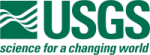 |
Isis 3 Programmer Reference
|
11 #include "ChipViewport.h"
15 #include <QMessageBox>
18 #include "ControlMeasure.h"
19 #include "ControlNet.h"
20 #include "ControlPoint.h"
22 #include "CubeViewport.h"
23 #include "SerialNumber.h"
37 setFixedSize(width, height);
38 setBackgroundRole(QPalette::Dark);
54 m_image =
new QImage(512, 512, QImage::Format_RGB32);
112 if (
chip == NULL || chipCube == NULL) {
114 "Can not view NULL chip pointer",
203 for (
int line = 1; line <
m_chip->
Lines(); line++) {
211 for (
int line = 1; line <=
m_chip->
Lines(); line++) {
224 stretch.
AddPair(-DBL_MAX, 0.0);
225 stretch.
AddPair(DBL_MAX, 255.0);
240 QRgb *rgb = (QRgb *)
m_image->scanLine(y);
244 rgb[x] = qRgb(r, g, b);
266 QPainter painter(
this);
272 painter.drawImage(0, 0, *
m_image);
276 painter.setPen(Qt::red);
282 painter.setPen(Qt::red);
289 if (m_controlNet && !serialNumber.isEmpty()
300 for (
int i = 0; i < measures.count(); i++) {
303 double samp = m->GetSample();
304 double line = m->GetLine();
310 if (m->Parent()->IsIgnored() ||
311 (!m->Parent()->IsIgnored() && m->IsIgnored())) {
312 painter.setPen(QColor(255, 255, 0));
316 painter.setPen(Qt::magenta);
319 painter.setPen(Qt::green);
324 painter.drawLine(x - 5, y, x + 5, y);
325 painter.drawLine(x, y - 5, x, y + 5);
501 if (e->key() == Qt::Key_Up) {
504 else if (e->key() == Qt::Key_Down) {
507 else if (e->key() == Qt::Key_Left) {
510 else if (e->key() == Qt::Key_Right) {
514 QWidget::keyPressEvent(e);
521 emit userMovedTackPoint();
536 QPoint p =
event->pos();
537 if (event->button() == Qt::LeftButton) {
541 emit userMovedTackPoint();
631 QString msg =
"Cannot geom chip.\n";
633 QMessageBox::information((
QWidget *)parent(),
"Error", msg);
656 QString msg =
"Cannot load no geom chip.\n";
658 QMessageBox::information((
QWidget *)parent(),
"Error", msg);
684 QString msg =
"Cannot load rotated chip.\n";
686 QMessageBox::information((
QWidget *)parent(),
"Error", msg);
712 "Can not view NULL chip pointer",
726 QString msg =
"Cannot reload chip.\n";
728 QMessageBox::information((
QWidget *)parent(),
"Error", msg);
738 QString msg =
"Cannot reload chip.\n";
740 QMessageBox::information((
QWidget *)parent(),
"Error", msg);
bool IsInsideChip(double sample, double line)
double m_zoomFactor
Zoom Factor.
Stretch stretch
Stretch for the band BandInfo constructor.
Chip * m_matchChip
The matching chip.
This class is used to accumulate statistics on double arrays.
virtual QString fileName() const
Returns the opened cube's filename.
int m_circleSize
Circle size.
void AddData(const double *data, const unsigned int count)
Add an array of doubles to the accumulators and counters.
void zoom(double zoomFactor)
Zoom by a specified factor.
void enterEvent(QEvent *e)
If mouse enters, make sure key events are processed w/o clicking.
double Percent(const double percent) const
Computes and returns the value at X percent of the histogram.
void setCircleSize(int size)
Set the size of the circle.
This is free and unencumbered software released into the public domain.
BandInfo m_gray
Info for the gray bands.
void TackCube(const double cubeSample, const double cubeLine)
This sets which cube position will be located at the chip tack position.
bool m_stretchLocked
Whether or not to lock the stretch when transforming.
