File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
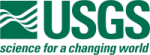 |
Isis 3 Programmer Reference
|
15 #include "PlaneShape.h"
18 #include "IException.h"
20 #include "Longitude.h"
21 #include "NaifStatus.h"
22 #include "ShapeModel.h"
23 #include "SurfacePoint.h"
78 std::vector<double> lookDirection) {
94 if (observerPos[2] < 0.0)
99 nvc2pl_c(zvec, 0.0, &plane);
101 SpiceDouble position[3];
102 SpiceDouble lookvector[3];
104 position[0] = observerPos[0];
105 position[1] = observerPos[1];
106 position[2] = observerPos[2];
108 lookvector[0] = lookDirection[0];
109 lookvector[1] = lookDirection[1];
110 lookvector[2] = lookDirection[2];
114 inrypl_c(&position, &lookvector, &plane, &nxpts, xpt);
191 vsub_c((ConstSpiceDouble *) &sB[0], pB, psB);
192 unorm_c(psB, upsB, &dist);
206 double angle = vdot_c(n, upsB);
248 vsub_c((SpiceDouble *) &uB[0], pB, puB);
249 unorm_c(puB, upuB, &dist);
262 double angle = vdot_c((SpiceDouble *) &n[0], upuB);
294 double radius = sqrt(pB[0]*pB[0] + pB[1]*pB[1] + pB[2]*pB[2]);
PlaneShape()
Initialize the PlaneShape.
This class is designed to encapsulate the concept of a Latitude.
SurfacePoint * surfaceIntersection() const
Returns the surface intersection for this ShapeModel.
void calculateLocalNormal(QVector< double * > cornerNeighborPoints)
There is no implementation for this method.
void setHasIntersection(bool b)
Sets the flag to indicate whether this ShapeModel has an intersection.
void calculateSurfaceNormal()
There is no implementation for this method.
Container for cube-like labels.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
void setNormal(const std::vector< double >)
Sets the normal for the currect intersection point.
double emissionAngle(const std::vector< double > &sB)
Computes and returns emission angle in degrees given the observer position.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
@ Kilometers
The distance is being specified in kilometers.
void setName(QString name)
Sets the shape name.
bool isDEM() const
Indicates that this shape model is not from a DEM.
double incidenceAngle(const std::vector< double > &uB)
Computes and returns incidence angle in degrees given the sun position.
void FromNaifArray(const double naifValues[3])
A naif array is a c-style array of size 3.
double kilometers() const
Get the displacement in kilometers.
Define shapes and provide utilities for Isis targets.
This is free and unencumbered software released into the public domain.
void calculateDefaultNormal()
There is no implementation for this method.
bool intersectSurface(std::vector< double > observerPos, std::vector< double > lookDirection)
Find the intersection point.
This class is used to create and store valid Isis targets.
const double RAD2DEG
Multiplier for converting from radians to degrees.
This is free and unencumbered software released into the public domain.
Distance localRadius(const Latitude &lat, const Longitude &lon)
Gets the local radius for the given latitude/longitude coordinate.