Loading [MathJax]/jax/output/NativeMML/config.js
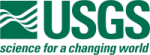 |
Isis 3 Programmer Reference
|
9 #include "CrismCamera.h"
19 #include "CameraFocalPlaneMap.h"
20 #include "LineScanCameraDetectorMap.h"
21 #include "LineScanCameraGroundMap.h"
22 #include "LineScanCameraSkyMap.h"
23 #include "Constants.h"
25 #include "IException.h"
28 #include "NaifStatus.h"
29 #include "SpecialPixel.h"
44 m_isBandDependent(true) {
56 QString sensor = (QString) inst [
"SensorId"];
66 double etStart = getEtTime((QString) inst [
"SpacecraftClockStartCount"]);
67 double etStop = getEtTime((QString) inst [
"SpacecraftClockStopCount"]);
74 int exposure = (int) inst[
"ExposureParameter"];
85 int reg = ((480 - exposure) * 83333) / 480;
89 int start_clocks = reg + (3 * 166);
90 if (start_clocks % 166) start_clocks += 166 - (start_clocks % 166);
105 double frameStartTime(etStart);
114 iTime myLineStartTime(etStart + ((25.0 - 1.0) * frame_time));
117 (void) sce2s_c(
naifSclkCode(), myLineStartTime.
Et(),
sizeof(sclk), sclk);
131 double stime(obsStartTime);
132 double scanTime(stop_time-start_time);
139 double endTime(stime-frame_time+scanTime);
143 int binning = inst[
"PixelAveragingWidth"];
152 double bLine =
getDouble(
"INS"+ikCode+
"_BORESIGHT_LINE");
153 double bSample =
getDouble(
"INS"+ikCode+
"_BORESIGHT_SAMPLE");
194 return (m_isBandDependent);
198 double CrismCamera::getEtTime(
const QString &sclk) {
Convert between undistorted focal plane and ra/dec coordinates.
void SetDetectorOrigin(const double sample, const double line)
Set the detector origin.
void SetBand(const int physicalBand)
Virtual method that sets the band number.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
double SpacecraftAltitude()
Returns the distance from the spacecraft to the subspacecraft point in km.
int ParentLines() const
Returns the number of lines in the parent alphacube.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
Parse and return pieces of a time string.
bool IsBandIndependent()
This is a band-dependant instrument.
QString m_instrumentNameLong
Full instrument name.
virtual iTime getClockTime(QString clockValue, int sclkCode=-1, bool clockTicks=false)
This converts the spacecraft clock ticks value (clockValue) to an iTime.
void SetDetectorSampleSumming(const double summing)
Set sample summing mode.
Container for cube-like labels.
void SetDetectorOffset(const double sampleOffset, const double lineOffset)
Set the detector offset.
virtual void createCache(iTime startTime, iTime endTime, const int size, double tol)
This method creates an internal cache of spacecraft and sun positions over a specified time range.
Convert between parent image coordinates and detector coordinates.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
void SetDetectorLineSumming(const double summing)
Set line summing mode.
@ Traverse
Search child objects.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
Generic class for Line Scan Cameras.
Convert between undistorted focal plane and ground coordinates.
Distort/undistort focal plane coordinates.
IO Handler for Isis Cubes.
QString m_spacecraftNameLong
Full spacecraft name.
SpiceInt naifSclkCode() const
This returns the NAIF SCLK code to use when reading from instrument kernels.
double FocalLength() const
Returns the focal length.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
double PixelPitch() const
Returns the pixel pitch.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
LineScanCameraDetectorMap * DetectorMap()
Returns a pointer to the LineScanCameraDetectorMap object.
QString m_spacecraftNameShort
Shortened spacecraft name.
void SetFocalLength()
Reads the focal length from the instrument kernel.
This is free and unencumbered software released into the public domain.
Convert between parent image coordinates and detector coordinates.
Container class for storing timing information for a section of an image.