Loading [MathJax]/jax/output/NativeMML/config.js
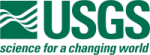 |
Isis 3 Programmer Reference
|
9 #include "ClipperNacRollingShutterCamera.h"
13 #include "CameraDistortionMap.h"
14 #include "CameraFocalPlaneMap.h"
15 #include "CameraGroundMap.h"
16 #include "CameraSkyMap.h"
19 #include "NaifStatus.h"
22 #include "RollingShutterCamera.h"
23 #include "RollingShutterCameraDetectorMap.h"
25 #include "TableRecord.h"
51 QString startTime = inst[
"StartTime"];
52 iTime etStart(startTime);
64 std::vector<double> sampleCoeffs, lineCoeffs, readoutTimes;
67 && cube.
hasTable(
"Normalized Main Readout Line Times")) {
69 for (
int i = 0; i < sampleCoefficients.
size(); i++) {
70 sampleCoeffs.push_back(sampleCoefficients[i].
toDouble());
74 for (
int i = 0; i < lineCoefficients.
size(); i++) {
75 lineCoeffs.push_back(lineCoefficients[i].
toDouble());
78 Table normalizedReadoutTimes(
"Normalized Main Readout Line Times", lab.
fileName(), lab);
80 for (
int i = 0; i < normalizedReadoutTimes.
Records(); i++) {
82 readoutTimes.push_back((
double) record[
"time"]);
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
virtual void SetDistortion(int naifIkCode)
Load distortion coefficients.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
virtual int CkFrameId() const
CK frame ID.
virtual int CkReferenceId() const
CK Reference ID - J2000.
A single keyword-value pair.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
Parse and return pieces of a time string.
QString m_instrumentNameLong
Full instrument name.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
Convert between undistorted focal plane and ground coordinates.
@ Traverse
Search child objects.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
Convert between parent image coordinates and detector coordinates.
~ClipperNacRollingShutterCamera()
Destructor for a ClipperNacRollingShutterCamera object.
Class for storing Table blobs information.
QString fileName() const
Returns the filename used to initialise the Pvl object.
Distort/undistort focal plane coordinates.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
ClipperNacRollingShutterCamera(Cube &cube)
Constructs a ClipperNacRollingShutterCamera object using the image labels.
double toDouble(const QString &string)
Global function to convert from a string to a double.
void LoadCache()
This loads the spice cache big enough for this image.
bool hasTable(const QString &name)
Check to see if the cube contains a pvl table by the provided name.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
Convert between undistorted focal plane and ra/dec coordinates.
Generic class for Rolling Shutter Cameras.
Clipper EIS Camera model.
int size() const
Returns the number of values stored in this keyword.
virtual int SpkReferenceId() const
SPK Reference ID - J2000.
PvlKeyword & findKeyword(const QString &name)
Find a keyword with a specified name.
int Records() const
Returns the number of records.
QString m_spacecraftNameShort
Shortened spacecraft name.
void SetFocalLength()
Reads the focal length from the instrument kernel.
This is free and unencumbered software released into the public domain.