit)";
115 <<
"],DoF[" <<
DoF() <<
"])";
132 hist <<
"Failed::Reason("<<
statusstr() <<
"),#Iters["
140 _history.add(
"OnFailureUse(LinearFit(Zf))");
144 _history.add(
"OnFailureUse(ZfBuffer)");
164 int nb = n - _badLines;
166 HiVector b1 = _data.subarray(0, nb-1);
178 int n0 =
MIN(nb, 20);
179 for (
int k = 0 ; k < n0 ; k++ ) {
180 double d = _b2[k] - (cc[0] + cc[1] * _timet(k));
187 n0 = (int) (0.9 * nb);
188 for (
int l = n0 ; l < nb ; l++ ) {
189 double d = _b2[l] - (cc[0] + cc[1] * _timet(l));
199 g[3] = -5.0/_timet(nb-1);
220 const NLVector &uncerts,
double cplxconj,
222 _chisq = pow(cplxconj, 2.0);
235 for (
int i = 0 ; i < n ; i++) {
236 double lt = _timet(i);
238 double Yi = a0 + (a1 * lt) + a2 * exp(
MIN(et,_maxLog));
239 f[i] = (Yi - _b2[i]);
253 for (
int i = 0; i < n; i++) {
254 double lt = _timet(i);
256 double p0 = exp (
MIN(et,_maxLog));
260 J[i][3] = a2 * lt * p0;
268 return (_data.copy());
273 for (
int i = 0 ; i < dcorr.dim() ; i++ ) {
274 double lt = _timet(i);
275 dcorr[i] = a[0] + (a[1] * lt) + a[2] * exp(a[3] * lt);
286 for (
int i = 0 ; i < v.dim() ; i++ ) {
287 vNorm[i] = v[i] - v0;
309 for (
int i = 0 ; i < n ; i++ ) {
310 double t = _timet(line0+i);
320 o <<
"# History = " <<
_history << endl;
328 for (
int i = 0 ; i < _data.dim() ; i++) {
HiHistory _history
Hierarchial component history.
bool IsEqual(const QString &v1, const QString &v2="TRUE")
Shortened string equality test.
int _fmtWidth
Default field with of double.
This class is used to accumulate statistics on double arrays.
void AddData(const double *data, const unsigned int count)
Add an array of doubles to the accumulators and counters.
QString Name() const
Returns the name of this property.
HiVector Solve(const HiVector &d)
Compute non-linear fit to (typically) ZeroBufferSmooth module.
QString ToString(const T &value)
Helper function to convert values to strings.
bool exists(const QString &key) const
Checks for the existance of a keyword.
Module manages HiRISE calibration vectors from various sources.
const HiVector & ref() const
Return data via a const reference.
T ConfKey(const DbProfile &conf, const QString &keyname, const T &defval, int index=0)
Find a keyword in a profile using default for non-existant keywords.
int goodLines(const HiVector &d) const
Returns the number of good lines in the image.
void Reset()
Reset all accumulators and counters to zero.
NLVector uncert() const
Return uncertainties from last fit processing.
bool gotGoodLines(const HiVector &d) const
Determines if the vector contains any valid lines.
void setMaxIters(int m)
Sets the maximum number of iterations.
Compute a low pass filter from a Module class content.
void AddData(const double *x, const double *y, const unsigned int count)
Add two arrays of doubles to the accumulators and counters.
NLVector coefs() const
Return coefficients from last fit processing.
int DoF() const
Returns the Degrees of Freedom.
QString formatDbl(const double &value) const
Properly format values that could be special pixels.
HiVector Normalize(const HiVector &v)
Compute normalized solution vector from result.
bool success() const
Determine success from last fit processing.
NonLinearLSQ Computes a fit using a Levenberg-Marquardt algorithm.
Container of multivariate statistics.
int toInt(const QString &string)
Global function to convert from a string to an integer.
int maxIters() const
Maximum number iterations for valid solution.
int ToInteger(const T &value)
Helper function to convert values to Integers.
virtual void printOn(std::ostream &o) const
Provides virtualized dump of data from this module.
NLMatrix df_x(const NLVector &a)
Computes the first derivative of the function at the current iteration.
bool IsTrueValue(const DbProfile &prof, const QString &key, const QString &value="TRUE")
Determines if the keyword value is the expected value.
T MIN(const T &A, const T &B)
Implement templatized MIN fumnction.
A DbProfile is a container for access parameters to a database.
TNT::Array1D< double > HiVector
1-D Buffer
std::string statusstr() const
Return error message pertaining to last fit procesing.
double Average() const
Computes and returns the average.
double ToDouble(const T &value)
Helper function to convert values to doubles.
void LinearRegression(double &a, double &b) const
Fits a line.
HiVector Yfit() const
Computes the solution vector using current coefficents.
HiVector poly_fit(const HiVector &d, const double line0=0.0) const
Compute a polyonomial fit using multivariate statistics.
double toDouble(const QString &string)
Global function to convert from a string to a double.
Namespace for the standard library.
int checkIteration(const int Iter, const NLVector &fitcoefs, const NLVector &uncerts, double cplxconj, int Istatus)
Computes the interation check for convergence.
NLVector f_x(const NLVector &a)
Computes the function value at the current iteration.
NLVector guess()
Compute the initial guess of the fit.
int nIterations() const
Return number of iterations from last fit processing.
double Chisq() const
Returns the Chi-Square value of the fit solution.
This is free and unencumbered software released into the public domain.