File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
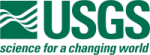 |
Isis 3 Programmer Reference
|
9 #include "ApolloPanoramicCamera.h"
11 #include "ApolloPanIO.h"
12 #include "ApolloPanoramicDetectorMap.h"
16 #include "CameraDistortionMap.h"
17 #include "CameraFocalPlaneMap.h"
18 #include "IException.h"
21 #include "LineScanCameraDetectorMap.h"
22 #include "LineScanCameraGroundMap.h"
23 #include "LineScanCameraSkyMap.h"
25 #include "PvlKeyword.h"
41 double constantTimeOffset = 0.0,
42 additionalPreroll = 0.0,
43 additiveLineTimeError = 0.0,
44 multiplicativeLineTimeError = 0.0;
66 QString msg =
"File does not appear to be an Apollo image";
78 additiveLineTimeError =
getDouble(ikernKey);
81 multiplicativeLineTimeError =
getDouble(ikernKey);
85 QString stime = (QString)inst[
"StartTime"];
87 str2et_c(stime.toLatin1().data(), &etStart);
88 stime = (QString) inst[
"StopTime"];
90 str2et_c(stime.toLatin1().data(), &etStop);
91 iTime isisTime( (QString) inst[
"StartTime"]);
95 double lineRate = ( (double) inst[
"LineExposureDuration"] )*0.005;
97 lineRate *= 1.0 + multiplicativeLineTimeError;
98 lineRate += additiveLineTimeError;
99 etStart += additionalPreroll * lineRate;
100 etStart += constantTimeOffset;
109 (etStart+etStop)/2.0,
110 (
double)lineRate, &lab);
123 double sampleBoreSight = 0.0;
124 double lineBoreSight = 0.0;
Convert between undistorted focal plane and ra/dec coordinates.
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
double m_residualStdev
Standard deviation of interior orientation residual vector length.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
Parse and return pieces of a time string.
double m_residualMean
Max interior orientation residual vector length.
QString m_instrumentNameLong
Full instrument name.
int m_CkFrameId
CK "Camera Matrix" kernel frame ID.
void SetDetectorSampleSumming(const double summing)
Set sample summing mode.
Container for cube-like labels.
void SetDetectorOffset(const double sampleOffset, const double lineOffset)
Set the detector offset.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
@ Traverse
Search child objects.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
int toInt(const QString &string)
Global function to convert from a string to an integer.
double meanResidual()
Mean (average) of interior orientation residual vector lengths, accesor.
double stdevResidual()
Standard deviation of interior orientation residual vector lengths, accesor.
Generic class for Line Scan Cameras.
Convert between undistorted focal plane and ground coordinates.
Distort/undistort focal plane coordinates.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
void SetStartingDetectorSample(const double sample)
Set the starting detector sample.
Convert between parent image (aka encoder aka machine) coordinates and detector coordinates.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
QString m_spacecraftNameShort
Shortened spacecraft name.
void SetFocalLength()
Reads the focal length from the instrument kernel.
double m_residualMax
Mean (average) of interior orientation residual vector length.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
double maxResidual()
Max interior orientation residual vector length, accesor.