Cube * m_chipCube
The chip's cube.
static QString Compose(Pvl &label, bool def2filename=false)
Compose a SerialNumber from a PVL.
double GetValue(int sample, int line)
Loads a Chip with a value.
double ChipSample() const
void SetCubePosition(const double sample, const double line)
Compute the position of the chip given a cube coordinate.
void paintImage()
Paints the chip viewport after the chip has been updated.
void setCross(bool checked)
Slot to change state of crosshair.
QList< ControlMeasure * > GetMeasuresInCube(QString serialNumber)
Get all the measures pertaining to a given cube serial number.
double tackSample()
Return the position of cube under cross hair.
double CubeSample() const
QImage * m_image
The image.
double tackLine()
Returns tack line.
QList< QString > GetCubeSerials() const
Use this method to get a complete list of all the cube serial numbers in the network.
bool m_showPoints
Draw control points.
void Load(Cube &cube, const double rotation=0.0, const double scale=1.0, const int band=1)
Load cube data into the Chip.
void computeStretch(Stretch &stretch, bool force=false)
Compute automatic stretch for a portion of the cube.
ChipViewport * m_tempView
Temporary viewport.
int TackSample() const
This method returns a chip's fixed tack sample; the middle of the chip.
void rotateChip(int rotation)
Slot to rotate chip.
@ Fixed
A Fixed point is a Control Point whose lat/lon is well established and should not be changed.
void keyPressEvent(QKeyEvent *e)
Process arrow keystrokes on cube.
int GetNumPoints() const
Return the number of control points in the network.
void refreshView(double tackSample, double tackLine)
Slot to refresh viewport when the tack point has changed.
virtual ~ChipViewport()
Destructor.
int TackLine() const
This method returns a chip's fixed tack line; the middle of the chip.
QString toString() const
Returns a string representation of this exception.
void reloadChip(double tackSample=0., double tackLine=0.)
Reloads the chip at the given tack point on the cube.
virtual void AddData(const double *data, const unsigned int count)
Add an array of doubles to the histogram counters.
void geomChip(Chip *matchChip, Cube *matchChipCube)
Slot to geom chip (apply geometry transformation)
void paintEvent(QPaintEvent *e)
Repaint the viewport.
Cube * m_matchChipCube
The matching chip's cube.
double zoomFactor()
Return the zoom factor.
bool m_circle
Draw circle.
void AddPair(const double input, const double output)
Adds a stretch pair to the list of pairs.
void ClearPairs()
Clears the stretch pairs.
double BestMaximum(const double percent=99.5) const
This method returns the better of the absolute maximum or the Chebyshev maximum.
void zoom1()
Zoom by a factor of one.
IO Handler for Isis Cubes.
Stretch * m_stretch
Current stretch on the chip viewport.
Widget to display Isis cubes for qt apps.
ChipViewport(int width, int height, QWidget *parent=0)
Construct an empty viewport.
void autoStretch()
Apply automatic stretch using data from entire chip.
void panRight()
Pan right.
void setChip(Chip *chip, Cube *chipCube)
Set chip.
void mousePressEvent(QMouseEvent *event)
Process mouse events.
void tackPointChanged(double)
< Signal sent when tack point changes
@ Programmer
This error is for when a programmer made an API call that was illegal.
void changeStretchLock(int)
Locks or unlocks the stretch on the chip viewport during transformations (zoom, pan,...
Container of a cube histogram.
double BestMinimum(const double percent=99.5) const
This method returns the better of the absolute minimum or the Chebyshev minimum.
A small chip of data used for pattern matching.
bool m_cross
Draw crosshair.
void setPoints(bool checked)
Slot to set whether control points are drawn.
void setCircle(bool checked)
Slot to change state of circle.
PointType GetType() const
Chip * chip() const
Return chip.
void loadView(ChipViewport &newView)
Load with another ChipViewport, used for blinking.
void SetChipPosition(const double sample, const double line)
Compute the position of the cube given a chip coordinate.
void nogeomChip()
Slot to un-geom chip (revert geometry transformation)
void stretchFromCubeViewport(Stretch *, CubeViewport *)
Applies a new stretch to the specified cube viewport.
bool cubeToViewport(double samp, double line, int &x, int &y)
Get viewport x and y from cube sample and line.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
double Map(const double value) const
Maps an input value to an output value based on the stretch pairs and/or special pixel mappings.